Trouble sending a form to the backend using the AI SDK libraries
Unanswered
Large garden bumble bee posted this in #help-forum
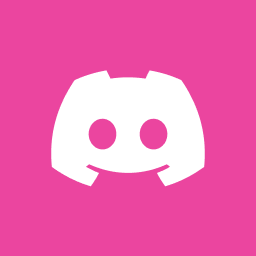
Large garden bumble beeOP
I have a form which is fairly simple and looks as follows:
I use this in one place like so, and it works fine:
However, I am trying to use this with the AI useChat and it's not working
Here's the problem: whenever I send to the backend, I can get one of multiple errors, the first being:
I've tried to set the
When I set it to
I've also tried passing through the
Strangely, I should add, manually passing an
Any ideas?
interface ChatFormProps extends ComponentProps<'form'> {
input?: string;
handleInputChange?: ChangeEventHandler<HTMLTextAreaElement>;
}
function ChatForm({
input,
handleInputChange,
...props
}: ComponentPropsWithRef<'form'>) {
const promptId = useId();
return (
<form {...props}>
<div role="group">
<label htmlFor={promptId}>Send Message</label>
<textarea id="prompt" name="prompt" value={input} onChange={handleInputChange} />
</div>
<button type="submit">Send</button>
</form>
);
}
I use this in one place like so, and it works fine:
function CreateChat() {
return (
<section className="flex flex-col">
<EmptyChatScreen />
<ChatForm method="POST" action="/api/v2/chat" />
</section>
);
}
However, I am trying to use this with the AI useChat and it's not working
export default function Chat({ chatId }) {
const csrfToken = useCsrfToken();
const { messages, handleSubmit, input, handleInputChange, isLoading } = useChat({
api: `/api/v2/chat/${chatId}/generate`,
headers: {
'X-CSRF-Token': csrfToken!,
},
});
return (
<section className="flex flex-col">
<ChatLog id={chatId} messages={messages} />
<ChatForm
onSubmit={handleSubmit}
aria-busy={isLoading}
input={input}
handleInputChange={handleInputChange}
/>
</section>
);
}
Here's the problem: whenever I send to the backend, I can get one of multiple errors, the first being:
TypeError: Content-Type was not one of "multipart/form-data" or "application/x-www-form-urlencoded".
I've tried to set the
'Content-Type'
in the header to application/x-www-form-urlencoded
, but when I inspect the form data, I don't have a prompt
availableWhen I set it to
multipart/form-data
, it gives me the error Failed to parse body as FormData.
export async function POST(request: NextRequest) {
const formData = await request.formData();
console.log(formData.get('prompt')!); // always null
return NextResponse.json();
}
I've also tried passing through the
input
and handleInputChange
to the ChatForm
using the handlers from useChat
, which also did not workStrangely, I should add, manually passing an
action
that makes a fetch request from the client does workAny ideas?