Fonts with Tailwind 4
Unanswered
False honey ant posted this in #help-forum
False honey antOP
What is the proper way to be importing fonts? All the tutorials I've seen have been for Tailwind 3 and since everything is supposed to be inside global.css now with the removal of tailwind.config.js. I can't seem to figure out how to use the next font package.
66 Replies
@Losti! https://tailwindcss.com/docs/font-family
False honey antOP
I've read the docs
... Let me show you how I do it.
import { Playfair_Display, Montserrat } from "next/font/google";
export const playfair_display = Playfair_Display({
variable: "--font-playfair-display",
subsets: ["latin"],
weight: ["400", "600", "800"],
style: ["normal", "italic"],
display: "swap",
adjustFontFallback: true,
preload: true,
});
export const montserrat = Montserrat({
variable: "--font-montserrat",
subsets: ["latin"],
weight: ["200", "300", "400", "500", "600"],
style: ["normal", "italic"],
display: "swap",
adjustFontFallback: true,
preload: true,
});
import { playfair_display, montserrat } from "./fonts";
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<html
suppressHydrationWarning
suppressContentEditableWarning
>
{env.NODE_ENV == "development" && (
<script
async
src="//unpkg.com/react-scan/dist/auto.global.js"
></script>
)}
<body
className={`${playfair_display.variable} ${montserrat.variable} antialiased`}
>
{children}
</body>
</html>
);
}
False honey antOP
Okay so there's basically no way to use tailwind for nextjs fonts anymore?
@import "tailwindcss";
@theme {
/* Fonts */
--font-playfair-display: var(--font-playfair-display);
--font-montserrat: var(--font-montserrat);
}
@False honey ant Okay so there's basically no way to use tailwind for nextjs fonts anymore?
If you can, you have to use CSS to import the fonts.
and
As you can see in the example of my global.css define them in theme as variables with the prefix font-<name-font-here>
that's all
False honey antOP
I did exactly this last night and was not getting the same results let me double check to make sure I didn't mess it up anywhere
Okey
False honey antOP
import "@/styles/globals.css";
import { Figtree } from "next/font/google";
import type { AppProps } from "next/app";
export const figtree = Figtree({
variable: "--font-figtree",
subsets: ["latin"],
weight: ["500"],
preload: true,
});
export default function App({ Component, pageProps }: AppProps) {
return (
<main className={`${figtree.variable}`}>
<Component {...pageProps} />;
</main>
)
}
@theme {
--font-figtree: var(--font-figtree);
}
Am I doing something wrong here?
No, it's okay
False honey antOP
It's still not working 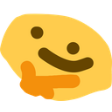
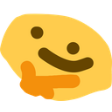
Is the result as expected or is there some strange behavior?
@False honey ant It's still not working <:thinkies:591480689057071132>
check the devtools (network side)
Check if the font is downloading.
False honey antOP
Alright one second
Doesn't look like the font is downloading
wait no it might be i actually can't tell the woff2 it's downloading has some arbitrary name
@False honey ant Doesn't look like the font is downloading
Okay, try other fonts (you don't have to use a specific one). We'll see if the same behavior persists with others.
@False honey ant wait no it might be i actually can't tell the woff2 it's downloading has some arbitrary name
That should be handled by next.js by default, as far as I know.
False honey antOP
import "@/styles/globals.css";
import { Figtree } from "next/font/google";
import type { AppProps } from "next/app";
import { Playfair_Display } from "next/font/google";
export const playfair_display = Playfair_Display({
variable: "--font-playfair-display",
subsets: ["latin"],
weight: ["400", "600", "800"],
style: ["normal", "italic"],
display: "swap",
adjustFontFallback: true,
preload: true,
});
export const figtree = Figtree({
variable: "--font-figtree",
subsets: ["latin"],
weight: ["500"],
preload: true,
});
export default function App({ Component, pageProps }: AppProps) {
return (
<main className={`${playfair_display.variable}`}>
<Component {...pageProps} />;
</main>
)
}
Same issue
This is all that's needed inside the global.css file right?
