How to use ar.js
Unanswered
Eurasian Wryneck posted this in #help-forum
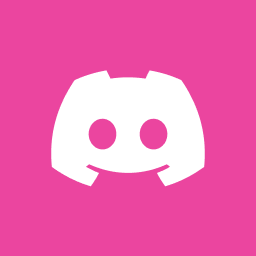
Eurasian WryneckOP
code⇓
11 Replies
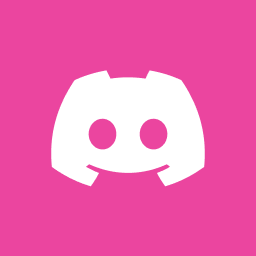
Eurasian WryneckOP
index.tsx
import { useEffect, useRef, useState } from "react";
import * as THREE from "three";
import { GLTFLoader } from "three/examples/jsm/loaders/GLTFLoader";
import { ARToolkitContext, ARToolkitSource, ARMarkerControls } from "ar.js";
const Home = () => {
const videoRef = useRef<HTMLVideoElement | null>(null);
const canvasRef = useRef<HTMLCanvasElement | null>(null);
const [,setModel] = useState<THREE.Object3D | null>(null);
useEffect(() => {
navigator.mediaDevices
.getUserMedia({ video: true })
.then((stream) => {
if (videoRef.current) {
videoRef.current.srcObject = stream;
videoRef.current.play();
}
})
.catch(() => console.log("Error accessing camera."));
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);
camera.position.z = 5;
const renderer = new THREE.WebGLRenderer({ canvas: canvasRef.current! });
renderer.setSize(window.innerWidth, window.innerHeight);
const arToolkitSource = new ARToolkitSource({
sourceType: "webcam",
});
const arToolkitContext = new ARToolkitContext({
cameraParametersUrl: "patt/hiro.patt",
detectionMode: "mono",
});
arToolkitSource.init(() => {
arToolkitContext.init(() => {
camera.projectionMatrix.copy(arToolkitContext.getProjectionMatrix());
});
});
new ARMarkerControls(arToolkitContext, camera, {
type: "pattern",
patternUrl: "patt/patt.hiro",
changeMatrixMode: "cameraTransformMatrix",
});
const loader = new GLTFLoader();
loader.load("/models/model.glb", (gltf: { scene: THREE.Group<THREE.Object3DEventMap>; }) => {
setModel(gltf.scene as THREE.Group);
scene.add(gltf.scene as THREE.Group);
});
const animate = () => {
requestAnimationFrame(animate);
if (arToolkitSource.ready) {
arToolkitContext.update(arToolkitSource.domElement);
}
renderer.render(scene, camera);
};
animate();
}, []);
return (
<div style={{ width: "100vw", height: "100vh" }}>
<video ref={videoRef} style={{ display: "none" }} />
<canvas ref={canvasRef} style={{ width: "100%", height: "100%" }} />
</div>
);
};
export default Home;
ar.js.d.ts
declare module 'ar.js' {
export class ARToolkitSource {
constructor(parameters: { sourceType: string });
init(onReady: () => void): void;
ready: boolean;
domElement: HTMLVideoElement;
}
export class ARToolkitContext {
constructor(parameters: { cameraParametersUrl: string; detectionMode: string });
init(onCompleted: () => void): void;
update(domElement: HTMLVideoElement): void;
getProjectionMatrix(): THREE.Matrix4;
}
export class ARMarkerControls {
constructor(context: ARToolkitContext, camera: THREE.Camera, parameters: { type: string; patternUrl: string; changeMatrixMode: string });
}
}
errorcode⇓
and
Warning: data for page "/_error" (path "/") is 8.49 MB which exceeds the threshold of 128 kB, this amount of data can reduce performance.
See more info here: https://nextjs.org/docs/messages/large-page-data
this code was made by copilot.
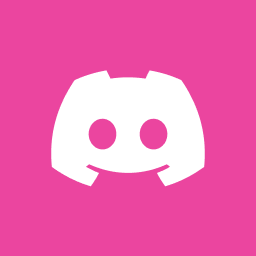
Eurasian WryneckOP
help me
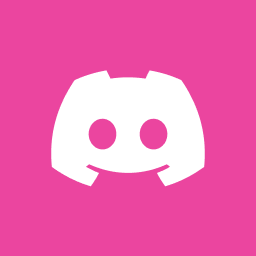
Eurasian WryneckOP
Please give me some advice