ModalProvider breaks the layout
Answered
Birman posted this in #help-forum
BirmanOP
Hi everyone, I'm a bit of a noob who is trying to write a web application based on tutorials. I added a navigation on the left, and I wanted to add a CreateServerModal modal that will be opened by clicking on the “+” button from the same navigation on the left. But here's the problem, when I add <ModalProvider /> inside RootLayout, I get an error like on the second screen and the navigation on the left is not displayed. What can be the problem?
here's ModalProvider code:
"use client";
import { CreateServerModal } from "@/components/modals/create-server-modal";
import { useEffect, useState } from "react";
export const ModalProvider = () => {
const [isMounted, setIsMounted] = useState(false);
useEffect(() => {
setIsMounted(true);
}, []);
if (!isMounted) {
return null;
}
return (
#Unknown Channel
<CreateServerModal />
</>
)
};
here's ModalProvider code:
"use client";
import { CreateServerModal } from "@/components/modals/create-server-modal";
import { useEffect, useState } from "react";
export const ModalProvider = () => {
const [isMounted, setIsMounted] = useState(false);
useEffect(() => {
setIsMounted(true);
}, []);
if (!isMounted) {
return null;
}
return (
#Unknown Channel
<CreateServerModal />
</>
)
};
Answered by Birman
I FOUND THE REAL SOLUTION
If you are having an issue with disappearing navbar & sidebar upon leaving the server, deleting the server etc.
You can fix this by moving UploadThing's styles into global.css at the end of the file instead of in the react component.
Step 1:
- Remove the import for upload-thing styles inside file-upload.tsx component
- DELETE => import "@uploadthing/react/styles.css";
Step 2:
- Add the import at the bottom of the globals.css file instead
// globals.css
...
@import "~@uploadthing/react/styles.css";
Step 3 (optional):
- Wrap the tailwind config with "withUt":
// tailwind.config.js
const { withUt } = require("uploadthing/tw");
module.exports = withUt({
...leave everything the same
});
If you are having an issue with disappearing navbar & sidebar upon leaving the server, deleting the server etc.
You can fix this by moving UploadThing's styles into global.css at the end of the file instead of in the react component.
Step 1:
- Remove the import for upload-thing styles inside file-upload.tsx component
- DELETE => import "@uploadthing/react/styles.css";
Step 2:
- Add the import at the bottom of the globals.css file instead
// globals.css
...
@import "~@uploadthing/react/styles.css";
Step 3 (optional):
- Wrap the tailwind config with "withUt":
// tailwind.config.js
const { withUt } = require("uploadthing/tw");
module.exports = withUt({
...leave everything the same
});
102 Replies
@Birman Hi everyone, I'm a bit of a noob who is trying to write a web application based on tutorials. I added a navigation on the left, and I wanted to add a CreateServerModal modal that will be opened by clicking on the “+” button from the same navigation on the left. But here's the problem, when I add <ModalProvider /> inside RootLayout, I get an error like on the second screen and the navigation on the left is not displayed. What can be the problem?
here's ModalProvider code:
"use client";
import { CreateServerModal } from "@/components/modals/create-server-modal";
import { useEffect, useState } from "react";
export const ModalProvider = () => {
const [isMounted, setIsMounted] = useState(false);
useEffect(() => {
setIsMounted(true);
}, []);
if (!isMounted) {
return null;
}
return (
<>
<CreateServerModal />
</>
)
};
Hi, I'd appreciate it if you could show me more code for your ModalProvider-related components.
- Code
- How to import
- Parent component
I think the error is partly how you've defined the exports in your modules. I'd like to verify this.
- Code
- How to import
- Parent component
I think the error is partly how you've defined the exports in your modules. I'd like to verify this.
Abyssinian
hey
@Losti! Hi, I'd appreciate it if you could show me more code for your ModalProvider-related components.
