[nodemailer] Render component when sending email
Unanswered
Savannah posted this in #help-forum
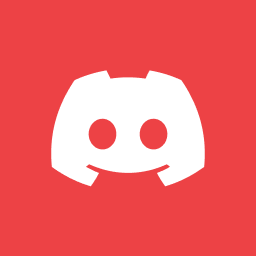
SavannahOP
Hi guys, i would like to know if it's possible to render a component when an email is send ?
import nodemailer from 'nodemailer';
import Mail from 'nodemailer/lib/mailer';
import SMTPTransport from "nodemailer/lib/smtp-transport";
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: process.env.SERVER_MAIL_USER,
pass: process.env.SERVER_MAIL_PASS
}
} as SMTPTransport.Options);
type SendEmailOptions = {
sender: Mail.Address,
recipients: Mail.Address[],
subject: string,
message: string
}
export const sendMail = async ({sender, recipients, subject, message}: SendEmailOptions) => {
console.log('sender', sender, 'recipients', recipients)
try {
await transporter.sendMail({
from: '"Maddison Foo Koch :ghost:" <maddison53@ethereal.email>',
to: "test.fafa@gmail.com",
subject: "Hello ✔",
text: "Hello world?",
html: "<b>Hello world?</b>"
});
console.log('Message sent successfully')
} catch (error) {
console.error('error sending message', error)
}
}
38 Replies
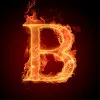
B33fb0n3
yes you can. You can return a specific response and if the response is successful, you can do stuff with the client. Either redirect him or conditionally render the component or ...
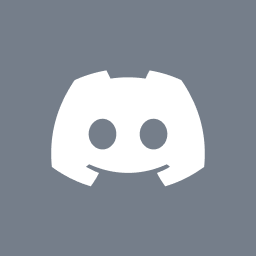
Gharial
bro i will suggest you to use React Email if you want to render components in email
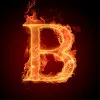
B33fb0n3
it seems like he want to render a component after the mail is sent.
... to render a component when an email ...Not the component inside the email.
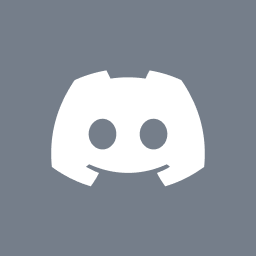
Gharial
in this case just use the response if mail sent successfully then show the success and not then error simple
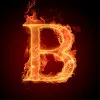
B33fb0n3
that's what I said: https://nextjs-forum.com/post/1244633427265978471#message-1244649092114808912
Do you read his or my messages in detail? For me it seem's like you don't 🤔
Do you read his or my messages in detail? For me it seem's like you don't 🤔
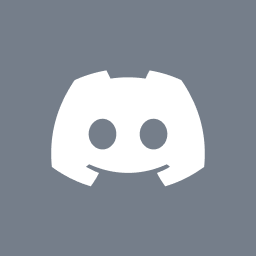
Gharial
sorry i forgot to read this message
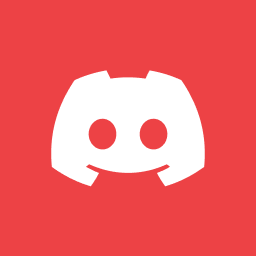
SavannahOP
Hey, thanks for the answer. But i'd like to render the component instead of
<b>Hello world?</b>
in the html variableI think that's what i'm looking for, thanks i'll check that asap 😉
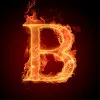
B33fb0n3
ah ok, yea. Then I read your message wrong
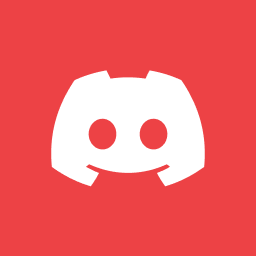
SavannahOP
Don't worry, I may have misspoken as well. x)
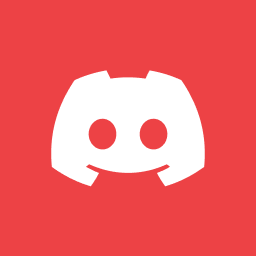
SavannahOP
It's working, thanks @B33fb0n3 and @Gharial ! Have a good day 🙂
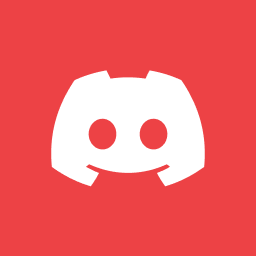
SavannahOP
May i ask you a question @Gharial please ?
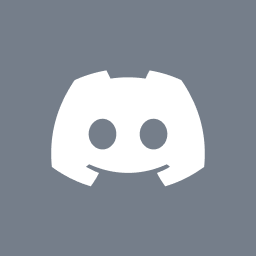
Gharial
Sure ask
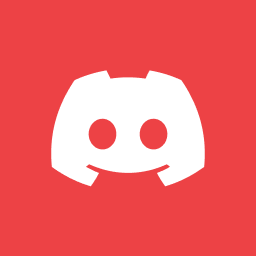
SavannahOP
Did you know why my className are not in the dom when i send an email ?
import {Button, Html} from "@react-email/components";
import "@/styles/emails/VerificationCodeTemplate.scss";
export function VerificationCodeTemplate() {
return (
<Html>
<div className="wrapper-template">
<div className="wrapper-header">
<Button href="https://www.google.com">Visiter le site</Button>
</div>
<div className="wrapper-texts">
<h1>Terminer l'inscription</h1>
<h2>Entrez le code de vérification suivant pour terminer votre inscription</h2>
</div>
<div className="wrapper-code">
<span>450994</span>
</div>
<p>Si vous n'êtes pas à l'origine de cette demande, veuillez ignorer ce message</p>
</div>
</Html>
)
}

