Middleware and cached Cookies
Unanswered
Phainopepla posted this in #help-forum
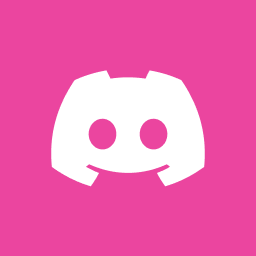
PhainopeplaOP
Im trying to set protected routes and redirections. I saw that there is a lot of similar issues here and I don't figure this out yet. Im just trying to read a cookie on the middleware for each request to determine if I have to redirect user or not. On dev mode is all good, even on build and start locally. But on server hosted build is not working properly.
Here is a bit of my code:
BTW, I have some links with prefetch to make the page faster
Here is a bit of my code:
import { type NextRequest, userAgent, NextResponse } from 'next/server';
import { type SellerDocument } from './const/types';
export function middleware(request: NextRequest) {
try {
const { isBot } = userAgent(request);
const currentUser: SellerDocument | undefined = request.cookies.get(
'loggedUser'
)?.value
? (JSON.parse(request.cookies.get('loggedUser')!.value) as SellerDocument)
: undefined;
const redirectCode = isBot ? 308 : 307;
console.log(
'Test middleware (No cache - Redirect): ',
currentUser?.verifiedLevel,
redirectCode
);
if (
currentUser &&
(request.nextUrl.pathname.startsWith('/login') ||
request.nextUrl.pathname.startsWith('/register'))
) {
console.log('Redirecting to dashboard');
const redirectResponse = NextResponse.redirect(
new URL('/dashboard', request.url),
redirectCode
);
return redirectResponse;
}
if (currentUser && request.nextUrl.pathname.startsWith('/sellers')) {
console.log('Redirecting to home');
const redirectResponse = NextResponse.redirect(
new URL('/', request.url),
redirectCode
);
return redirectResponse;
}
return NextResponse.next();
} catch (error) {
console.error('Middleware error: ', error);
return NextResponse.next();
}
}
export const config = {
matcher: ['/((?!api|_next/static|_next/image|favicon.ico).*)']
};
BTW, I have some links with prefetch to make the page faster
9 Replies
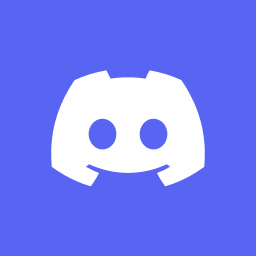
Asian black bear
@Phainopepla I think maybe you need to do some basic debugging here. Are there any errors/messages in the Vercel logs for the middleware? Does the
console.error
ever fire, or the messages saying a redirect is being performed?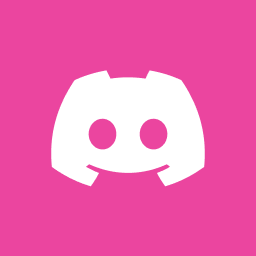
PhainopeplaOP
Im not using vercel hosting, im using Firebase Hosting with Web frameworks option. On the Cloud run I dont get any middleware errors
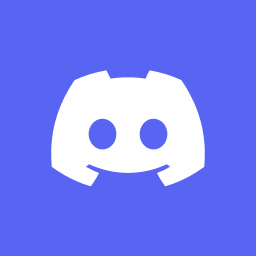
Ancient Murrelet
@Phainopepla do you have any progress I have the same issues
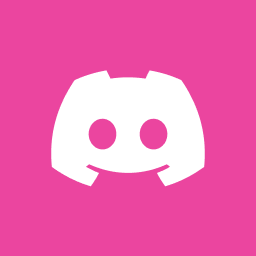
PhainopeplaOP
@Ancient Murrelet Unfortunately not, managed routing on pages even though is not the best approach. Was going to research more in deep in the following days but if you get it to properly work please let me know
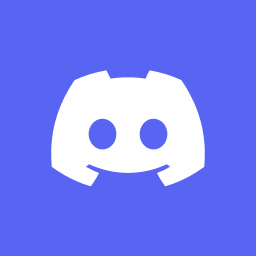
Ancient Murrelet
I’ve come to a solution and written a blog post about it:
https://www.julianmwagner.com/articles/nextjs-secure-seamless-private-data
https://www.julianmwagner.com/articles/nextjs-secure-seamless-private-data
Does your implementation work if you access it directly? not through a <Link> component?
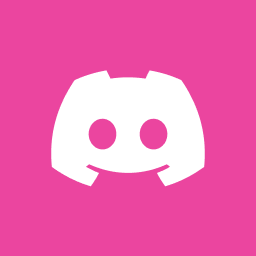
PhainopeplaOP
@Ancient Murrelet yes cause I do it on component mount like checking auth state and do a router push. But is not the best practice. The best case scenario would be that the middleware say when to route user to other route with a redirection if there is no cookie for example. But the issue is that the cookies are not being read on each navigation properly and prefetch also struggles for the reason I said before. Like you are un dashboard and prefetch other sections that with no cookie they return a redirect and you get redirected.
@Ancient Murrelet just read your blog, didn't saw the skip Middleware config option before, will give it a try. You should also try an test out with the matcher on the Middleware
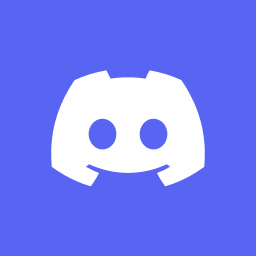
Ancient Murrelet
Yeah i think you have a similar problem that I had. Now it works really smoothly for us. Let me know how it goes. Yes you are right I could solve it with the matcher as well, but I didn’t have the patience to build the right regex for it