How do I do the equivalent of getStaticProps in app router?
Unanswered
Tan posted this in #help-forum
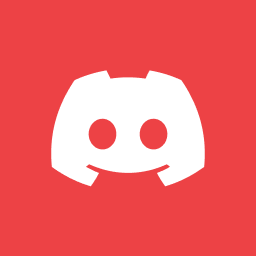
TanOP
I've been able to use generateStaticParams to create routes at build time, how do I populate my pages with data at build time and create a partly static site?
2 Replies
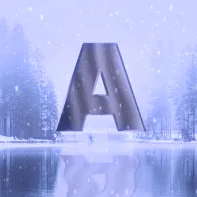
You've to fetch data in server component, pass to client component
// app/posts/[id]/page.tsx
// Function to fetch data
async function getPostData(id: string) {
const res = await fetch(`https://api.example.com/posts/${id}`, {
cache: 'force-cache', // Ensures data is fetched at build time
});
if (!res.ok) {
throw new Error('Failed to fetch data');
}
return res.json();
}
export default async function PostPage({ params }: { params: { id: string } }) {
const data = await getPostData(params.id);
return (
// Optionally pass to client component
<div>
<h1>{data.title}</h1>
<p>{data.content}</p>
</div>
);
}
// Static parameters generation for static paths
export async function generateStaticParams() {
// Fetch and return the static paths
return [
{ id: '1' },
{ id: '2' },
{ id: '3' },
];
}