@theme {
--font-figtree: var(--font-figtree);
--font-playfair-display: var(--font-playfair-display);
}
Can you show me your package.json? (only the vital stuff please)
False honey antOP
AH
False honey antOP
Oh no lmao
I wish that was the case though
You had me sweating for a second there
"dependencies": {
"react": "^19.0.0",
"react-dom": "^19.0.0",
"next": "15.2.2"
},
"devDependencies": {
"typescript": "^5",
"@types/node": "^20",
"@types/react": "^19",
"@types/react-dom": "^19",
"@tailwindcss/postcss": "^4",
"tailwindcss": "^4",
"eslint": "^9",
"eslint-config-next": "15.2.2",
"@eslint/eslintrc": "^3"
}
@False honey ant json
"dependencies": {
"react": "^19.0.0",
"react-dom": "^19.0.0",
"next": "15.2.2"
},
"devDependencies": {
"typescript": "^5",
"@types/node": "^20",
"@types/react": "^19",
"@types/react-dom": "^19",
"@tailwindcss/postcss": "^4",
"tailwindcss": "^4",
"eslint": "^9",
"eslint-config-next": "15.2.2",
"@eslint/eslintrc": "^3"
}
Looks good, have you migrated to the tailwind client?
Just to make sure it's not a tailwind migration error.
$ npx @tailwindcss/upgrade
Just to make sure it's not a tailwind migration error.
(I've had problems with the migration client)
False honey antOP
This is my first time messing with next so idk for sure but is this importing the variable as intended?
False honey antOP
@tailwindcss/upgrade@4.0.13
Ok to proceed? (y) y
≈ tailwindcss v4.0.13
│ Git directory is not clean. Please stash or commit your changes before migrating.
│ You may use the `--force` flag to silence this warning and perform the migration.
I should continue with this then?
It seems to work except it doesn't show the font family. This is because the variable is specified, but you haven't used it, right? You can put it inside your elements. In case you've already tried it and it doesn't work for testing, create a test project by just importing the fonts and seeing if the behavior is different.
@False honey ant
@tailwindcss/upgrade@4.0.13
Ok to proceed? (y) y
≈ tailwindcss v4.0.13
│ Git directory is not clean. Please stash or commit your changes before migrating.
│ You may use the `--force` flag to silence this warning and perform the migration.
I should continue with this then?
If you already have Tailwind 4, there is no need to upgrade further, it's a strange error.
v4 is now the stable version so I would say stay there
(Unless you see something that prompts you to move to the latest version.)
False honey antOP
Alright
I'll try and make a new project see if I have same error
I assume usig Turbopack and not having a AppRouter shouldn't be the cause to this issue right?
@False honey ant I assume usig Turbopack and not having a AppRouter shouldn't be the cause to this issue right?
No problem at all unless you have a specific configuration on your part (own experience).
False honey antOP
Okay so seems like it's functioning the same. I kept everything as default:
import "@/styles/globals.css";
import type { AppProps } from "next/app";
import { Playfair_Display } from "next/font/google";
export const playfair_display = Playfair_Display({
variable: "--font-playfair-display",
subsets: ["latin"],
weight: ["400", "600", "800"],
style: ["normal", "italic"],
display: "swap",
adjustFontFallback: true,
preload: true,
});
export default function App({ Component, pageProps }: AppProps) {
return (
<main className={`${playfair_display.variable}`}>
<p>test</p>
<Component {...pageProps} />;
</main>
)
}
@import "tailwindcss";
:root {
--background: #ffffff;
--foreground: #171717;
}
@theme inline {
--color-background: var(--background);
--color-foreground: var(--foreground);
--font-sans: var(--font-geist-sans);
--font-mono: var(--font-geist-mono);
}
@theme {
--font-playfair-display: var(--font-playfair-display);
}
@media (prefers-color-scheme: dark) {
:root {
--background: #0a0a0a;
--foreground: #ededed;
}
}
body {
background: var(--background);
color: var(--foreground);
font-family: Arial, Helvetica, sans-serif;
}
However when I used this format it worked:
<div className="font-[family-name:var(--font-playfair-display))]">
False honey antOP
Yeah very
I guess I'll just go with this for now
I suggest you ask for help on the tailwind forum. So far, we've tested next.js and it hasn't had any issues. Tailwind just has a strange problem when declaring custom font variables.
Thank you for sharing the details calmly.
False honey antOP
Alright I'll do that whenever I find the time. Thanks for the help I appreciate it
@Losti! Thank you for sharing the details calmly.
False honey antOP
and yeah of course!