Local storage
Answered
Zepeto posted this in #help-forum
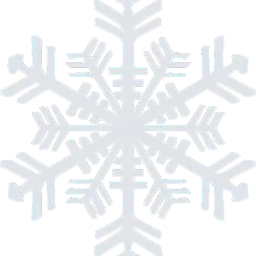
ZepetoOP
Hi, I'm trying to access the local storage in my helper.ts file, however I have this error :
How can I accomplish that ?
Error: localStorage is not defined
How can I accomplish that ?
Answered by B33fb0n3
no, because it needs the local storage. The server (server component) don't know any local storage. Because of that, it need to be importated like that and need to be clientside
29 Replies
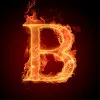
B33fb0n3
localStorage is only available in client component. You added
use client
on top?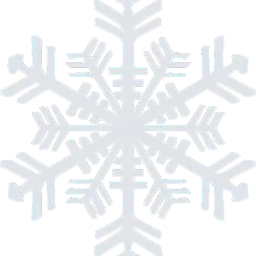
ZepetoOP
Yes but then I have an error when calling the function in my helper file
TypeError: (0 , _lib_helper__WEBPACK_IMPORTED_MODULE_3__.testFunction) is not a function
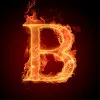
B33fb0n3
try to import the component that need the helper function like this:
const ComponentC = dynamic(() => import('path/to/Component'), { ssr: false })
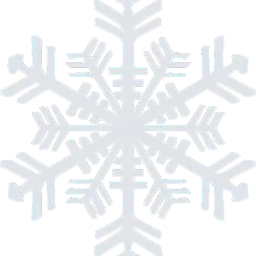
ZepetoOP
You mean I should import the helper file this way ?
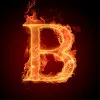
B33fb0n3
nah, only the component that uses your helper
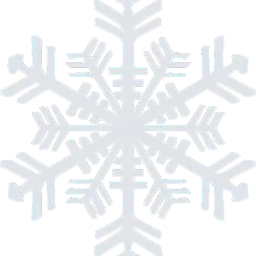
ZepetoOP
Oh okay, but I'm using the function in the server component of my page
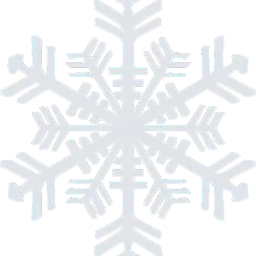
ZepetoOP
Should I call it in a client component ?
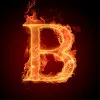
B33fb0n3
yea, localStorage is only available in client component.
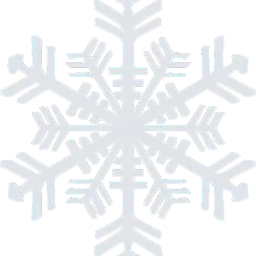
ZepetoOP
And then call my client component in my server one ?
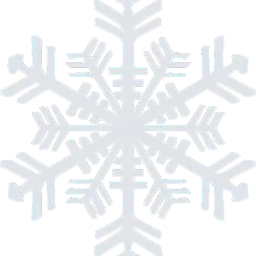
ZepetoOP
I created this function in a file
Then I call it in my helper file (which is not a client component)
But I still have this error
"use client";
export const getLocalStorage = (key: string) => {
return localStorage.getItem(key);
};
Then I call it in my helper file (which is not a client component)
export const testFunction = () => {
const test = getLocalStorage("test");
console.log(test);
};
But I still have this error
(0 , _client_helper__WEBPACK_IMPORTED_MODULE_2__.getLocalStorage) is not a function
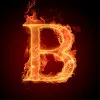
B33fb0n3
try to import the component that need the helper function like this:
const ComponentC = dynamic(() => import('path/to/Component'), { ssr: false })
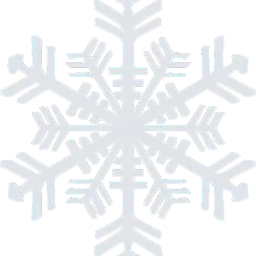
ZepetoOP
Promise<typeof import("...mypath")>' is not assignable to parameter of type 'DynamicOptions<{}> | Loader<{}>'.
