NextJS / Supabase App Project
Unanswered
Transvaal lion posted this in #help-forum
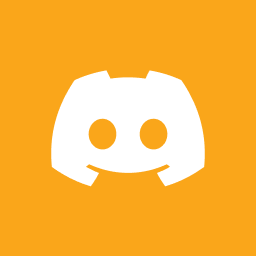
Transvaal lionOP
Hey All,
I am a bit of a nextJS noobie, but I am attempting to make a full stack web app with nextJS and Supabase. I would ideally like to make an app that lets a user sign in to a dashboard full of data, and if there is no data there, then have a form prompt them to fill in data. I currently have a starter pack set up with Auth, and a protected landing page. I have attached my code in this as a zip file. Would love to chat with someone for like 30 minutes to figure out how i can get going on this project.
I am a bit of a nextJS noobie, but I am attempting to make a full stack web app with nextJS and Supabase. I would ideally like to make an app that lets a user sign in to a dashboard full of data, and if there is no data there, then have a form prompt them to fill in data. I currently have a starter pack set up with Auth, and a protected landing page. I have attached my code in this as a zip file. Would love to chat with someone for like 30 minutes to figure out how i can get going on this project.
4 Replies
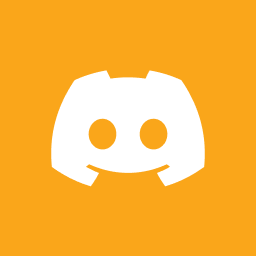
Transvaal lionOP
the basic gist of my question is how can I effectively make a system that logs in a user(done), then lands them on a dashboard style page populated with their data(from supabase). If no data is there, prompt them to fill out a form or something.
Can show code if necessary!
Can show code if necessary!
// This page should load data from the database and display it in a table, it is for logged in users only
// the data should be loaded from the table "restaurants" and "menus"
// the schema for the tables are as follows:
// [
// {
// "id": "8ba93cb3-51a3-4ff2-9ed8-d3c094065623",
// "restaurant_id": null,
// "title": "Testing menu",
// "description": null,
// "created_at": "2024-04-21 03:32:55+00",
// "video_url": null,
// "metadata": null
// }
// ]
// [
// {
// "id": "8ba93cb3-51a3-4ff2-9ed8-d3c094065623",
// "restaurant_id": null,
// "title": "Testing menu",
// "description": null,
// "created_at": "2024-04-21 03:32:55+00",
// "video_url": null,
// "metadata": null
// }
// ]
// incorporate a request to the database to get the data
// add a table to the page that displays the data
// add a button to the page that allows the user to add a new restaurant, if there is no data in the table, it should display a form to add a new restaurant
import { createClient } from "@/utils/supabase/server";
import { redirect } from "next/navigation";
import AuthButton from "../../components/AuthButton";
export default async function ProtectedPage() {
const supabase = createClient();
const {
data: { user },
} = await supabase.auth.getUser();
if (!user) {
return redirect("/login");
}
return (
<div className="flex-1 w-full flex flex-col gap-20 items-center">
<div className="w-full">
<div className="py-6 font-bold bg-purple-950 text-center">
This is a protected page that you can only see as an authenticated
user
{supabase && <AuthButton />}
</div>
<nav className="w-full flex justify-center border-b border-b-foreground/10 h-16">
</nav>
</div>
</div>
);
}
// the data should be loaded from the table "restaurants" and "menus"
// the schema for the tables are as follows:
// [
// {
// "id": "8ba93cb3-51a3-4ff2-9ed8-d3c094065623",
// "restaurant_id": null,
// "title": "Testing menu",
// "description": null,
// "created_at": "2024-04-21 03:32:55+00",
// "video_url": null,
// "metadata": null
// }
// ]
// [
// {
// "id": "8ba93cb3-51a3-4ff2-9ed8-d3c094065623",
// "restaurant_id": null,
// "title": "Testing menu",
// "description": null,
// "created_at": "2024-04-21 03:32:55+00",
// "video_url": null,
// "metadata": null
// }
// ]
// incorporate a request to the database to get the data
// add a table to the page that displays the data
// add a button to the page that allows the user to add a new restaurant, if there is no data in the table, it should display a form to add a new restaurant
import { createClient } from "@/utils/supabase/server";
import { redirect } from "next/navigation";
import AuthButton from "../../components/AuthButton";
export default async function ProtectedPage() {
const supabase = createClient();
const {
data: { user },
} = await supabase.auth.getUser();
if (!user) {
return redirect("/login");
}
return (
<div className="flex-1 w-full flex flex-col gap-20 items-center">
<div className="w-full">
<div className="py-6 font-bold bg-purple-950 text-center">
This is a protected page that you can only see as an authenticated
user
{supabase && <AuthButton />}
</div>
<nav className="w-full flex justify-center border-b border-b-foreground/10 h-16">
</nav>
</div>
</div>
);
}
// This page should load data from the database and display it in a table, it is for logged in users only
