generateStaticParams() weird behavior with fetching
Answered
filip posted this in #help-forum
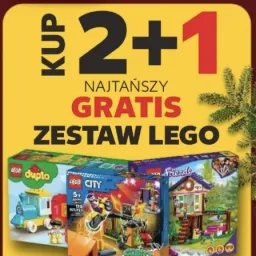
filipOP
Hello, I am having an issue with generateStaticParams().
The problem is that I've changed some code in my api route that I am fetching data from, but it seems the generateStaticParams doesn't fetch the new data after change. Even after i restart the dev server, generateStaticParams still displays the old data and doesn't call fetch.
Here is the code:
The problem is that I've changed some code in my api route that I am fetching data from, but it seems the generateStaticParams doesn't fetch the new data after change. Even after i restart the dev server, generateStaticParams still displays the old data and doesn't call fetch.
Here is the code:
.../[slug]/page.jsx
import { notFound } from 'next/navigation'
import ProductView from '@components/products/filtertab/ProductView'
export async function generateStaticParams() {
//fetch all paths
const res = await fetch(`${process.env.NEXT_PUBLIC_API_URL}/api/products/all/paths`)
const result = await res.json()
const products = result ? result.data : undefined
console.log("products after fetching: -----------------------------------------")
console.log(products)
if (!products) {
return {}
}
// Generate all product paths for static generation
return products.map((product) => ({
slug: product.path,
}))
}
export default async function ProductPage({ params }) {
const { slug } = params
console.log("trying to fetch")
const productPromise = fetch(`${process.env.NEXT_PUBLIC_API_URL}/api/products/single`, { method: "GET" })
console.log("fetched")
// fetch product
const { product } = await productPromise
// handle case where product is not found
if (!product) {
notFound()
}
return (
<ProductView product={product} relatedProducts={relatedProducts} />
)
}
Answered by B33fb0n3
The fetch requests are cached by default. Change it to:
Also keep in mind, that the generateStaticParams are only called during build time. So only when you build your project the function will be called
fetch('https://...', { cache: 'no-cache' })
Also keep in mind, that the generateStaticParams are only called during build time. So only when you build your project the function will be called
6 Replies
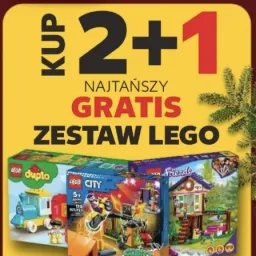
filipOP
and more code:
When i access the api from the browser it displays properly:
but from the generateStaticPaths (console logs):
.../api/products/all/paths/route.js
import pool from "@utils/sqldb";
import { NextResponse } from "next/server";
export const GET = async (req) => {
try {
const headers = new Headers({
'Cache-Control': 'no-store', // Disables caching
});
const [q] = await pool.query("SELECT REPLACE(LOWER(name), ' ', '-') AS `name` FROM Products;")
console.log('q---------------------------------------\n\n')
console.log(q)
console.log('\n\n--------------------')
return NextResponse.json({ data: q }, { status: 200, headers: headers })
} catch (error) {
console.log(error)
return NextResponse.json({ error: "Error fetching data" }, { status: 500 })
}
}
When i access the api from the browser it displays properly:
{"data":[{"name":"art1"},{"name":"art12314"},{"name":"motor123421"},{"name":"test1"}]}
but from the generateStaticPaths (console logs):
GET /api/products/all/paths 200 in 295ms
trying to fetch
fetched
GET /sklep/test1 404 in 138ms
products after fetching: -----------------------------------------
[
{ "REPLACE(LOWER(name), ' ', '-')": 'art1' },
{ "REPLACE(LOWER(name), ' ', '-')": 'art12314' },
{ "REPLACE(LOWER(name), ' ', '-')": 'motor123421' },
{ "REPLACE(LOWER(name), ' ', '-')": 'test1' }
]
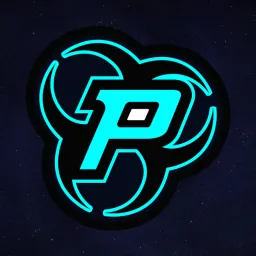
What version of next are you using?
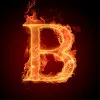
The fetch requests are cached by default. Change it to:
Also keep in mind, that the generateStaticParams are only called during build time. So only when you build your project the function will be called
fetch('https://...', { cache: 'no-cache' })
Also keep in mind, that the generateStaticParams are only called during build time. So only when you build your project the function will be called
Answer
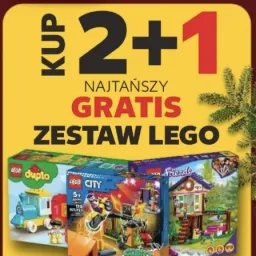
filipOP
thank you, i thought it works differently when using local dev server
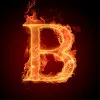
Sure thing