use server action to post data to route handler
Answered
Elite posted this in #help-forum
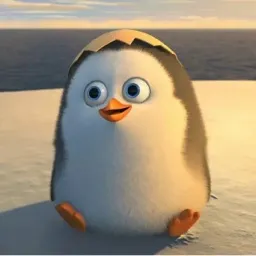
EliteOP
trying to use server action to post data to route handler
code:
code:
(page.tsx)
import ExploreTable from './explore-table';
import { auth } from '@clerk/nextjs';
export const metadata = {
title: "PartnerFind | Explore"
};
export default async function ExplorePage() {
async function fetchAllTableData(userId: string) { // fetch all the partners as well as user specific partners list
'use server'
try {
const options = {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ userID: userId }), // Pass the user ID to the backend
};
const getAllRows = await fetch('/api/db/fetch-all-rows-with-user', options); // TODO
if (getAllRows.ok) {
const data = await getAllRows.json();
return data
} else {
throw new Error("Error fetching rows from DB.");
}
} catch (error) {
console.error("An error occurred while fetching from the DB:", error);
throw new Error("An error occurred while fetching from the DB:");
}
}
const { userId } : { userId: string | null } = auth(); //get clerk user ID
let data: string | null = null;
if (userId !== null) {
data = await fetchAllTableData(userId);
}
return (
<>
<ExploreTable data={data || ''} />
</>
);
}
Answered by !=tgt
you can access server side stuff in a server component like what you have there
35 Replies

!=tgt
you can access server side stuff in a server component like what you have there
Answer

!=tgt
you don't need a route handler
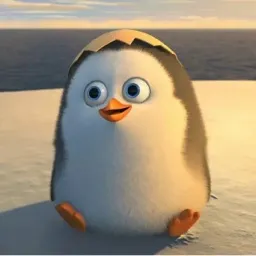
EliteOP
so just do my api calls directly. and not fetch my route handlers?
is it a waste to even fetch from my route handlers?

!=tgt
yeah it is
just directly do the call
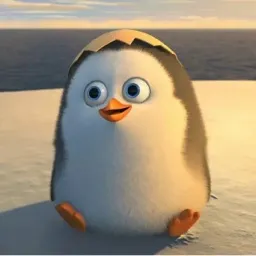
EliteOP
ah i see. so jsut like in my lib folder

!=tgt
yep
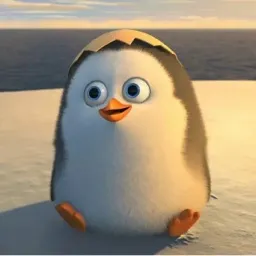
EliteOP
quick question:
if all my page.tsx are server components,
then theres no point to having route handlers then right?
cause all the logic can happen in the
unless I have a client page.tsx
then I can do a fetch request from the client side if I want. or a server action running on the server
right?
if all my page.tsx are server components,
then theres no point to having route handlers then right?
cause all the logic can happen in the
lib
or util
folders right?unless I have a client page.tsx
then I can do a fetch request from the client side if I want. or a server action running on the server
right?

!=tgt
yeah
there's no point unless it's client, and even then, you could use a server action
there's no point unless it's client, and even then, you could use a server action
I use a mix
like i have a setInterval where i send an image to the server, thats a handler
like i have a setInterval where i send an image to the server, thats a handler
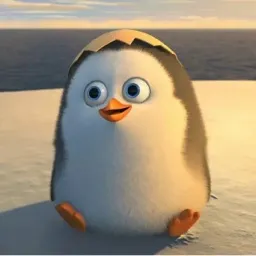
EliteOP
So route handlers are pretty much useless? Unless I want designated routes forever
So I want to in the future make my server side logic public to users. Like an api for them to use.
How would I build that with nextjs natively?
Like if I don’t have any route handlers. And all my logic is above the return method of a page.tsx(because all of my pages are server components with child components that may be client)
How would I build that with nextjs natively?
Like if I don’t have any route handlers. And all my logic is above the return method of a page.tsx(because all of my pages are server components with child components that may be client)

!=tgt
Route handlers still have a use
Like I have an application that uploads an image to a DB every 10 seconds, triggered by a useEffect
and a route handler is much more flexible in that case
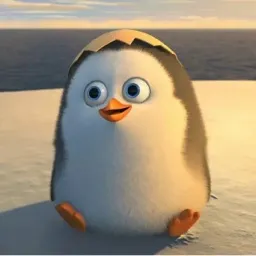
EliteOP
Oh ye but if it’s a server component I don’t need to use route handlers at all?

