Trouble with adding user id to NextAuth session
Unanswered
Gharial posted this in #help-forum
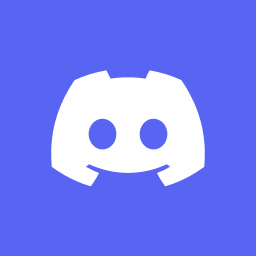
GharialOP
I need my users's id in my session, so I added a callback to the nextauth options.
This works fine except that ESLint seems to hate it for some reason and I cant figure out why because the error message is giant and confusing.
This works fine except that ESLint seems to hate it for some reason and I cant figure out why because the error message is giant and confusing.
import GoogleProvider from "next-auth/providers/google"
import PostgresAdapter from "@auth/pg-adapter"
import { Pool } from "pg"
type SessionProps = {
session: {
user: {
id: string;
name: string;
email: string;
};
};
user: {
id: string;
};
};
const pool = new Pool({
host: process.env.POSTGRES_HOST,
user: process.env.POSTGRES_USER,
password: process.env.POSTGRES_PASSWORD,
database: process.env.POSTGRES_DATABASE,
max: 20,
idleTimeoutMillis: 30000,
connectionTimeoutMillis: 2000,
ssl: {rejectUnauthorized: false} // This is for local development only
});
const googleProvider = GoogleProvider({
clientId: process.env.GOOGLE_CLIENT_ID || "",
clientSecret: process.env.GOOGLE_CLIENT_SECRET || ""
});
const callbacks = {
async session({ session, user}: SessionProps) {
console.log('user', user);
if (session?.user) session.user.id = user.id;
return session;
}
}
export const authOptions = {
adapter: PostgresAdapter(pool),
providers: [googleProvider],
callbacks: callbacks
}
9 Replies
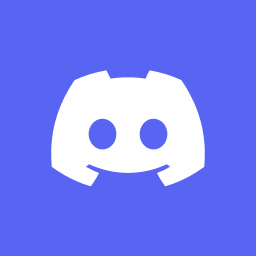
GharialOP
this runs fine, but eslint throws this:
Type error: Argument of type '[{ adapter: Adapter; providers: OAuthConfig<GoogleProfile>[]; callbacks: { session({ session, user }: SessionProps): Promise<{ user: { id: string; name: string; email: string; }; }>; }; }]' is not assignable to parameter of type 'GetServerSessionParams<GetServerSessionOptions>'.
Type '[{ adapter: Adapter; providers: OAuthConfig<GoogleProfile>[]; callbacks: { session({ session, user }: SessionProps): Promise<{ user: { id: string; name: string; email: string; }; }>; }; }]' is not assignable to type '[GetServerSessionOptions]'.
Type '{ adapter: Adapter; providers: OAuthConfig<GoogleProfile>[]; callbacks: { session({ session, user }: SessionProps): Promise<{ user: { id: string; name: string; email: string; }; }>; }; }' is not assignable to type 'GetServerSessionOptions'.
Type '{ adapter: Adapter; providers: OAuthConfig<GoogleProfile>[]; callbacks: { session({ session, user }: SessionProps): Promise<{ user: { id: string; name: string; email: string; }; }>; }; }' is not assignable to type '{ callbacks?: (Omit<Partial<CallbacksOptions<Profile, Account>> | undefined, "session"> & { session?: ((params: { session: Session; token: JWT; user: AdapterUser; } & { ...; }) => any) | undefined; }) | undefined; }'.
Types of property 'callbacks' are incompatible.
Type '{ session({ session, user }: SessionProps): Promise<{ user: { id: string; name: string; email: string; }; }>; }' is not assignable to type 'Omit<Partial<CallbacksOptions<Profile, Account>> | undefined, "session"> & { session?: ((params: { session: Session; token: JWT; user: AdapterUser; } & { ...; }) => any) | undefined; }'. Type '{ session({ session, user }: SessionProps): Promise<{ user: { id: string; name: string; email: string; }; }>; }' is not assignable to type '{ session?: ((params: { session: Session; token: JWT; user: AdapterUser; } & { newSession: any; trigger: "update"; }) => any) | undefined; }'.
Types of property 'session' are incompatible.
Type '({ session, user }: SessionProps) => Promise<{ user: { id: string; name: string; email: string; }; }>' is not assignable to type '(params: { session: Session; token: JWT; user: AdapterUser; } & { newSession: any; trigger: "update"; }) => any'.
Types of parameters '__0' and 'params' are incompatible.
Type '{ session: Session; token: JWT; user: AdapterUser; } & { newSession: any; trigger: "update"; }' is not assignable to type 'SessionProps'.
The types of 'session.user' are incompatible between these types.
Type '{ name?: string | null | undefined; email?: string | null | undefined; image?: string | null | undefined; } | undefined' is not assignable to type '{ id: string; name: string; email: string; }'.
Type 'undefined' is not assignable to type '{ id: string; name: string; email: string; }'.
