Function only runs properly if internal button clicked
Answered
White Shepherd posted this in #help-forum
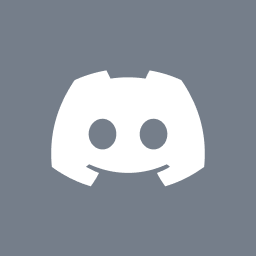
White ShepherdOP
So I have some code like so:
Playground: https://playcode.io/2175341
When I click the "Start" button, all is good. But when I click the "Clicky" button. Nothing works, even though the
import React, { useState, useRef, useEffect } from 'react';
const Stopwatch = (triggerStart, triggerEnd, triggerReset) => {
const [time, setTime] = useState({ minutes: 0, seconds: 0, milliseconds: 0 });
const intervalRef = useRef(0);
const runningTime = useRef(0); // Tracks total elapsed time in milliseconds
const startStopwatch = () => {
if (intervalRef.current) return; // Avoid multiple intervals
console.log('starting stopwatch');
intervalRef.current = setInterval(() => {
runningTime.current += 10; // Increment by 10 ms
const totalMs = runningTime.current;
const minutes = Math.floor(totalMs / 60000);
const seconds = Math.floor((totalMs % 60000) / 1000);
const milliseconds = totalMs % 1000;
setTime({ minutes, seconds, milliseconds });
}, 10); // Update every 10 ms
console.log('finishes setting intervalref');
};
const stopStopwatch = () => {
clearInterval(intervalRef.current);
intervalRef.current = 0;
};
const resetStopwatch = () => {
stopStopwatch();
runningTime.current = 0;
setTime({ minutes: 0, seconds: 0, milliseconds: 0 });
};
useEffect(() => {
return () => stopStopwatch(); // Cleanup on unmount
}, []);
// StartStopwatch doesn't work
useEffect(() => {
if (triggerStart) {
startStopwatch();
}
if (triggerEnd) {
stopStopwatch();
}
if (triggerReset) {
resetStopwatch();
}
}, [triggerStart, triggerEnd, triggerReset]);
return (
<div>
<p>{`${time.minutes}:${time.seconds}:${Math.floor(
time.milliseconds / 10
)}`}</p>
<button onClick={startStopwatch}>Start</button>
<button onClick={stopStopwatch}>Stop</button>
<button onClick={resetStopwatch}>Reset</button>
</div>
);
};
export function App(props) {
const [start, setStart] = useState(false);
function doStart() {
setStart(true);
}
return (
<div className='App'>
<h1>Hello React.</h1>
<Stopwatch triggerStart={start} />
<h2>Start editing to see some magic happen!</h2>
<button onClick={doStart}>Clicky</button>
</div>
);
}
Playground: https://playcode.io/2175341
When I click the "Start" button, all is good. But when I click the "Clicky" button. Nothing works, even though the
startStopwatch
function is runAnswered by White Shepherd
It was the argument structure, needed to put them in ({args}) instead of (args)
2 Replies
Original message was deleted
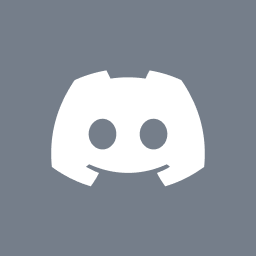
White ShepherdOP
🔎 This post has been indexed in our web forum and will be seen by search engines so other users can find it outside Discord
Love that
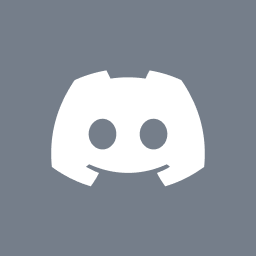
White ShepherdOP
It was the argument structure, needed to put them in ({args}) instead of (args)
Answer