Filter data using searchParams
Unanswered
Cape lion posted this in #help-forum
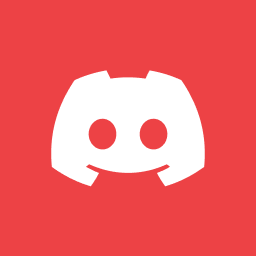
Cape lionOP
Hello, im trying to render dinamically the courses sorting by the course status using search params. But for some reason, it doesn't register the searchParams change. how Can i implement that? or what is the best way to do that? The search params are changing in the client side using nuqs
import React from "react";
import CoursesSelector from "@/components/dashboard/courses/courses-selector";
import CreateNewCourse from "@/components/dashboard/courses/form/create-new-course";
import { auth } from "@/lib/auth/auth";
import { CoursesService } from "@/lib/dashboard/courses/course.service";
import { CoursePreviewCard } from "@/components/dashboard/courses/cards/course-preview-card";
import { unstable_cacheTag as cacheTag } from "next/cache";
import { CourseStatus } from "@prisma/client";
export default async function MyCourses({
searchParams,
}: { searchParams: Promise<{ filterBy: Lowercase<CourseStatus> }> }) {
const session = await auth();
const professorId =
session?.user.role === "TEACHER" ? session?.user.id : undefined;
const resolvedSearchParams = await searchParams;
const filterBy = resolvedSearchParams.filterBy;
return (
<main className="grid grid-cols-3 gap-y-3">
<header className="flex flex-row col-span-3 gap-3 mt-4">
<CoursesSelector />
<CreateNewCourse professorId={professorId} />
</header>
<CoursesList
professorId={professorId}
role={session?.user.role}
filterBy={filterBy}
/>
</main>
);
}
async function CoursesList({ professorId, role, filterBy }: any) {
const courses = await getCoursesList(professorId, filterBy);
return (
<ul className="w-full col-span-3 grid grid-cols-1 md:grid-cols-2 lg:grid-cols-3 gap-3">
{courses.map((course) => (
<li className="col-span-1">
<CoursePreviewCard key={course.id} {...course} role={role} />
</li>
))}
</ul>
);
}
async function getCoursesList(
professorId: string,
filterBy: Lowercase<CourseStatus>,
) {
"use cache";
cacheTag("courses-list");
const response = await CoursesService.getManyByType("MICROCOURSE", {
professorId: professorId,
status: filterBy.toUpperCase() as CourseStatus,
});
if (response.success) return response.data.courses;
return [];
}
1 Reply
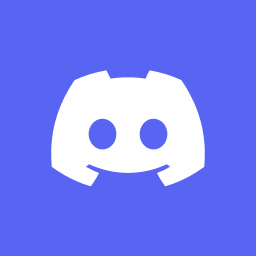
Brown bear
If you want to refresh search params on the server, you’ll have to specify
https://nuqs.47ng.com/docs/options#shallow
shallow: false
in the nuqs options. By default updates are client-side only to avoid spamming the server with requests it may not need.https://nuqs.47ng.com/docs/options#shallow