Revalidate Path
Unanswered
Abyssinian posted this in #help-forum
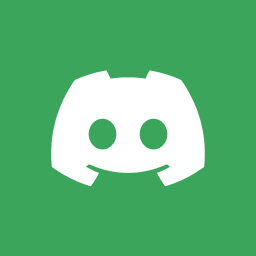
AbyssinianOP
When I submit the form on the /form page, the userName rerenders with the new value due to the revalidatePath function.
The same things happens on the / page as well.
BUT when I update the userName on the /form page the / page doesn't rerender with the new userName.
Why is this happening and how can I achieve this?
app/form/page.tsx
app/page.tsx
app/actions/updateUserName
The same things happens on the / page as well.
BUT when I update the userName on the /form page the / page doesn't rerender with the new userName.
Why is this happening and how can I achieve this?
app/form/page.tsx
import { updateUserName } from "../actions/updateUserName";
const FormPage = async () => {
const response = await fetch("http://localhost:3001/api/getUserName");
const userName = await response.json();
return (
<main className="min-h-screen flex flex-col gap-2 items-center justify-center">
<h1>Form Page</h1>
<h1 className="text-4xl">UserName: {userName}</h1>
<form action={updateUserName} className="flex flex-col gap-2">
<input
className="border-white border rounded-md px-4 py-2 bg-black text-3xl"
type="text"
name="userName"
/>
<button className="bg-black border border-white px-4 py-2 rounded-md ">
Change UserName
</button>
</form>
</main>
);
};
export default FormPage;
app/page.tsx
import { prisma } from "./lib/prisma";
const HomePage = async () => {
const userName = (await prisma.user.findFirst())?.userName ?? "No userName";
const formAction = async (formData: FormData) => {
"use server";
const userName = formData.get("userName")?.toString();
if (!userName) return console.log({ userName });
const response = await prisma.user.update({
where: {
id: 1,
},
data: {
userName,
},
});
console.log({ response });
};
return (
<main className="min-h-screen flex flex-col gap-2 items-center justify-center">
<h1>HomePage</h1>
<h1 className="text-4xl">UserName: {userName}</h1>
<form action={formAction} className="flex flex-col gap-2">
<input
className="border-white border rounded-md px-4 py-2 bg-black text-3xl"
type="text"
name="userName"
/>
<button className="bg-black border border-white px-4 py-2 rounded-md ">
Change UserName
</button>
</form>
</main>
);
};
export default HomePage;
app/actions/updateUserName
"use server";
import { revalidatePath } from "next/cache";
import { prisma } from "../lib/prisma";
// import { redirect } from "next/navigation";
export const updateUserName = async (formData: FormData) => {
const userName = formData.get("userName")?.toString();
if (!userName) return console.log({ userName });
const response = await prisma.user.update({
where: {
id: 1,
},
data: {
userName,
},
});
console.log({ response });
revalidatePath("/", "layout");
};