how to good handle error ?
Unanswered
American Chinchilla posted this in #help-forum
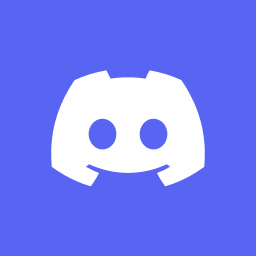
American ChinchillaOP
I'm having a bit of trouble understanding error handling for my API.
Should I put my try-catch in my API class or in each component?
If I understand correctly, let's say I login my user and the credentials are wrong, the server will return a 401 invalid credential error.
However, in the console log it will show me :
auth.js:6 : POST http://localhost:3333/api/auth/login 400 (Bad Request)
Now this is a ‘normal’ error and so I have to add it to my store Error for the message.
What are the best practices for building my exception catch?
I have a :
and a store zustand :
Should I put my try-catch in my API class or in each component?
If I understand correctly, let's say I login my user and the credentials are wrong, the server will return a 401 invalid credential error.
However, in the console log it will show me :
auth.js:6 : POST http://localhost:3333/api/auth/login 400 (Bad Request)
Now this is a ‘normal’ error and so I have to add it to my store Error for the message.
What are the best practices for building my exception catch?
I have a :
export default class AuthApi extends Api{
static async login(credentials){
const response = await fetch(`${this.baseUrl}/api/auth/login`, {
method: ‘POST’,
headers: this.getHeaders(),
body: JSON.stringify(credentials)
});
if (!response.ok) {
const errorData = await response.json();
return {type: ‘error’, message: errorData.message || response.statusText}
}
return await response.json();
}
and a store zustand :
const useErrorStore = createSelectors(create((set) => ({
error: {
type: null,
message: null,
},
setError: ({ type, message }) => set({ error: { type, message } }),
clearError: () => set({ error: { type: null, message: null } }),
})));
1 Reply
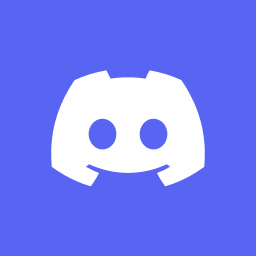
American ChinchillaOP
and to send api :
const handleSubmit = async (e) => {
e.preventDefault();
setLoading(true);
try {
const response = await AuthApi.login({ email, password });
setToken(response.token);
setUser(response.user);
setAuthenticated(true);
setError({ type: 'success', message: 'Logged in successfully' });
} catch (error) {
setError({ type: 'error', message: error.message });
} finally {
setLoading(false);
}
};