- Code
- How to import
- Parent component
I think the error is partly how you've defined the exports in your modules. I'd like to verify this.
BirmanOP
Hello!
use-modal-store.ts file from hooks folder:
modal-provider.tsx
use-modal-store.ts file from hooks folder:
import { create } from 'zustand';
export type ModalType = "createServer";
interface ModalStore {
type: ModalType | null;
isOpen: boolean;
onOpen: (type: ModalType) => void;
onClose: () => void;
}
export const useModal = create<ModalStore>((set) => ({
type: null,
isOpen: false,
onOpen: (type) => set({ isOpen: true, type }),
onClose: () => set({ type: null, isOpen: false })
}));
modal-provider.tsx
"use client";
import { CreateServerModal } from "@/components/modals/create-server-modal";
import { useEffect, useState } from "react";
export const ModalProvider = () => {
const [isMounted, setIsMounted] = useState(false);
useEffect(() => {
setIsMounted(true);
}, []);
if (!isMounted) {
return null;
}
return (
<>
<CreateServerModal />
</>
)
};
@Losti! Hi, I'd appreciate it if you could show me more code for your ModalProvider-related components.
- Code
- How to import
- Parent component
I think the error is partly how you've defined the exports in your modules. I'd like to verify this.
Abyssinian
can you help me with a few things? sorry for replying in this ticket. i'm very new to nextjs
That's simple, you have to read:
https://nextjs.org/docs/app/building-your-application/routing/route-groups
But in summary, within the path group, any file that is page.tsx the path segment "/" as long as it is not inside a directory.
https://nextjs.org/docs/app/building-your-application/routing/route-groups
But in summary, within the path group, any file that is page.tsx the path segment "/" as long as it is not inside a directory.
Sorry, I see it now saya.
BirmanOP
root layout.tsx
import "./globals.css";
import type { Metadata } from "next";
import { Open_Sans } from "next/font/google";
import { ClerkProvider } from '@clerk/nextjs';
import { enUS } from '@clerk/localizations';
import { cn } from "@/lib/utils";
import { ThemeProvider } from "@/components/providers/theme-provider";
import { ModalProvider } from "@/components/providers/modal-provider";
const font = Open_Sans({
subsets: ['latin']
});
export const metadata: Metadata = {
title: "Khabar App",
description: "Generated by create next app",
};
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<ClerkProvider localization={localization} >
<html lang="en" suppressHydrationWarning>
<body className={cn(
font.className,
"bg-white dark:bg-[#0F172B]"
)}>
<ThemeProvider
attribute="class"
defaultTheme="dark"
enableSystem={false}
storageKey="khabar-theme"
>
<ModalProvider />
{children}
</ThemeProvider>
</body>
</html>
</ClerkProvider>
);
}
navigation-action.tsx
"use client";
import { Plus } from "lucide-react";
import { ActionTooltip } from "@/components/action-tooltip";
import { useModal } from "@/hooks/use-modal-store";
export const NavigationAction = () => {
const { onOpen } = useModal();
return (
<div>
<ActionTooltip side="right" align="center" label="New server">
<button
onClick = {() => onOpen("createServer")}
className="group flex items-center">
<div className="flex mx-3 h-[48px] w-[48px] rounded-[24px] group-hover:rounded-[16px] transition-all overflow-hidden items-center justify-center bg-slate-50 dark:bg-slate-700 group-hover:bg-orange-500">
<Plus
className="group-hover:text-white transition text-orange-500"
size={25}
/>
</div>
</button>
</ActionTooltip>
</div>
)
}
@Losti! Sorry, I see it now saya.
BirmanOP
I can translate create-server-modal.tsx for you that I already sent if you need 🙂
I was just following this tutorial. timecode is 2:50:00
https://www.youtube.com/watch?v=ZbX4Ok9YX94
https://www.youtube.com/watch?v=ZbX4Ok9YX94
Abyssinian
if i place not-found.tsx in (error)
@Birman I can translate create-server-modal.tsx for you that I already sent if you need 🙂
I'm looking at it, and it seems to me that we both missed a small detail.
