Docker next.js production deployment error, "Only plain objects, and a few built-ins, can be passed"
Unanswered
Asiatic Lion posted this in #help-forum
Original message was deleted.
2 Replies
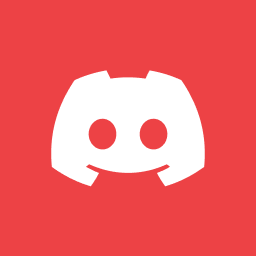
Asiatic Lion


/**
* @function createUserAccount
* @description ran from the onboarding page after signing up with an email or with
* an auth provider (Google, Clever, or Microsoft). Adds all relevant user info to the database.
*
* @param {CreateUserSubmitData} data the data provided by the user on the onboarding page.
* @returns {Promise<{success: boolean; message: string; user: {id: string | number; specificUserId: string | number} | null }>}
* success status, a response message, and the session user object
*/
export const createUserAccount = async (
data: CreateUserSubmitData
): Promise<{
success: boolean;
user: { id: string | number; specificUserId: string | number } | null;
message: string;
}> => {
try {
if (!data.emailSignUp) {
const session = await auth();
if (!session) {
return { success: false, message: 'Unable to connect', user: null };
}
} else if (!data.password) {
return { success: false, user: null, message: 'Missing password entry' };
}
const response: Response = await fetch(`${baseUrl}/api/auth/create-user`, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(data),
});
const result: {
success: boolean;
user: { id: string | number; specificUserId: string | number } | null;
message: string;
} = await response.json();
if (!response.ok || !result.user) {
return {
success: false,
user: null,
message: result.message || 'Failed to create user account',
};
}
return result;
} catch (err) {
console.error('Fetch error:', err);
return {
success: false,
user: null,
message: 'Something went wrong when creating your account',
};
}
};