Cache non-page component
Answered
filip posted this in #help-forum
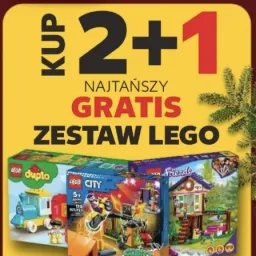
filipOP
Hi, i was wondering how to not use 'use client'
and somehow cache the fatched data so the component is rendered on the server.
Here is the fragment of my code:
the filter tab appears on a page dedicated for searching products. I am confused how and which components to try to cache. The filter tab i just want to revalidate like once a week maybe, but the products grid needs to be dynamic and rendered on the client side (i think).
and somehow cache the fatched data so the component is rendered on the server.
Here is the fragment of my code:
components/products/filtertab/FilterTab.jsx
'use client'
import React, { useEffect, useState } from 'react'
import FilterCheck from './FilterCheck'
const FilterTab = ({ handleBrand, curBrand, handleCategory, curCategory, isDrop = false }) => {
const [brandsList, setBrandsList] = useState([])
const [categoriesList, setCategoriesList] = useState([])
const fetchData = async () => {
const res = await fetch("api/products/filters", { method: "GET" })
const result = await res.json()
setBrandsList(result?.data?.brands)
setCategoriesList(result?.data?.categories)
}
useEffect(() => {
fetchData()
}, [])
the filter tab appears on a page dedicated for searching products. I am confused how and which components to try to cache. The filter tab i just want to revalidate like once a week maybe, but the products grid needs to be dynamic and rendered on the client side (i think).
Answered by American black bear
fetch everything you want on server than pass it is a props
8 Replies
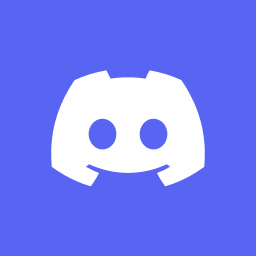
American black bear
fetch everything you want on server than pass it is a props
Answer
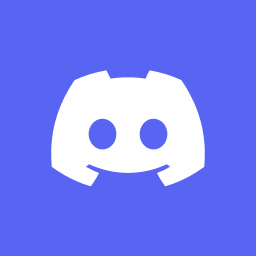
American black bear
it is probably best to never fetch data in useEffect if you mustn't
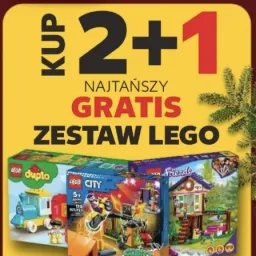
filipOP
even search for products i should code without useEffect? i am pretty new to this
// app/page.js or app/(your-component)/page.js
async function fetchData() {
const res = await fetch('https://jsonplaceholder.typicode.com/posts/1', {
cache: 'no-store', // Optional: 'no-store' for SSR, 'force-cache' for SSG
});
return res.json();
}
export default async function Page() {
const data = await fetchData();
return (
<div>
<h1>{data.title}</h1>
<p>{data.body}</p>
</div>
);
}
so something like this?
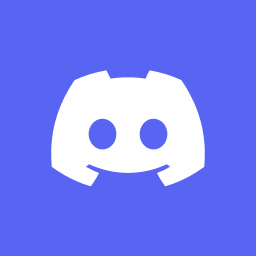
American black bear
for search you should be using searchParams, and then fetch the page based on search params on server.
it is completly normal to be confused by this since some older guides on react and next.js use useEffect for a lot of things where it shouldn't be used in
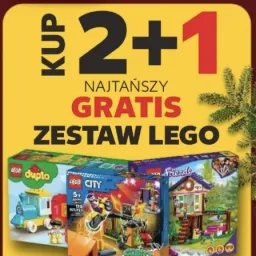
filipOP
thank you for your answers. I have one more question, how would i rerender the page when products change based on search?
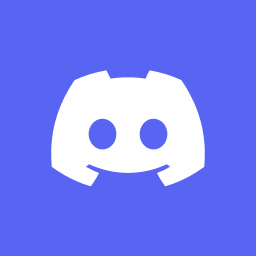
American black bear
the page rerenders itself if you use router.replace when changing the search params