I assumed your root layout was well-defined, but you have a variable that doesn't exist, I don't think. You use it in your root layout:
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<ClerkProvider localization={localization /* this variable */}>
<html lang="en" suppressHydrationWarning>
<body className={cn(font.className, "bg-white dark:bg-[#0F172B]")}>
<ThemeProvider
attribute="class"
defaultTheme="dark"
enableSystem={false}
storageKey="khabar-theme"
>
<ModalProvider />
{children}
</ThemeProvider>
</body>
</html>
</ClerkProvider>
);
}
But in your imports this does not exist.
import "./globals.css";
import type { Metadata } from "next";
import { Open_Sans } from "next/font/google";
import { ClerkProvider } from "@clerk/nextjs";
import { enUS } from "@clerk/localizations";
import { cn } from "@/lib/utils";
import { ThemeProvider } from "@/components/providers/theme-provider";
import { ModalProvider } from "@/components/providers/modal-provider";
At no time do you import location
BirmanOP
you mean this part?
const localization = {
...enUS,
signUp: {
start: {
title: 'Жаңа аккаунт',
subtitle: '{{applicationName}} жалғастыру үшін тіркелу керек',
actionText: 'Аккаунт бар ма?',
actionLink: 'Кіру',
},
emailLink: {
title: 'Поштаңызды растаңыз',
subtitle: '{{applicationName}} жалғастыру үшін',
resendButton: 'Хат алмадыңыз ба? Қайта жіберу',
},
},
dividerText: 'немесе',
formFieldLabelusername: 'Пайдаланушы аты',
formFieldLabelemailAddress: 'Пошта',
formFieldInputPlaceholderemailAddress: 'Поштаңызды енгізіңіз',
formFieldLabelpassword: 'Құпия сөз',
formFieldInputPlaceholderpassword: 'Құпия сөзді енгізіңіз',
formButtonPrimary: 'Жалғастыру',
formFieldLabelemailAddress_username: 'Пошта немесе пайдаланушы аты',
formFieldInputPlaceholder__emailAddress_username: 'Пошта немесе пайдаланушы атын енгізіңіз',
socialButtonsBlockButton: '{{provider|titleize}} арқылы кіру',
form_param_format_invalid: 'Енгізілген сөз қате форматта. Тексеріп, түзетіңіз.',
signIn: {
start: {
actionLink: 'Тіркелу',
actionText: 'Аккаунт жоқ па?',
subtitle: 'Қош келдіңіз! Жалғастыру үшін аккаунтыңызға кіріңіз',
subtitleCombined: undefined,
title: '{{applicationName}} кіру',
},
}
};
const localization = {
...enUS,
signUp: {
start: {
title: 'Жаңа аккаунт',
subtitle: '{{applicationName}} жалғастыру үшін тіркелу керек',
actionText: 'Аккаунт бар ма?',
actionLink: 'Кіру',
},
emailLink: {
title: 'Поштаңызды растаңыз',
subtitle: '{{applicationName}} жалғастыру үшін',
resendButton: 'Хат алмадыңыз ба? Қайта жіберу',
},
},
dividerText: 'немесе',
formFieldLabelusername: 'Пайдаланушы аты',
formFieldLabelemailAddress: 'Пошта',
formFieldInputPlaceholderemailAddress: 'Поштаңызды енгізіңіз',
formFieldLabelpassword: 'Құпия сөз',
formFieldInputPlaceholderpassword: 'Құпия сөзді енгізіңіз',
formButtonPrimary: 'Жалғастыру',
formFieldLabelemailAddress_username: 'Пошта немесе пайдаланушы аты',
formFieldInputPlaceholder__emailAddress_username: 'Пошта немесе пайдаланушы атын енгізіңіз',
socialButtonsBlockButton: '{{provider|titleize}} арқылы кіру',
form_param_format_invalid: 'Енгізілген сөз қате форматта. Тексеріп, түзетіңіз.',
signIn: {
start: {
actionLink: 'Тіркелу',
actionText: 'Аккаунт жоқ па?',
subtitle: 'Қош келдіңіз! Жалғастыру үшін аккаунтыңызға кіріңіз',
subtitleCombined: undefined,
title: '{{applicationName}} кіру',
},
}
};
it's just discord won't let me send long messages 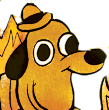
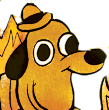
Try this.
