How to Respond to a POST Request by Rendering a Page Route with Access to the Request Payload
Unanswered
Asian black bear posted this in #help-forum
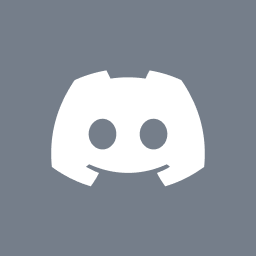
Asian black bearOP
I'm working on an unusual use case where I need to respond to a POST request by rendering a Next.js page. Specifically, I want to receive a POST request at /api/cars that includes car data in the payload. After receiving this request, I need to render the /cars page using the payload data and respond with the resulting HTML. How can I pass the payload data to the page route for server-side rendering and return the rendered HTML in the response to the POST request?
12 Replies
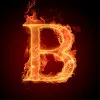
in the app router you can directly get your data without the need of an extra route handler:
Like that you can also use this data (that got returned) to render it inside your HTML (content of your page)
export default async function Page() {
let data = await fetch('https://api.vercel.app/blog')
let posts = await data.json()
return (
<ul>
{posts.map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
)
}
Like that you can also use this data (that got returned) to render it inside your HTML (content of your page)
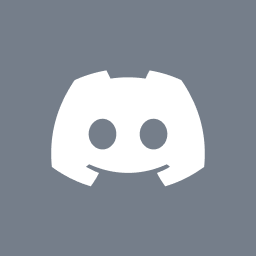
Asian black bearOP
the thing is we don't have an API for the cars, that's why the backend guy will send me a post request that includes the cars data, and I should render the cars page with the payload and then respond with the resulted html
I am new to the company, but they have a legacy backend and want to integrate my cars next js application that way, send me a post request with the needed data, I pass the data to the route and render it, then respond with the rendered html
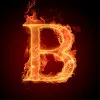
you can't access the body data of your request when someone send a request. All of them are just GET requests. However: you can read the searchParams or the dynamic params itself to render the specific data
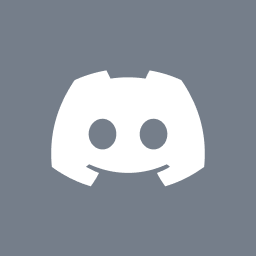
Asian black bearOP
yes, the thing with searchParams is the size of the payload should be small and the payload will be showed on the url.
I found that I can create a custom server in next js, to listed for the post request and respond with the rendered route with passing the payload on the query
dev ? "development" : process.env.NODE_ENV
}
I found that I can create a custom server in next js, to listed for the post request and respond with the rendered route with passing the payload on the query
const { createServer } = require("http");
const { parse } = require("url");
const next = require("next");
const port = parseInt(process.env.PORT || "3000", 10);
const dev = process.env.NODE_ENV !== "production";
const app = next({ dev });
const handle = app.getRequestHandler();
app.prepare().then(() => {
createServer((req, res) => {
const parsedUrl = parse(req.url, true);
const condition = true;
const payload = {};
if (condition) {
app.render(req, res, parsedUrl.pathname, {
...parsedUrl.query,
payload: JSON.stringify(payload),
});
return;
}
handle(req, res, parsedUrl);
}).listen(port);
console.log(
> Server listening at http://localhost:${port} as ${dev ? "development" : process.env.NODE_ENV
}
);
});
what I understand is that way the payload won't be shown on the url and won't have limitation on the size.
in the targeted route I can access to it like that
in the targeted route I can access to it like that
import { SearchParams } from "next/dist/server/request/search-params";
export default async function Home({ searchParams }: { searchParams: SearchParams }) {
const search = await searchParams;
const payload = JSON.parse(search.payload as string);
return <div>{JSON.stringify(payload)}</div>;
}
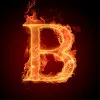
that doesn't looks like nextjs. Keep in mind, that you need a serverfull enviorement to support that
most of the time only an id to the data will be shared. Like that you can request with the ID the needed data from your server
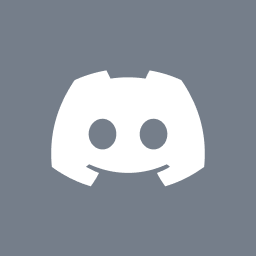
Asian black bearOP
it's a next js custom server
https://nextjs.org/docs/pages/building-your-application/configuring/custom-server
https://nextjs.org/docs/pages/building-your-application/configuring/custom-server
Yeah, I talked about the lead to store the payload in a db and share just the id with the route, but he said we prefer to do it without a need for db
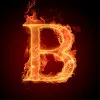
I am unfamiliar with the use of a custom server. All the best for your project
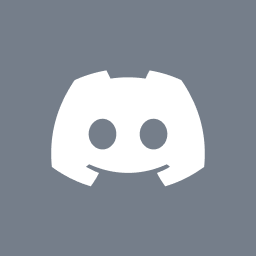
Asian black bearOP
Thanks for the helpt