!=tgt
nope
in a server component
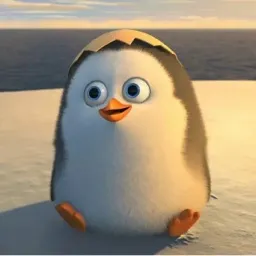
EliteOP
Like I’d only use route handlers for certain cases on the client side.
Cause all of my pages are server
Cause all of my pages are server

!=tgt
you can directly query a db for example
yeah
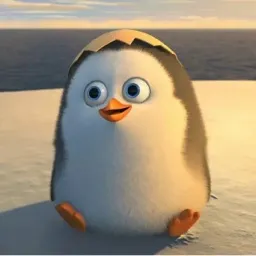
EliteOP
Ye ok but what if I wanted to make a public api that users can use that has this logic.
I dont want the same code in 2 places (in my server component and a protected by auth route handler)
Cause I can’t call route handlers from server components
I dont want the same code in 2 places (in my server component and a protected by auth route handler)
Cause I can’t call route handlers from server components
Do I just put the logic I want to be shared in a util or lib folder?
And then import that in my logic for a server component.
If it’s reusable (both server side and for the api route a user can access), do I just import the function to a route handler?
And then import that in my logic for a server component.
If it’s reusable (both server side and for the api route a user can access), do I just import the function to a route handler?
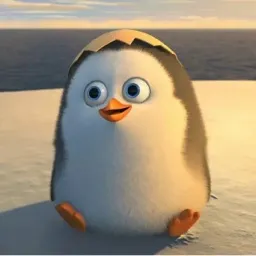
EliteOP
Hey sorry for the ping Dyk what I could do

!=tgt
Yeah you can put it like that
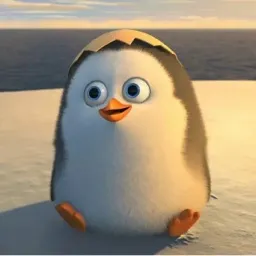
EliteOP
Alr. So just to clarify if I want logic to be reusable for the public and used in my code, I put the code in /util. And import and return that in an api route. But in my code like the pages, I just import the util function and not the api route correct?

!=tgt
yep
pretty much
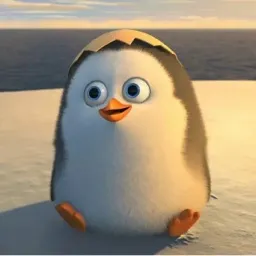
EliteOP
Bet
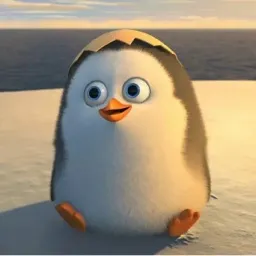
EliteOP
yo so for error handling what should i do if I have my logic in like a util/logicHere.ts?
like heres an example of my old code:
so like instead of returning a nextresponse with a 500 status code, waht should i do?
like heres an example of my old code:
let userList = null;
try {
userList = await db.select().from(userFavorites).where(s`${userFavorites.userID} = ${userID}`);
} catch (err: any) {
console.error(err)
return NextResponse.json(
{ error: `Failed to query userFavorites | ${err.message}` },
{ status: 500 }
)
}
so like instead of returning a nextresponse with a 500 status code, waht should i do?

!=tgt
for me I just throw an error
if it fails
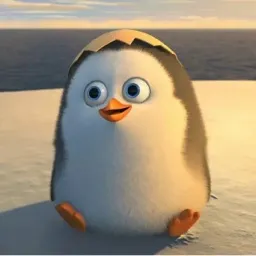
EliteOP
alr and then can i access the thrown error's message?

!=tgt
yeah