Where to place my API fetching methods before my NextJS app mounts/renders
Unanswered
Asiatic Lion posted this in #help-forum
Asiatic LionOP
- Using: NextJS 14, Typescript, Zustand
- App section: front end (not backend used)
- Nextjs Experience: less than a year (new
)
- Coming from: vue.js
- Description:
* I want to fetch data from an API before the landing page mounts, so that I can populate the different components that need the data. I have zustand stores with a method that does the fetching but I'm having a hard time grasping the concept of where should I call this methods for data fetching. I understand I can use "useEffect" on the page (page.tsx). But is there any other place that will execute the fetching and have data ready for components to use ?
- App section: front end (not backend used)
- Nextjs Experience: less than a year (new
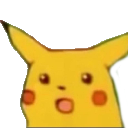
- Coming from: vue.js
- Description:
* I want to fetch data from an API before the landing page mounts, so that I can populate the different components that need the data. I have zustand stores with a method that does the fetching but I'm having a hard time grasping the concept of where should I call this methods for data fetching. I understand I can use "useEffect" on the page (page.tsx). But is there any other place that will execute the fetching and have data ready for components to use ?
11 Replies
Asiatic LionOP
As an example this is my landing "page.tsx":
'use client'
imports ...
const metadata: Metadata = {
title: "LANDING PAGE",
}
export default function Page() {
const {products, fetchProducts} = useProducts();
const {fetchEvents} = useEvents();
useEffect( () => {
async function fetchProductsABWSAPI() {
try{
await Promise.all([fetchProducts(), fetchEvents()]);
}
catch(error){
console.error('Error fetching initial data:', error);
}
}
fetchProductsABWSAPI();
}, [fetchProducts,fetchEvents]);
if(!products.length){
return <div> Loading ... </div>;
}
return (
<main>
<div className="flex justify-center bg-[#FEF6F0]">
<h1 className=" my-5 text-3xl text-[#8A7562] font-bold underline">Welcome to</h1>
</div>
<div >
<Carousel images={getHomeImages()}/>
</div>
<div className="ml-[5%] mr-[5%] mb-[2%] border-solid border-green-500 border-2">
<div className={creepster.className}>
<UIButton name="Seasonal Menu" path="/products/season"/>
</div>
<BestSellerBox />
<div>
<InfoArea info={InfoPanels} />
</div>
</div>
</main>
);
}
@Asiatic Lion As an example this is my landing "page.tsx":
typescript
'use client'
imports ...
const metadata: Metadata = {
title: "LANDING PAGE",
}
export default function Page() {
const {products, fetchProducts} = useProducts();
const {fetchEvents} = useEvents();
useEffect( () => {
async function fetchProductsABWSAPI() {
try{
await Promise.all([fetchProducts(), fetchEvents()]);
}
catch(error){
console.error('Error fetching initial data:', error);
}
}
fetchProductsABWSAPI();
}, [fetchProducts,fetchEvents]);
if(!products.length){
return <div> Loading ... </div>;
}
return (
<main>
<div className="flex justify-center bg-[#FEF6F0]">
<h1 className=" my-5 text-3xl text-[#8A7562] font-bold underline">Welcome to</h1>
</div>
<div >
<Carousel images={getHomeImages()}/>
</div>
<div className="ml-[5%] mr-[5%] mb-[2%] border-solid border-green-500 border-2">
<div className={creepster.className}>
<UIButton name="Seasonal Menu" path="/products/season"/>
</div>
<BestSellerBox />
<div>
<InfoArea info={InfoPanels} />
</div>
</div>
</main>
);
}
First of all this will fail since you can’t export
Also if you want to make sure data is fetched before you start rendering the actual HTML output then just use a Server Component.
To do this:
1. remove “use client” from the top
2. This will make your page component a Server Component (default) and you can mark it as async.
3. Now you can fetch the data directly inside the component and you’ll await for the fetching to be done before you render the UI that depends on that data.
Result: data fetching will be executed, so your data will be ready for your component to use it.
You can follow the same principles to fetch data in your Layout.tsx file and forward that data into a Context provider or use it to render UI
metadata
from a page file marked with “use client”.Also if you want to make sure data is fetched before you start rendering the actual HTML output then just use a Server Component.
To do this:
1. remove “use client” from the top
2. This will make your page component a Server Component (default) and you can mark it as async.
3. Now you can fetch the data directly inside the component and you’ll await for the fetching to be done before you render the UI that depends on that data.
Result: data fetching will be executed, so your data will be ready for your component to use it.
You can follow the same principles to fetch data in your Layout.tsx file and forward that data into a Context provider or use it to render UI
For the loading state, at first you’re gonna loose it since now you’re using server components, to have it back add a loading.tsx next to your page.tsx file. And here put the fallback that will be displayed while your server component is fetching data. This is effectively a <Suspense> boundary under the hood (not sure if you’re familiar with it, it’s a react primitive)
If you try let me know, did I help with the main issue you were having?
Asiatic LionOP
Thank you for you help @LuisLl , and yes will do.
I have not applied the changes (tired towards the end of the day) but tomorrow I will.
I have not applied the changes (tired towards the end of the day) but tomorrow I will.
@Asiatic Lion any updates?
@Asiatic Lion solved?
Clumber Spaniel
@LuisLl sorry for hijacking this thread, but what if the data here could mutate while on the page? would then be better to setup something like tanstack query to handle these mutations to sync them? the fetching would then be completely done on client though
@Clumber Spaniel <@744377057139753020> sorry for hijacking this thread, but what if the data here could mutate while on the page? would then be better to setup something like tanstack query to handle these mutations to sync them? the fetching would then be completely done on client though
That would completely depend on the needs of your app, if you really need up-to-date data and have it always synchronized then React Query is the best solution.
Server Components tend to get outdated and stale since you need to trigger revalidation and wait until the next request to get the fresh data + ui back.
Server Components tend to get outdated and stale since you need to trigger revalidation and wait until the next request to get the fresh data + ui back.
What the OP wanted to do, if I remember correctly, he wanted to make sure data was fetched before the UI started rendering, which is a nice use case for server components.
Also, have in mind that Server Actions in next.js also refresh the current page you’re on, in the same round rip to the server since they return the fresh data + updated UI, but the other pages that depend on that revalidated data will need to be requested again in order to show fresh data and UI