CORS Issue calling get method
Answered
Horned oak gall posted this in #help-forum
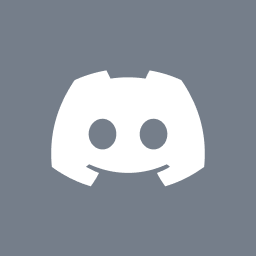
Horned oak gallOP
Curious as how to solve this.
API get call:
Page it is being called:
API get call:
export default async function getAllReservationsForIdentity(identityId, token) {
const res = await fetch( `${process.env.NEXT_PUBLIC_HADES_URL}/reservation/identity/${identityId}`, {
method: "GET",
cache: "no-cache",
headers: {
"Content-Type": "application/json",
"Authorization": "Bearer " + token,
}
})
if (!res.ok) throw new Error('Failed to fetch reservations')
console.log('msg res')
console.log(res)
return res.json()
}
Page it is being called:
'use client';
import {useSession} from "next-auth/react";
import getAllReservationsForIdentity from "@/lib/getAllReservationsForIdentity";
export default function ReservationsPage ({ params }: { params: { identityId: string, string }}) {
const { data: session } = useSession();
let reservationData: Reservation[] = [];
const getReservationsData = async () => {
reservationData = await getAllReservationsForIdentity(session?.user.identityId, session?.user.backendToken);
}
getReservationsData();
console.log(reservationData);
// const reservationsData: Promise<Reservation[]> = await getAllReservationsForIdentity(params.identityId);
return (
<h4>Hi</h4>
)
}

Answered by Horned oak gall
https://www.baeldung.com/spring-cors
^Scroll down to the "cors with spring security" section
^Scroll down to the "cors with spring security" section
26 Replies
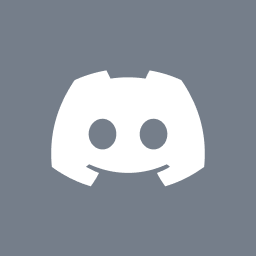
Horned oak gallOP
So I tried adding a middleware.ts and it didn't help, still ran into CORS
I think this may be because of the fact that the fetch methods are just functions and not API routes?(I don't want to have to add them under the API directory if avoidable)


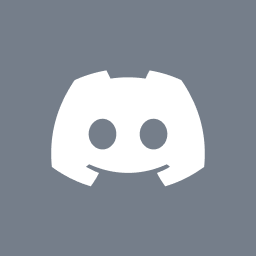
Horned oak gallOP
bump
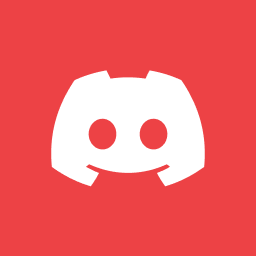
Cape lion
bro you are using external server at 8080? which server is it ? express or some other framework
if you are using node js express then install
npm i cors
app.use(cors())
this can solve the issue you can use wild card like "" or you can specify domain as well
make sure that if you are sharing cookies then you shouldn't use wild card like "" because it will not work as expexted
if you are using node js express then install
npm i cors
app.use(cors())
this can solve the issue you can use wild card like "" or you can specify domain as well
make sure that if you are sharing cookies then you shouldn't use wild card like "" because it will not work as expexted
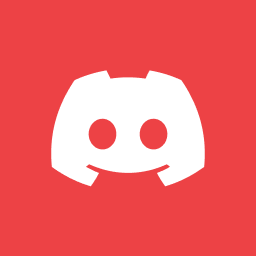
Cape lion
in spring boot
import cors origin inside your controller
import cors origin inside your controller
i have recently done a project on it
to correct cors policy import cors origin packages in your each controller
like for product controler
before your class name import it like
to correct cors policy import cors origin packages in your each controller
like for product controler
before your class name import it like
check this out bro @Horned oak gall
3000 is my react server so i added this make sure not to hardcode like i did if you want to use it for production then config a env variable
and all set
3000 is my react server so i added this make sure not to hardcode like i did if you want to use it for production then config a env variable
and all set

