cookies sat in the browser but not getting in the tsx file
Answered
Billy posted this in #help-forum
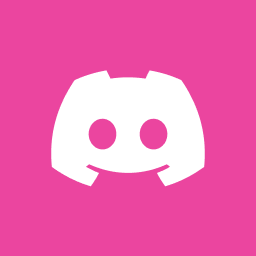
BillyOP
Hey guys, I'm working on a Nextjs project, and using
I have an endpoint request for getting all users and I get the
I tried to paste the token manually instead of the
NOTE: the token is shown in the browser
the
js-cookie
.I have an endpoint request for getting all users and I get the
Cookies.get("token")
from the browser saved cookies after setting it in login endpoint function.I tried to paste the token manually instead of the
${Cookies.get("token")}
it works perfectly with no issues.NOTE: the token is shown in the browser
the
action.tsx
import Cookies from "js-cookie";
import axios from "axios";
import { baseURL, endpoints, http } from "./endpoints";
export async function handleSignIn(
username: string,
password: string,
remeberMe: boolean
) {
// the backend request uses form method
const FD: FormData = new FormData();
FD.append("UserName", username);
FD.append("Password", password);
try {
const res = await http.post(endpoints.auth.signin, FD);
const token = await res.data.data.accessToken;
const refreshToken = await res.data.data.refreshToken;
if (token) {
Cookies.set("token", token, { path: "" });
console.log(Cookies.set("token", token, { path: "" }));
Cookies.set("refresh-token", JSON.stringify(refreshToken));
console.log("token saved");
}
console.log("successfully logined");
window.location.href = "/dashboard";
} catch (error) {
console.log(error);
}
}
export async function getAllUsers() {
try {
if (Cookies.get("token")) console.log("token found");
else console.log("not found");
const res = await axios.get(baseURL + endpoints.users.users, {
headers: {
Authorization: `Bearer ${Cookies.get("token")}`,
},
});
console.log("users", res);
return res;
} catch (error) {
console.log(error);
}
}

Answered by iyxan23
heya, you seem to confuse the boundaries between the server and client
in easier terms, you could say it's trying to get the "cookies of the server", but the fact is that the server doesn't store any cookies; the browser does, and it sends out the cookies in every requests it sends to the server.
in nextjs, the way how you could get the cookies of the requests that's coming in, is by using
js-cookie
is a library used to manage client-side cookies. its code is run on the browser to retrieve the cookies it had stored (unless for cookies that are HttpOnly
)action.ts
(using .tsx
for the action.ts
file is incorrect) is a file that defines server actions that run on the server. using js-cookie
here won't work because under the hood, it uses browser APIs to retrieve the cookiesin easier terms, you could say it's trying to get the "cookies of the server", but the fact is that the server doesn't store any cookies; the browser does, and it sends out the cookies in every requests it sends to the server.
in nextjs, the way how you could get the cookies of the requests that's coming in, is by using
cookies()
from next/headers
, see https://nextjs.org/docs/app/api-reference/functions/cookies4 Replies

heya, you seem to confuse the boundaries between the server and client
in easier terms, you could say it's trying to get the "cookies of the server", but the fact is that the server doesn't store any cookies; the browser does, and it sends out the cookies in every requests it sends to the server.
in nextjs, the way how you could get the cookies of the requests that's coming in, is by using
js-cookie
is a library used to manage client-side cookies. its code is run on the browser to retrieve the cookies it had stored (unless for cookies that are HttpOnly
)action.ts
(using .tsx
for the action.ts
file is incorrect) is a file that defines server actions that run on the server. using js-cookie
here won't work because under the hood, it uses browser APIs to retrieve the cookiesin easier terms, you could say it's trying to get the "cookies of the server", but the fact is that the server doesn't store any cookies; the browser does, and it sends out the cookies in every requests it sends to the server.
in nextjs, the way how you could get the cookies of the requests that's coming in, is by using
cookies()
from next/headers
, see https://nextjs.org/docs/app/api-reference/functions/cookiesAnswer

also, from a security perspective, i wouldn't suggest modifying or accessing the cookies from the client. it could be an opening for "hackers" to steal tokens from your users' browsers.
it's better to purely handle cookies in the server with
it's better to purely handle cookies in the server with
HttpOnly
cookies.here's a good article about cookies to learn more https://developer.mozilla.org/en-US/docs/Web/HTTP/Cookies
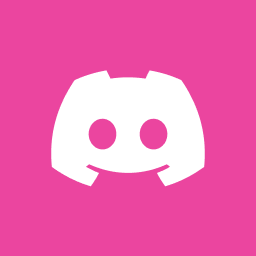
BillyOP
Hey man, thank you. I appreciate your effort in this long reply.