// the data should be loaded from the table "restaurants" and "menus"
// the schema for the tables are as follows:
// [
// {
// "id": "8ba93cb3-51a3-4ff2-9ed8-d3c094065623",
// "restaurant_id": null,
// "title": "Testing menu",
// "description": null,
// "created_at": "2024-04-21 03:32:55+00",
// "video_url": null,
// "metadata": null
// }
// ]
// [
// {
// "id": "8ba93cb3-51a3-4ff2-9ed8-d3c094065623",
// "restaurant_id": null,
// "title": "Testing menu",
// "description": null,
// "created_at": "2024-04-21 03:32:55+00",
// "video_url": null,
// "metadata": null
// }
// ]
// incorporate a request to the database to get the data
// add a table to the page that displays the data
// add a button to the page that allows the user to add a new restaurant, if there is no data in the table, it should display a form to add a new restaurant
import { createClient } from "@/utils/supabase/server";
import { redirect } from "next/navigation";
import AuthButton from "../../components/AuthButton";
export default async function ProtectedPage() {
const supabase = createClient();
const {
data: { user },
} = await supabase.auth.getUser();
if (!user) {
return redirect("/login");
}
return (
<div className="flex-1 w-full flex flex-col gap-20 items-center">
<div className="w-full">
<div className="py-6 font-bold bg-purple-950 text-center">
This is a protected page that you can only see as an authenticated
user
{supabase && <AuthButton />}
</div>
<nav className="w-full flex justify-center border-b border-b-foreground/10 h-16">
</nav>
</div>
</div>
);
}
// the data should be loaded from the table "restaurants" and "menus"
// the schema for the tables are as follows:
// [
// {
// "id": "8ba93cb3-51a3-4ff2-9ed8-d3c094065623",
// "restaurant_id": null,
// "title": "Testing menu",
// "description": null,
// "created_at": "2024-04-21 03:32:55+00",
// "video_url": null,
// "metadata": null
// }
// ]
// [
// {
// "id": "8ba93cb3-51a3-4ff2-9ed8-d3c094065623",
// "restaurant_id": null,
// "title": "Testing menu",
// "description": null,
// "created_at": "2024-04-21 03:32:55+00",
// "video_url": null,
// "metadata": null
// }
// ]
// incorporate a request to the database to get the data
// add a table to the page that displays the data
// add a button to the page that allows the user to add a new restaurant, if there is no data in the table, it should display a form to add a new restaurant
import { createClient } from "@/utils/supabase/server";
import { redirect } from "next/navigation";
import AuthButton from "../../components/AuthButton";
export default async function ProtectedPage() {
const supabase = createClient();
const {
data: { user },
} = await supabase.auth.getUser();
if (!user) {
return redirect("/login");
}
return (
<div className="flex-1 w-full flex flex-col gap-20 items-center">
<div className="w-full">
<div className="py-6 font-bold bg-purple-950 text-center">
This is a protected page that you can only see as an authenticated
user
{supabase && <AuthButton />}
</div>
<nav className="w-full flex justify-center border-b border-b-foreground/10 h-16">
</nav>
</div>
</div>
);
}
// This page should load data from the database and display it in a table, it is for logged in users only
// the data should be loaded from the table "restaurants" and "menus"
// the schema for the tables are as follows:
// [
// {
// "id": "8ba93cb3-51a3-4ff2-9ed8-d3c094065623",
// "restaurant_id": null,
// "title": "Testing menu",
// "description": null,
// "created_at": "2024-04-21 03:32:55+00",
// "video_url": null,
// "metadata": null
// }
// ]
// [
// {
// "id": "8ba93cb3-51a3-4ff2-9ed8-d3c094065623",
// "restaurant_id": null,
// "title": "Testing menu",
// "description": null,
// "created_at": "2024-04-21 03:32:55+00",
// "video_url": null,
// "metadata": null
// }
// ]
// incorporate a request to the database to get the data
// add a table to the page that displays the data
// add a button to the page that allows the user to add a new restaurant, if there is no data in the table, it should display a form to add a new restaurant
import { createClient } from "@/utils/supabase/server";
import { redirect } from "next/navigation";
import AuthButton from "../../components/AuthButton";
export default async function ProtectedPage() {
const supabase = createClient();
const {
data: { user },
} = await supabase.auth.getUser();
if (!user) {
return redirect("/login");
}
return (
<div className="flex-1 w-full flex flex-col gap-20 items-center">
<div className="w-full">
<div className="py-6 font-bold bg-purple-950 text-center">
This is a protected page that you can only see as an authenticated
user
{supabase && <AuthButton />}
</div>
<nav className="w-full flex justify-center border-b border-b-foreground/10 h-16">
</nav>
</div>
</div>
);
}