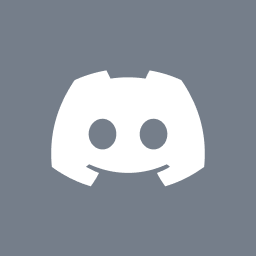
Gharial
Can I know what is your file name
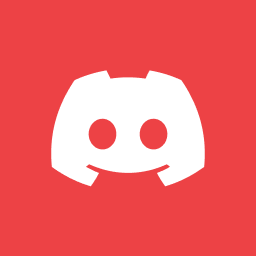
SavannahOP
Which one ? For the style or the component ?
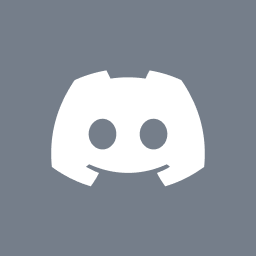
Gharial
With extension use .jsx and tsx if typescript
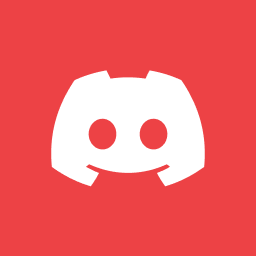
SavannahOP

my extensions is tsx
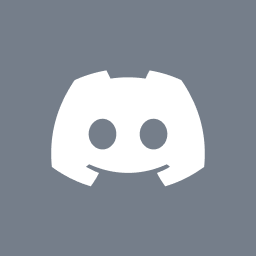
Gharial
How you are rendering on nodemailer
Can I see?
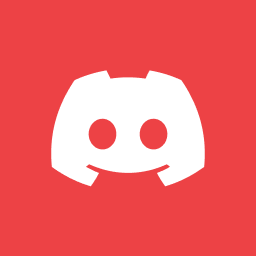
SavannahOP
Like this
import nodemailer from 'nodemailer';
import Mail from 'nodemailer/lib/mailer';
import SMTPTransport from "nodemailer/lib/smtp-transport";
import {render} from "@react-email/render";
import {VerificationCodeTemplate} from "@/app/components/emails/VerificationCodeTemplate";
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: process.env.SERVER_MAIL_USER,
pass: process.env.SERVER_MAIL_PASS
}
} as SMTPTransport.Options);
type SendEmailOptions = {
sender: Mail.Address,
recipients: Mail.Address[],
subject: string,
message: string
}
export const sendMail = async ({sender, recipients, subject, message}: SendEmailOptions) => {
try {
await transporter.sendMail({
from: `${sender.name} <${sender.address}>`,
to: recipients.map(recipient => `${recipient.name} <${recipient.address}>`).join(', '),
subject: subject,
html: render(VerificationCodeTemplate())
});
console.log('Message sent successfully')
} catch (error) {
console.error('error sending message', error)
}
}
I got the email

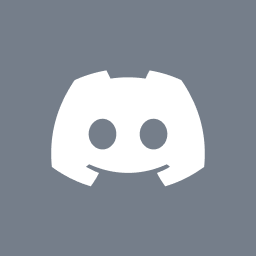
Gharial
Sorry this will not support classnam
Use on documentations for this
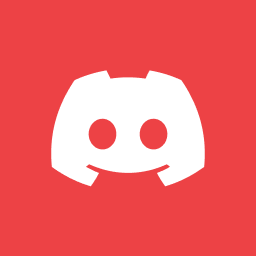
SavannahOP
Ohh, okay. And how can i custom it then ?
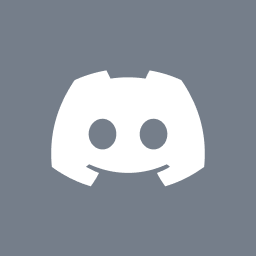
Gharial
Use style tag or jsx style
You can use tailwind also but you need to check the docs
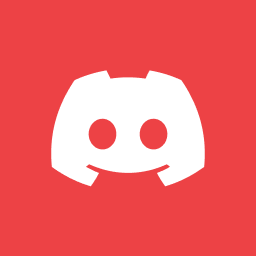
SavannahOP
yeah, i see
thanks brother
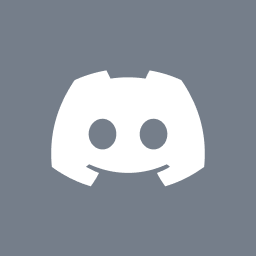
Gharial
I am not sure but className work I need to check
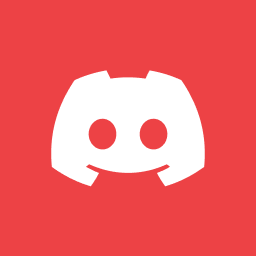
SavannahOP
if you can find it, i'll take it 😉
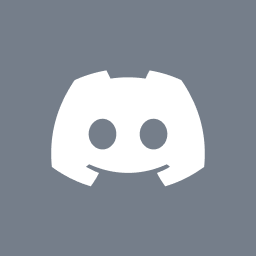
Gharial
bro you use tailwind like this - https://react.email/docs/components/tailwind
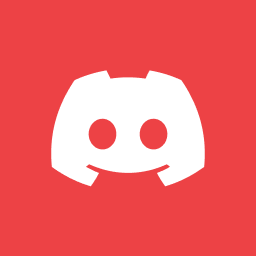
SavannahOP
Yeah, i see it on the doc. But i'm not using Tailwind 😉
But i use the style tag
and it's working great
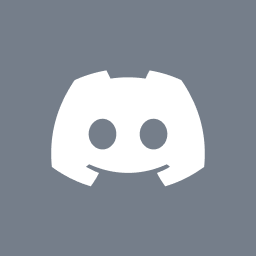
Gharial
okay