HELP: Cannot connect NEXT-JS to PostgreSQL
Answered
Asian black bear posted this in #help-forum
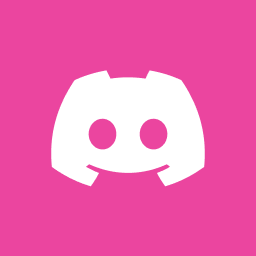
Asian black bearOP
I have one file that connects to postgreSQL
file1.js
in another file Im trying to connect to postgres and actually use it.
what am I doing wrong ??
why
file1.js
import { Client } from 'pg';
const db = new Client({
user: 'user',
host: 'localhost',
database: 'dbName',
password: 'P@ss',
port: 2232,
});
export default db ;
in another file Im trying to connect to postgres and actually use it.
import db from 'file1';
var client = await db.connect();
export async function GET() {
try {
client = await db.connect();
console.log("client is NOW connected!");
} catch (error) {
console.log("client is already connected - "+error ) ; // Terminal responded : client is already connected - Error: Client has already been connected. You cannot reuse a client.
}
try {
console.log("try is fired"); // Terminal responded : try is fired
console.log("client: " + client); // Terminal responded : client: undefined
console.log("db: " + db); // Terminal responded : db: [object Object]
console.log("db.user: " + db.user); // Terminal responded : db.user: user
await client.query(`BEGIN`); // Terminal error "TypeError: Cannot read properties of undefined (reading 'query')"
///////// also tried await client.sql(`BEGIN`); // Terminal error "TypeError: Cannot read properties of undefined (reading 'sql')"
await client.query(`COMMIT`);
return Response.json({ message: 'success' });
} catch (error) {
console.log("error")
await client.query(`ROLLBACK`);
return Response.json({ error }, { status: 500 });
}
}
what am I doing wrong ??
why
client
is still undefined ????9 Replies

the
.connect
call has a return type of voidit just connects to the db
you still use the existing
db
variable
@Yi Lon Ma the `.connect` call has a return type of void
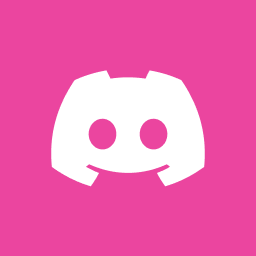
Asian black bearOP
what does that mean? Im following a tutorial that worked for him but not for me. im not sure what is wrong or what happened
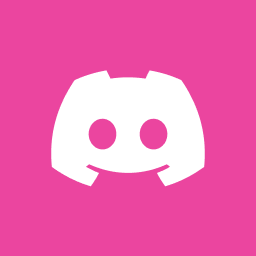
@Asian black bear what does that mean? Im following a tutorial that worked for him but not for me. im not sure what is wrong or what happened

he must be using a different version of pg
Answer

@Yi Lon Ma he must be using a different version of pg
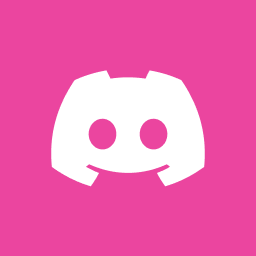
Asian black bearOP
use client correctly, the rest of the code has many
I've also looked into pg page for next and it has similar steps.
what is the right way to do it then ?
await client.query();
.. when I use that it raises an errorI've also looked into pg page for next and it has similar steps.
what is the right way to do it then ?
import pg from 'pg'
const { Client } = pg
const client = new Client()
await client.connect()
const res = await client.query('SELECT $1::text as message', ['Hello world!'])
console.log(res.rows[0].message) // Hello world!
await client.end()

https://node-postgres.com/#getting-started
please refer to the documentation
please refer to the documentation