import { enUS as localization } from "@clerk/localizations";
BirmanOP
it shows this error
@Birman it shows this error
oh my bad
I'm looking at it right now, so it has nothing to do with the provider.
@Losti! Can you see if what clerkprovider expects is the full localization variable? <:this_is_fine:770004314634321971>
BirmanOP
you think navigation isn't displaying because of clerk?
@Birman you think navigation isn't displaying because of clerk?
Clerk is working as expected, right? It provides language-based routing and all that...? If so, it has nothing to do with the problem you're having.
Abyssinian
whats the problem
i'm new but i can help
frfr
@Abyssinian i'm new but i can help
BirmanOP
well when i add <ModalProvider /> inside my root layout.tsx, navigation on the left side disappeares
but when i remove <ModalProvider /> navigation appears again
Abyssinian
girl 😭 🙏
i come from react
i'm very new to this
but
i'd say
shadcn dialogs
@Abyssinian girl 😭 🙏
BirmanOP
oh thank u anyway 😭
always did the modals in my apps that way too
but it way always werid and messy
@Abyssinian but it way always werid and messy
BirmanOP
thx!
@Birman Click to see attachment
It's strange behavior.
@Abyssinian shadcn dialogs
They are very helpful
@Losti! It's strange behavior.
BirmanOP
yes, i did everything like in the video tutorial
idk what's wrong...
I don't understand. If they're components made from scratch, there must be something there that's causing a conflict with the content. I assumed you were using shadcn.
BirmanOP
i am using shdcn fot ui
here in create-server-modal.tsx
So this problem shouldn't be happening, since the dialog is set to false, the content isn't displayed at all.
By default you have it set to false, it should not affect the interface.
BirmanOP
yess
@Birman yess
Can you check the dev tools and see if your sidebar has moved in a strange way?
They make it easy for you to see that kind of behavior.
chatgpt said i can ignore these warnings idk
What we need to see is if the sidebar is rendered in the HTML and where it is.
If the position of the sidebar is outside the window we will know it and we will also be able to see the possible causes due to the HTML
Abyssinian
ello
yal got progress?
@Abyssinian yal got progress?
BirmanOP
nah
Abyssinian
oo
rip
i'm not a help):
@Losti! What we need to see is if the sidebar is rendered in the HTML and where it is.
BirmanOP
where should i look for it? 😭
BirmanOP
here's main layout.tsx
import { NavigationSidebar } from "@/components/navigation/navigation-sidebar";
const MainLayout = async ({
children
}: {
children: React.ReactNode;
}) => {
return (
<div className="h-full">
<div className="hidden md:flex h-full w-[72px] z-30 flex-col fixed inset-y-0">
<NavigationSidebar />
</div>
<main className="md:pl-[72px] h-full">{children}</main>
</div>
);
}
export default MainLayout;
import { NavigationSidebar } from "@/components/navigation/navigation-sidebar";
const MainLayout = async ({
children
}: {
children: React.ReactNode;
}) => {
return (
<div className="h-full">
<div className="hidden md:flex h-full w-[72px] z-30 flex-col fixed inset-y-0">
<NavigationSidebar />
</div>
<main className="md:pl-[72px] h-full">{children}</main>
</div>
);
}
export default MainLayout;
@Birman where should i look for it? 😭
In Elements, find your sidebar's container. Selecting the HTML tag should show you where it's located on the screen. If you're using Flex or Grid, you can highlight it with a small button (click it and it will add a border to the container box) next to the open HTML tag.