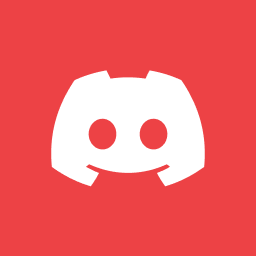
Cape lion
dude kindly share me one of a controller
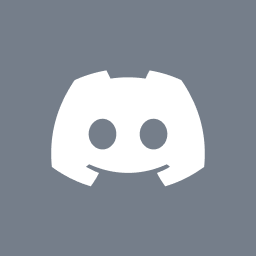
Horned oak gallOP
I think because my security config is intercepting it before it hits the controller
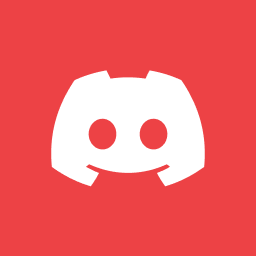
Cape lion
show me the security configs too
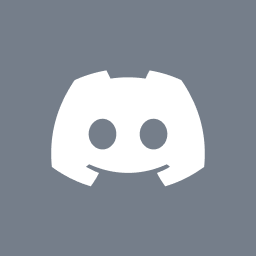
Horned oak gallOP
package com.reeftrader.Hades.reservation;
import com.reeftrader.Hades.listing.Listing;
import com.reeftrader.Hades.listing.ListingRequest;
import com.reeftrader.Hades.listing.ListingResponse;
import com.reeftrader.Hades.listing.ListingService;
import lombok.RequiredArgsConstructor;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@CrossOrigin(origins = "http://localhost:3000")
@RestController
@RequestMapping("/reservation")
@RequiredArgsConstructor
public class ReservationController {
private final ReservationService reservationService;
@GetMapping("identity/{identityId}")
public ResponseEntity<List<Reservation>> getReservationsForIdentity (@PathVariable Integer identityId) {
return ResponseEntity.ok(reservationService.getReservationsForIdentity(identityId));
}
}
package com.reeftrader.Hades.security.config;
import com.reeftrader.Hades.user.User;
import com.reeftrader.Hades.user.UserService;
import jakarta.servlet.Filter;
import lombok.AllArgsConstructor;
import lombok.RequiredArgsConstructor;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationProvider;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.security.web.SecurityFilterChain;
import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
@Configuration
@EnableWebSecurity
@RequiredArgsConstructor
public class WebSecurityConfig {
private final JwtAuthFilter jwtAuthFilter;
private final AuthenticationProvider authenticationProvider;
private final static List<UserDetails> APPLICATION_USERS = Arrays.asList(
new org.springframework.security.core.userdetails.User(
"email.com",
"password",
Collections.singleton(new SimpleGrantedAuthority("ROLE_ADMIN"))
)
);
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http
.csrf()
.disable()
.authorizeHttpRequests()
.requestMatchers("/user/**", "/event", "/listing/event/**")
.permitAll()
.anyRequest()
.authenticated()
.and()
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.authenticationProvider(authenticationProvider)
.addFilterBefore(jwtAuthFilter, UsernamePasswordAuthenticationFilter.class);
return http.build();
}
}
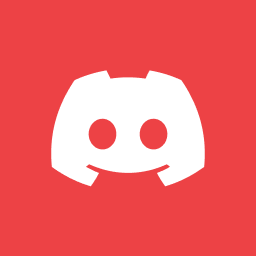
Cape lion
bro before security config class import the cors origin as well and try
follow him maybe help you out
@Horned oak gall
https://stackoverflow.com/questions/49049312/cors-error-when-connecting-local-react-frontend-to-local-spring-boot-middleware
try these bro cors mostly comes fot java
the problem is not with nextjs code its from java so try findings a solutions in that possibly you will find a work around
try these bro cors mostly comes fot java
the problem is not with nextjs code its from java so try findings a solutions in that possibly you will find a work around
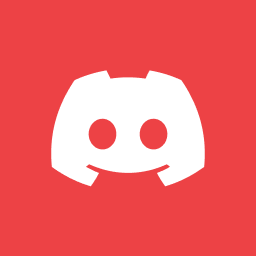
@Cape lion https://youtu.be/phs90_s0Mjk?si=BggPIx2vsu6SMMZL
from 8:44
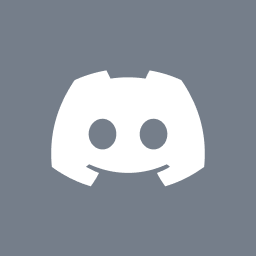
Horned oak gallOP
Still failing after adding this in... looking at the rest of the video n such to see if anything stands out
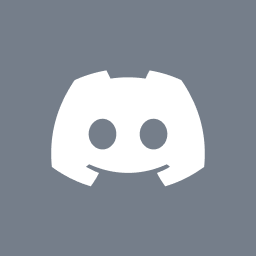
Horned oak gallOP
Solution found
configuration.setAllowedHeaders(Arrays.asList("*"));
^Needs to also be added to the corsConfigurationSource() method shown in the video above
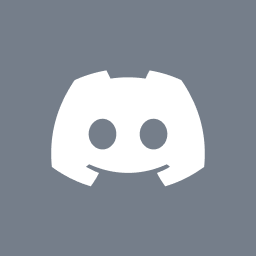
Horned oak gallOP
https://www.baeldung.com/spring-cors
^Scroll down to the "cors with spring security" section
^Scroll down to the "cors with spring security" section
Answer
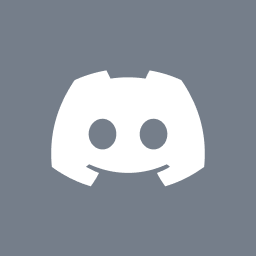
Horned oak gallOP
Thanks for the help @Cape lion
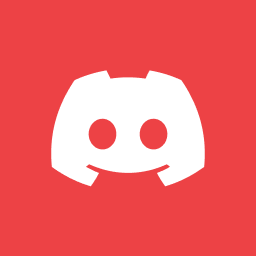
Cape lion
Happy to help you bro