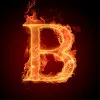
B33fb0n3
which one did you try now?
testFunction
or getLocalStorage
?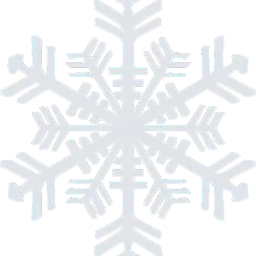
ZepetoOP
importing getLocalStorage in the helper file (which contain the testFunction)
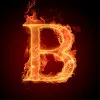
B33fb0n3
can you give me an reproduction repo? For example through jsfiddle or https://codesandbox.io/ ?
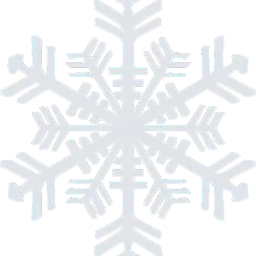
ZepetoOP
Sure let me 15min
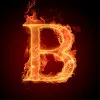
B33fb0n3
I modified your repo a bit to make it work: https://codesandbox.io/p/devbox/vibrant-wilbur-nd5fg5
You can see, that you need to import the Component that uses the helper function without SSR. After that, there are no more errors
You can see, that you need to import the Component that uses the helper function without SSR. After that, there are no more errors
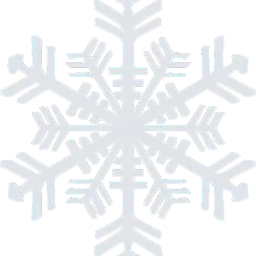
ZepetoOP
I still see the errors
weird
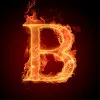
B33fb0n3
Whoops, I shared the wrong link. This is the right one: https://codesandbox.io/p/devbox/epic-panka-xykssv
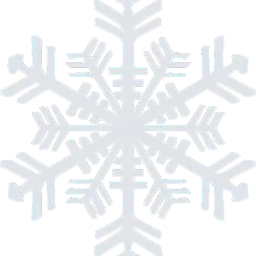
ZepetoOP
My page.tsx is a server component 🥲
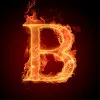
B33fb0n3
I changed the code, so page.tsx is not a client component anymore
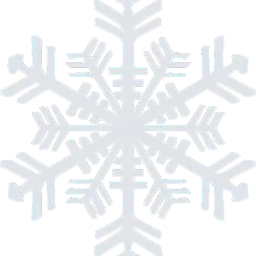
ZepetoOP
Thanks, can SomeComponent.tsx be a server component too ?
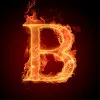
B33fb0n3
no, because it needs the local storage. The server (server component) don't know any local storage. Because of that, it need to be importated like that and need to be clientside
Answer
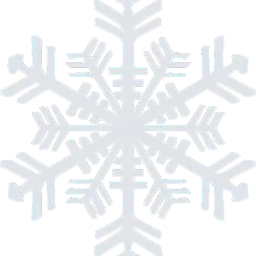
ZepetoOP
Ok, So I need to create a client component and then inside of it I have to call my function then ?
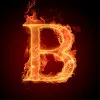
B33fb0n3
yes
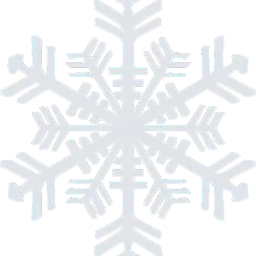
ZepetoOP
okay thanks 🙂
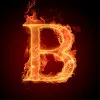
B33fb0n3
sure thing