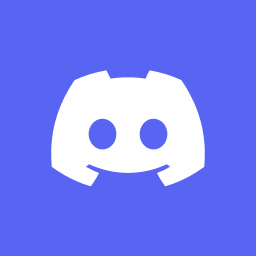
GharialOP
i think this is the same problem: https://github.com/nextauthjs/next-auth/issues/7132
but im afraid of switching to whole other version of nextauth to fix it, especially a beta one
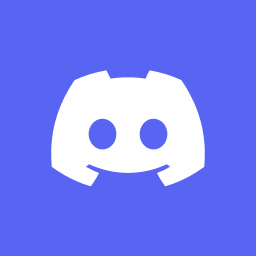
GharialOP
okay guess im just gonna trying upgrading to beta since i cant fix it
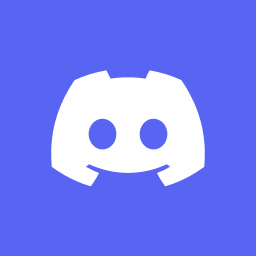
GharialOP
think im almost there, but still gettting this issue on the "callbacks" part of the nextauth config
./auth.ts:46:2
Type error: Type '{ session: ({ session, user }: SessionProps) => { user: { id: string; name?: string; email: string; image?: string; }; }; }' is not assignable to type '{ signIn?: ((params: { user: AdapterUser | User; account:
Account | null; profile?: Profile | undefined; email?: { verificationRequest?: boolean | undefined; } | undefined; credentials?: Record<...> | undefined; }) => Awaitable<...>) | undefined; redirect?: ((params: { ...; }) => Awaitable<...>) | undefined; session...'.
Type '{ session: ({ session, user }: SessionProps) => { user: { id: string; name?: string; email: string; image?: string; }; }; }' is not assignable to type '{ signIn?: ((params: { user: AdapterUser | User; account: Account |
null; profile?: Profile | undefined; email?: { verificationRequest?: boolean | undefined; } | undefined; credentials?: Record<...> | undefined; }) => Awaitable<...>) | undefined; redirect?: ((params: { ...; }) => Awaitable<...>) | undefined; session...'.
The types returned by 'session(...)' are incompatible between these types.
Type '{ user: { id: string; name?: string | undefined; email: string; image?: string | undefined; }; }' is not assignable to type 'Awaitable<Session | DefaultSession>'.
Property 'expires' is missing in type '{ user: { id: string; name?: string | undefined; email: string; image?: string | undefined; }; }' but required in type 'DefaultSession'.
44 | adapter: PostgresAdapter(pool),
45 | providers: [googleProvider],
> 46 | callbacks: callbacks
| ^
47 | });
48 |
49 |
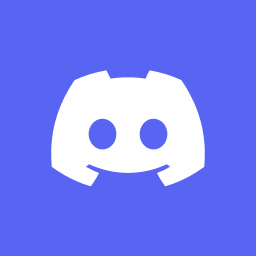
GharialOP
another error caused by the same thing
./app/account/page.tsx:9:17
Type error: Type 'import("Y:/Projects/Websites/votelo/node_modules/@auth/core/types").Session' is not assignable to type 'Session'.
Types of property 'user' are incompatible.
Type 'User | undefined' is not assignable to type '{ name?: string | undefined; id?: string | undefined; } | undefined'.
Type 'User' is not assignable to type '{ name?: string | undefined; id?: string | undefined; }'.
Types of property 'name' are incompatible.
Type 'string | null | undefined' is not assignable to type 'string | undefined'.
Type 'null' is not assignable to type 'string | undefined'.
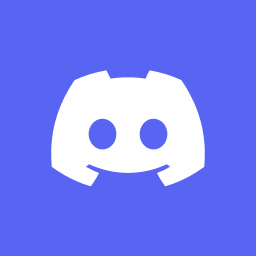
GharialOP
new ./auth.js file:
import NextAuth from "next-auth"
import GoogleProvider from "next-auth/providers/google"
import PostgresAdapter from "@auth/pg-adapter"
import { Pool } from "pg"
type SessionProps = {
session: {
user: {
id: string;
name?: string;
email: string;
image?: string;
};
};
user: {
id: string;
};
};
const pool = new Pool({
host: process.env.POSTGRES_HOST,
user: process.env.POSTGRES_USER,
password: process.env.POSTGRES_PASSWORD,
database: process.env.POSTGRES_DATABASE,
max: 20,
idleTimeoutMillis: 30000,
connectionTimeoutMillis: 2000,
ssl: {rejectUnauthorized: false} // This is for local development only
});
const googleProvider = GoogleProvider({
clientId: process.env.GOOGLE_CLIENT_ID || "",
clientSecret: process.env.GOOGLE_CLIENT_SECRET || ""
});
const callbacks = {
session: ({ session, user }: SessionProps) => {
session.user.id = user.id;
return session;
}
}
export const { auth, handlers, signIn, signOut } = NextAuth({
adapter: PostgresAdapter(pool),
providers: [googleProvider],
callbacks: callbacks
});
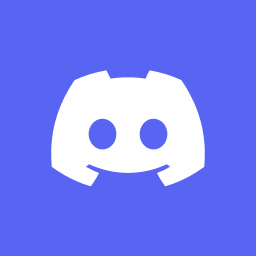
GharialOP
I tried adding this
which i think is supposed to modify the defined type for session to include the user id
then I inserted
"next-auth.d.ts"
file: import NextAuth, { DefaultSession } from 'next-auth'
declare module 'next-auth' {
interface Session {
user: {
id: string;
} & DefaultSession['user']
}
}
which i think is supposed to modify the defined type for session to include the user id
then I inserted
"next-auth.d.ts"
into the "include" array under tsconfig.json
, but that doesn't seem to make any difference.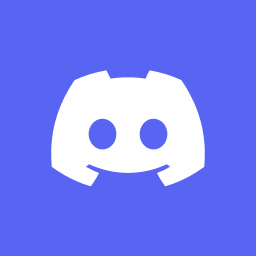
GharialOP
there's gotta be a way to fix this, or an example of how to do it right somewhere