Window.postMessage message is blocked because of Cross-Origin-Opener-Policy policy
Unanswered
Polar bear posted this in #help-forum
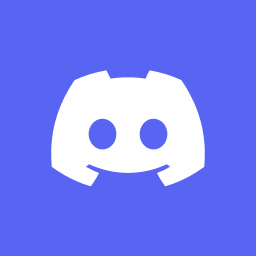
Polar bearOP
During my Google OAuth flow, when the user enters its credential, he gets redirected to the set callback page and this page reads the authentication and gets the Google user info. However, when the callback needs to post the message to the parent window, it gets blocked because of cross-origin opener policy headers. How should I deal with that, any idea?
code:
code:
"use client";
import { LoadingSpinner } from "@/components/my-ui/loading-spinner.server";
import { getGoogleUserInfo } from "@/services/google/google-auth-service.client";
import { useSearchParams } from "next/navigation";
import { useEffect } from "react";
/**
* The GoogleCallbackPage component is used to handle the Google OAuth callback.
* The user is redirected to this page after they have successfully authenticated with Google.
* The page will then send a message to the parent window with the user's information because the Google OAuth flow is done in a popup window.
*/
export default function GoogleCallback() {
const searchParams = useSearchParams();
useEffect(() => {
const authorizationCode = searchParams.get("code");
if (!authorizationCode) {
return;
}
(async () => {
let messageToSendToParentWindow = {};
if (authorizationCode) {
const googleUserInfoQuery = await getGoogleUserInfo(authorizationCode);
const googleUserInfo = await googleUserInfoQuery.json();
// check if the google user info is not empty
if (googleUserInfo.email && googleUserInfo.googleId) {
messageToSendToParentWindow = {
googleUserInfo,
};
}
}
window.opener?.postMessage(
messageToSendToParentWindow,
process.env.NEXT_PUBLIC_WEBSITE_DOMAIN,
);
})();
// eslint-disable-next-line react-hooks/exhaustive-deps
}, []);
return (
<div className="flex min-h-screen flex-col items-center justify-center bg-background">
<LoadingSpinner />
</div>
);
}
1 Reply
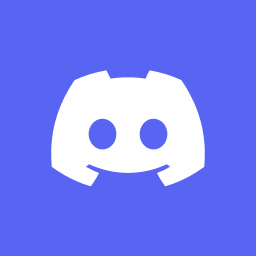
Polar bearOP
bump