Issue calling fetch and bringing it into a 'use client' component
Unanswered
Horned oak gall posted this in #help-forum
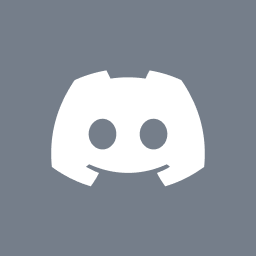
Horned oak gallOP
Having trouble with the correct way of doing this one... Basically I have a message panel that I first want to verify a user is logged in before fetching their messages to display to them.
First component to verify the user is authenticated to then pass their Id and token into the component which will retrieve their messages
Component which retrieves the messages then passes them to a client side component to render them
Then the component to render...(haven't fulled finished this yet since struggling to get the messages into it)
First component to verify the user is authenticated to then pass their Id and token into the component which will retrieve their messages
'use client'
import {useSession} from "next-auth/react";
import React from "react";
import MessageWindow from "@/components/MessageWindow";
export default function CheckUserIsLoggedIn() {
const {data: session, status} = useSession();
console.log(session?.user.identityId + 'first')
if (status === 'authenticated') {
return <MessageWindow identityId={session?.user.identityId} token={session?.user.backendToken}/>
}
return
}
Component which retrieves the messages then passes them to a client side component to render them
import React, {useEffect, useState} from "react";
import getAllMessagesForIdentity from "@/lib/getAllMessagesForIdentity";
import MessageWindowDetails from "@/components/MessageWindowDetails";
type Props = {
identityId: number | undefined,
token: string | undefined
}
export default async function MessageWindow({identityId, token}: Props) {
const messageData: Promise<Message[]> = await getAllMessagesForIdentity(identityId, token);
return (
<>
<MessageWindowDetails messages={messageData}/>
</>
)
}
Then the component to render...(haven't fulled finished this yet since struggling to get the messages into it)
"use client"
import {signOut, useSession} from "next-auth/react";
import React from "react";
import { useState, useEffect } from "react";
type Props = {
messages: Promise<Message[]>
}
export default function MessageWindowDetails({ messages }: Props) {
const {data: session, status} = useSession();
const [openCloseMessageWindow, setOpenCloseMessageWindow] = useState(false);
function openClose() {
if (!openCloseMessageWindow) {
setOpenCloseMessageWindow(true);
} else {
setOpenCloseMessageWindow(false);
}
}
if (status === 'authenticated') {
return (
<>
{openCloseMessageWindow && (
<div className="rounded-3xl border border-black p-8 w-80 h-96 bg-white fixed bottom-12 right-32">
<div>UL list mapped showing the conversations1</div>
</div>
)}
<button onClick={() => openClose()}
className="flex place-content-center items-center rounded-full border border-black p-8 w-8 h-8 hover:bg-rtOrange fixed bottom-12 right-12">
<svg xmlns="http://www.w3.org/2000/svg" className="absolute" width="24" height="24"
viewBox="0 0 24 24" fill="none" stroke="#000000" strokeWidth="2" strokeLinecap="round"
strokeLinejoin="round">
<path
d="M21 11.5a8.38 8.38 0 0 1-.9 3.8 8.5 8.5 0 0 1-7.6 4.7 8.38 8.38 0 0 1-3.8-.9L3 21l1.9-5.7a8.38 8.38 0 0 1-.9-3.8 8.5 8.5 0 0 1 4.7-7.6 8.38 8.38 0 0 1 3.8-.9h.5a8.48 8.48 0 0 1 8 8v.5z"></path>
</svg>
</button>
</>
)
}
}
4 Replies
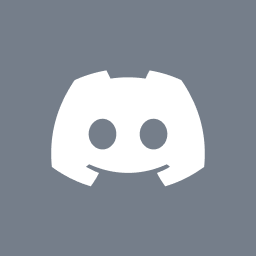
Horned oak gallOP
Also here is the fetch call
export default async function getAllMessagesForIdentity(identityId, token) {
const res = await fetch( `${process.env.NEXT_PUBLIC_HADES_URL}/message/identity/${identityId}`, {
method: "POST",
cache: "no-cache",
headers: {
"Content-Type": "application/json",
"Authorization": "Bearer " + token,
}
})
if (!res.ok) throw new Error('Failed to fetch messages')
return res.json()
}
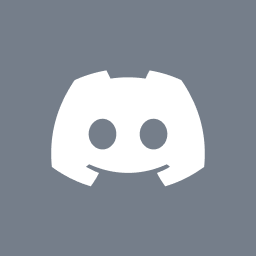
Horned oak gallOP
It would seem the problem is having a server component as a child of a client component... still working on the solution part.
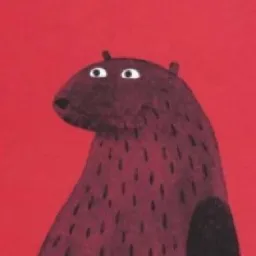
You cannot have a server component be a child of a client component. Consider your app structure like a tree, where the trunk and branches are server components, and the leaves are client components.
If you need things like context etc, you can have client component wrappers, but they need their children to be props that are passed through, like:
If you need things like context etc, you can have client component wrappers, but they need their children to be props that are passed through, like:
export function MyProvider({children}){
// blah blah all your context stuff
return <div>{children}</div>
}