Problem with a middleware
Answered
Yacare Caiman posted this in #help-forum
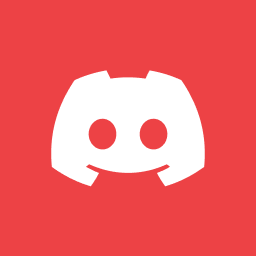
Yacare CaimanOP
I have made this middleware following the NextJS authentication documentation and I want that even if the user is logged in he can access to the public routes.
import { NextRequest, NextResponse } from "next/server";
import { decrypt } from "@/app/lib/session";
import { cookies } from "next/headers";
// Protected routes and public routes
const protectedRoutes = ["/dashboard", "/dashboard/:path*"];
const publicRoutes = ["/auth/login", "/"];
export default async function middleware(request: NextRequest) {
// Check if the current route is protected or public
const path = request.nextUrl.pathname;
const isProtectedRoute = protectedRoutes.includes(path);
const isPublicRoute = publicRoutes.includes(path);
// Decrypt the session from the cookie
const cookie = (await cookies()).get("session")?.value;
const session = await decrypt(cookie);
// Redirect to 404 if the user is not authenticated and the route is protected
if (isProtectedRoute && !session?.userId) {
return NextResponse.rewrite(new URL("/404", request.url));
}
// Redirect to dashboard if the user is authenticated
if (
isPublicRoute &&
session?.userId &&
!request.nextUrl.pathname.startsWith("/dashboard")
) {
return NextResponse.redirect(new URL("/dashboard", request.url));
}
return NextResponse.next();
}
// Routes Middleware should not run on
export const config = {
matcher: ["/((?!api|_next/static|_next/image|.*\\.png$).*)"],
};
Answered by Double-striped Thick-knee
remove the part where you're redirecting user when he's authenticated
2 Replies
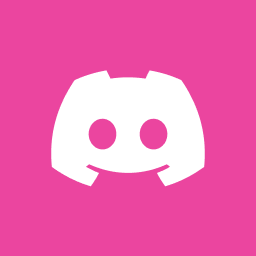
Double-striped Thick-knee
remove the part where you're redirecting user when he's authenticated
Answer
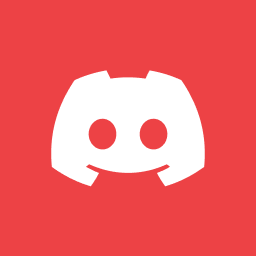
Yacare CaimanOP
I'm stupid, thanks