can't resolve 'net'
Answered
West Siberian Laika posted this in #help-forum
West Siberian LaikaOP
I made this simple button with the only purpose of deleting a product, but it breaks my project whenever I import it to my admin page.
this error seems to be related to my
All related code (I use app router):
"use client";
I call the button in this component:
this error seems to be related to my
postgres
package which I use to interact with my supabase db.All related code (I use app router):
src/components/ui/dashboard/delete-button.tsx
"use client";
import { deleteProduct } from "@/lib/actions";
import { Button } from "../button";
import { useState } from "react";
export const DeleteProduct = (props: any) => {
const [error, setError] = useState(null);
const id = props.id;
async function handleDelete(e: any) {
const result = await deleteProduct(id);
if (!result) {
setError(e.error);
}
}
return <Button onClick={() => handleDelete} />;
};
src/lib/actions.ts
import { revalidatePath } from "next/cache";
import sql from "../../db";
export async function deleteProduct(id: any) {
try {
const product = await sql`
delete from products
where id = ${id};
`;
revalidatePath("/admin");
return product;
} catch (e) {
console.log("Error deleting product: ", e);
throw e;
}
}
I call the button in this component:
src/app/admin/page.tsx
import { DeleteProduct } from "@/components/ui/dashboard/delete-button";
import { Products } from "@/components/ui/dashboard/products";
import { getProducts } from "@/lib/actions";
export default async function Page() {
const products = await getProducts(100);
return (
{products?.map((product) => (
<>
<Products
title={product.title}
imageurl={product.imageurl}
price={1000}
/>
<DeleteProduct id={product.id} />
</>
))}
);
}
15 Replies
@joulev Add "use server" at the top of the actions.ts file
West Siberian LaikaOP
Wow, I searched many forums but no one suggested that, what was the cause of this? I thought Nextjs components are server components by default.
Server components arent the same as server actions.
@West Siberian Laika Wow, I searched many forums but no one suggested that, what was the cause of this? I thought Nextjs components are server components by default.
delete-button is a client component, so you were asking your app to run the sql query on the browser which obviously doesn’t work
West Siberian LaikaOP
Thank you so much guys @joulev @Arinji I was also gonna ask you why my button wasn't working but I realized I wasn't calling the function properly lol
No worries, mark a solution :D
Original message was deleted
boop
West Siberian LaikaOP
ofc, I'll also give it a better name
Giant panda
import { deleteProduct } from "@/lib/actions";
import { Button } from "../button";
import { useState } from "react";
export const DeleteProduct = (props: any) => {
const [error, setError] = useState(null);
const id = props.id;
async function handleDelete(e: any) {
const result = await deleteProduct(id);
if (!result) {
setError(e.error);
}
}
return <Button onClick={() => handleDelete} />;
};
why you are passing the function like that when it doesn't have params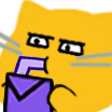
@Giant panda js
import { deleteProduct } from "@/lib/actions";
import { Button } from "../button";
import { useState } from "react";
export const DeleteProduct = (props: any) => {
const [error, setError] = useState(null);
const id = props.id;
async function handleDelete(e: any) {
const result = await deleteProduct(id);
if (!result) {
setError(e.error);
}
}
return <Button onClick={() => handleDelete} />;
}; why you are passing the function like that when it doesn't have params
West Siberian LaikaOP
My coding philosophy, write first, think later lol
"use client";
import { deleteProduct } from "@/lib/actions";
import { Button } from "../button";
import { useState } from "react";
export default function DeleteProduct(props: any) {
const [error, setError] = useState(null);
const id = props.id;
async function handleDelete(e: any) {
const result = await deleteProduct(id);
if (!result) {
setError(e.error);
}
}
return (
<Button variant={"destructive"} onClick={() => handleDelete(id)}>
Delete
</Button>
);
}
just added parenthesis to click event
It works so I probably won't touch it for the next 10 light years
Giant panda
great