Dynamic Route Navigation on Production
Unanswered
West African Crocodile posted this in #help-forum
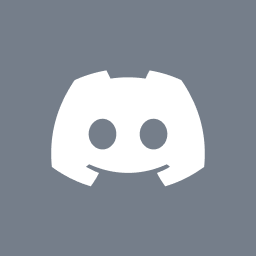
West African CrocodileOP
I have made a deploy of a NextJS 14 (with App Routing) project in Vercel, which works well locally but in production the dynamic routes update the URL well but instead of going to the dynamic page it takes me to the main page
3 Replies
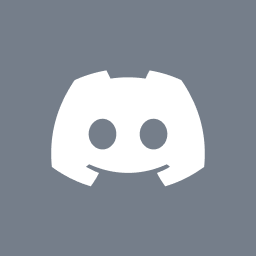
West African CrocodileOP
This is the code:
// app/random/page.tsx
"use client";
import { useState } from "react";
import Link from "next/link";
export default function RandomPage() {
const [input, setInput] = useState("");
return (
<div className="flex flex-col items-center justify-center min-h-screen bg-gray-100">
<h1 className="text-3xl font-bold mb-6">Enter a Value</h1>
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)}
placeholder="Type something..."
className="border border-gray-300 rounded px-4 py-2 mb-4 focus:outline-none focus:ring-2 focus:ring-blue-500"
aria-label="Input for dynamic page"
/>
<Link
href={input ? `/random/${input}` : "/random"}
className="bg-blue-500 text-white px-4 py-2 rounded hover:bg-blue-600 transition"
>
Go to Dynamic Page
</Link>
</div>
);
}
// app/random/[inputs]/page.tsx
"use client";
import Link from "next/link";
import { useParams } from "next/navigation";
export default function DynamicPage() {
const params = useParams();
const inputs = params?.inputs as string | undefined;
return (
<div className="flex flex-col items-center justify-center min-h-screen bg-gray-100">
<h1 className="text-3xl font-bold mb-6">Dynamic Page</h1>
{inputs ? (
<p className="text-xl mb-4">You entered: {inputs}</p>
) : (
<p className="text-xl mb-4">No input provided</p>
)}
<Link
href="/random"
className="bg-blue-500 text-white px-4 py-2 rounded hover:bg-blue-600 transition"
>
Go Back
</Link>
</div>
);
}
Why would it work locally but redirect me to the main page instead on production when deployed to Vercel? Thanks!