This will highlight the element.
This will highlight the element.
I just want to know if the sidebar is displayed correctly and where it is when the modal provider exists within the layout. Maybe it's just a styling issue...
look when i remove the check i can see sidebar
should i remove it?
@Birman should i remove it?
As long as the viewport size is smaller than md (764px if I'm not mistaken) it will be with display none, it shouldn't affect... even so let's try removing it
try removing inset-y-0 as well
@Losti! As long as the viewport size is smaller than md (764px if I'm not mistaken) it will be with display none, it shouldn't affect... even so let's try removing it
BirmanOP
yea now sidebar appeared and modal works when i press "+" button. do you think it won't affect my code in the future if i remove hidden?
@Losti! try removing inset-y-0 as well
BirmanOP
okay
@Birman yea now sidebar appeared and modal works when i press "+" button. do you think it won't affect my code in the future if i remove hidden?
The idea behind hiding it is to make it scrollable with an animation on the X axis when the screen size is very small. If this is the only problem you're having with your code right now, it's a good idea to use some help.
https://ui.shadcn.com/blocks#sidebar-07
https://ui.shadcn.com/blocks#sidebar-07
Perhaps try adding this sidebar and modifying it to your liking.
@Losti! The idea behind hiding it is to make it scrollable with an animation on the X axis when the screen size is very small. If this is the only problem you're having with your code right now, it's a good idea to use some help.
https://ui.shadcn.com/blocks#sidebar-07
BirmanOP
okay! thank you very much! you really helped me, i think now i am gonna read all the docs
w yal
shadcn on top
This is what I did in my case; it helped a lot.
I know this doesn't directly solve your problem (and it still seems like a styling issue to me), but I hope it can help relieve this stress you're probably carrying.
I know this doesn't directly solve your problem (and it still seems like a styling issue to me), but I hope it can help relieve this stress you're probably carrying.
I'm glad, go ahead, I hope things go well for you saya.
@Losti! I'm glad, go ahead, I hope things go well for you saya.
BirmanOP
thank you! good luck to you too! 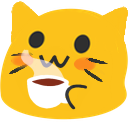
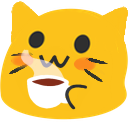
BirmanOP
I FOUND THE REAL SOLUTION
If you are having an issue with disappearing navbar & sidebar upon leaving the server, deleting the server etc.
You can fix this by moving UploadThing's styles into global.css at the end of the file instead of in the react component.
Step 1:
- Remove the import for upload-thing styles inside file-upload.tsx component
- DELETE => import "@uploadthing/react/styles.css";
Step 2:
- Add the import at the bottom of the globals.css file instead
// globals.css
...
@import "~@uploadthing/react/styles.css";
Step 3 (optional):
- Wrap the tailwind config with "withUt":
// tailwind.config.js
const { withUt } = require("uploadthing/tw");
module.exports = withUt({
...leave everything the same
});
If you are having an issue with disappearing navbar & sidebar upon leaving the server, deleting the server etc.
You can fix this by moving UploadThing's styles into global.css at the end of the file instead of in the react component.
Step 1:
- Remove the import for upload-thing styles inside file-upload.tsx component
- DELETE => import "@uploadthing/react/styles.css";
Step 2:
- Add the import at the bottom of the globals.css file instead
// globals.css
...
@import "~@uploadthing/react/styles.css";
Step 3 (optional):
- Wrap the tailwind config with "withUt":
// tailwind.config.js
const { withUt } = require("uploadthing/tw");
module.exports = withUt({
...leave everything the same
});
Answer
@Losti! Glad you found it, congratulations! :)
BirmanOP
thx!