What's wrong with my library?
Unanswered
Blue-winged Teal posted this in #help-forum
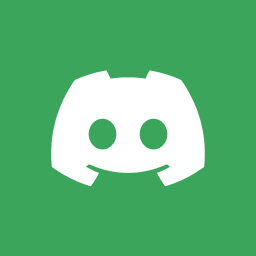
Blue-winged TealOP
inb4: https://github.com/vergonha/homura
I have a small typescript library that allows me to interact with LastFM API. It works fine when running directly with bun or node, for example:
The example above works fine, there's nothing wrong with it. But when I try to use it inside a NextJS function, it throws the following error:
What am I doing wrong? Here are my exports in
Is there a better way to do this?
I have a small typescript library that allows me to interact with LastFM API. It works fine when running directly with bun or node, for example:
// bun run test.ts
import { User } from "homura"
async function Test() {
const _ = new User("<secret.apikey>")
const response = await _.getRecentTracks("atencioso")
if ("error" in response)
return
console.log(response);
// ok!!!
}
Test()
The example above works fine, there's nothing wrong with it. But when I try to use it inside a NextJS function, it throws the following error:
⚠ ./app/page.tsx
Attempted import error: 'homura/dist/User/User' does not contain a default export (imported as 'User').
Import trace for requested module:
./app/page.tsx
./app/page.tsx
Attempted import error: 'homura/dist/User/User' does not contain a default export (imported as 'User').
What am I doing wrong? Here are my exports in
index.d.ts
file:import type iLastFMError from "./helpers/errors/iError";
export declare class Homura {
private readonly _URL;
private readonly _ApiKey;
constructor(key: string);
protected _fetch<T>(_parameters: Record<string, string>): Promise<iLastFMError | T>;
private generateParameters;
}
export { default as Artist } from "./Artist/Artist";
export { default as Album } from "./Album/Album";
export { default as User } from "./User/User";
Is there a better way to do this?
6 Replies
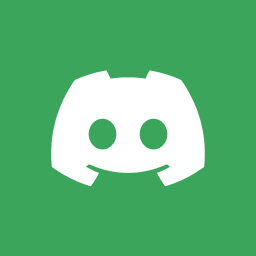
Blue-winged TealOP
Also, here is my NextJS component:
"use client"
import User from "homura"
import { useEffect, useState } from "react"
export default function Page() {
const f = new User("<secret key>")
const [music, setMusic] = useState<string>("")
useEffect(() => {
const get = async () => {
const response = await f.getRecentTracks("atencioso")
if (!("error" in response)) { // ugly, i know. its just a test!
const { name, artist } = response.recenttracks.track[0]
setMusic(`${name} - ${artist}`)
}
}
})
return (
<div>
{music}
</div>
)
}
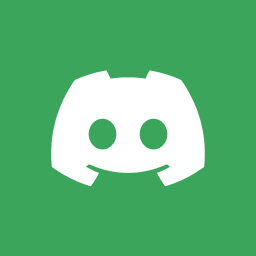
@Blue-winged Teal Also, here is my NextJS component:
ts
"use client"
import User from "homura"
import { useEffect, useState } from "react"
export default function Page() {
const f = new User("<secret key>")
const [music, setMusic] = useState<string>("")
useEffect(() => {
const get = async () => {
const response = await f.getRecentTracks("atencioso")
if (!("error" in response)) { // ugly, i know. its just a test!
const { name, artist } = response.recenttracks.track[0]
setMusic(`${name} - ${artist}`)
}
}
})
return (
<div>
{music}
</div>
)
}
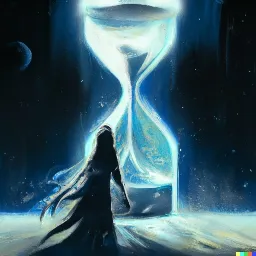
2nd line
change
to
change
import User from "homura"
to
import {User} from "homura"
also, i noticed that you are hard coding your api secret key
this is a bad practice (for example when sharing your code in github, or when asking for help you might forget to hide it, also you might want to change it without changing the code )
so you have to use envirement variables
in your case this won't hide the secret since it's used in the frontend, so make sure (in the documentation) that it is okay to expose it
const f = new User("<secret key>")
this is a bad practice (for example when sharing your code in github, or when asking for help you might forget to hide it, also you might want to change it without changing the code )
so you have to use envirement variables
in your case this won't hide the secret since it's used in the frontend, so make sure (in the documentation) that it is okay to expose it
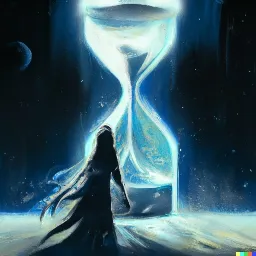
@`${ViNoS}` also, i noticed that you are hard coding your api secret key
`const f = new User("<secret key>")`
this is a bad practice (for example when sharing your code in github, or when asking for help you might forget to hide it, also you might want to change it without changing the code )
so you have to use envirement variables
in your case this won't hide the secret since it's used in the frontend, so make sure (in the documentation) that it is okay to expose it
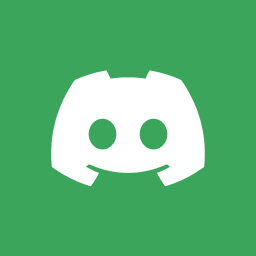
Blue-winged TealOP
Hii, mate!
The LastFM key must be public as API requests will be made in the browser.
Changing the 2nd line gives the same error:
The LastFM key must be public as API requests will be made in the browser.
Changing the 2nd line gives the same error:
Import trace for requested module:
./app/page.tsx
./app/page.tsx
Attempted import error: 'User' is not exported from 'homura' (imported as 'User').
Import trace for requested module:
./app/page.tsx
⚠ ./app/page.tsx
Attempted import error: 'User' is not exported from 'homura' (imported as 'User').
Import trace for requested module:
./app/page.tsx
./app/page.tsx
Attempted import error: 'User' is not exported from 'homura' (imported as 'User')
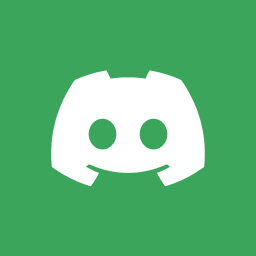
@Blue-winged Teal Hii, mate!
The LastFM key must be public as API requests will be made in the browser.
Changing the 2nd line gives the same error:
Import trace for requested module:
./app/page.tsx
./app/page.tsx
Attempted import error: 'User' is not exported from 'homura' (imported as 'User').
Import trace for requested module:
./app/page.tsx
⚠ ./app/page.tsx
Attempted import error: 'User' is not exported from 'homura' (imported as 'User').
Import trace for requested module:
./app/page.tsx
./app/page.tsx
Attempted import error: 'User' is not exported from 'homura' (imported as 'User')
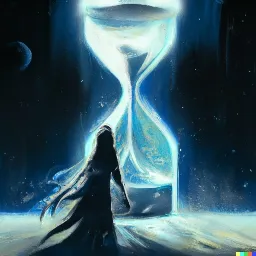
for the secret then it's alright
still better to use env (check next.js docs on envs, in short, you should name it
for the error, I don't have an idea, but here are some steps to debug, try and tell me what u get:
check node modules and see what is actually getting exported from
still better to use env (check next.js docs on envs, in short, you should name it
NEXT_PUBLIC_<whatever>
)for the error, I don't have an idea, but here are some steps to debug, try and tell me what u get:
check node modules and see what is actually getting exported from
homura
btw, are you running the component on the edge runtime ? @Blue-winged Teal