Invalid <Link> with <a> child. Please remove <a> or use <Link legacyBehavior>
Answered
White-tailed Hawk posted this in #help-forum
White-tailed HawkOP
Found some old project, decided to update, and an error appears on the / page; Solutions on the official resource didn't help
Help me understand what the problem is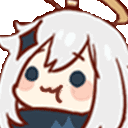
import { IconName } from '@fortawesome/fontawesome-svg-core'
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome'
import { GenericMeta } from 'components/GenericMeta'
import { NowPlayingCard } from 'components/NowPlayingCard'
import { Weather } from 'components/Weather'
import { Account, AccountData } from 'data/accounts'
import { MainLayout } from 'layouts/MainLayout'
import { useTheme } from 'next-themes'
import dynamic from 'next/dynamic'
import toast from 'react-hot-toast'
import { v4 as uuidv4 } from 'uuid'
const Time = dynamic(() => import('components/Time'), {
ssr: false,
})
const SocialLink = ({ name, href, icon, copyEmail }: Account) => {
return (
<a
aria-label={name}
onClick={() => name === 'Email' && copyEmail()}
className="cursor-pointer fill-current focus:outline-none transition duration-300 ease-in-out hover:text-indigo-900 dark:hover:text-indigo-200"
href={href}
rel="noopener noreferrer"
target="_blank"
>
<FontAwesomeIcon size="1x" icon={icon ? icon : ['fab', name.toLowerCase() as IconName]} />
</a>
)
}
const Home = () => {
const { theme } = useTheme()
const copyEmail = () => {
navigator.clipboard.writeText('email@gmail.com')
theme === 'dark'
? toast.success('Copied email to clipboard!', {
style: {
background: '#333',
color: '#fff',
},
})
: toast.success('Copied email to clipboard!')
}
return (
<>
<GenericMeta
title="title"
description="description"
/>
<MainLayout margin={false}>
<h1 className="text-6xl font-bold">title</h1>
<p className="text-lg text-gray-600 dark:text-gray-400 max-w-sm mt-2">
text <span className="font-semibold">text-semibold</span> text.
</p>
<div className="grid grid-flow-col w-48 mt-3 text-lg">
{AccountData.map((account) => (
<SocialLink
key={uuidv4()}
name={account.name}
href={account.href}
icon={account.icon}
copyEmail={copyEmail}
/>
))}
</div>
<div className="grid my-8 gap-2">
<Time />
<Weather />
</div>
<div>
<NowPlayingCard />
</div>
</MainLayout>
</>
)
}
export default Home
⨯ node_modules\next\dist\client\link.js (274:22) @ LinkComponent
⨯ Error: Invalid <Link> with <a> child. Please remove <a> or use <Link legacyBehavior>.
Learn more: https://nextjs.org/docs/messages/invalid-new-link-with-extra-anchor
at LinkComponent (webpack-internal:///./node_modules/next/dist/client/link.js:269:23)
page: '/'
null
Help me understand what the problem is
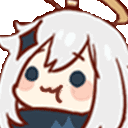
Answered by not-milo.tsx
Either do this:
or this:
<Link href="">Your link label</Link>
or this:
<Link href="" legacyBehaviour>
<a>Your link label</a>
</Link>
2 Replies
The problem is perfectly described in the error message. You can't have an
a
tag inside a Link
component cause it already renders one. It used to be that you had to do that, but since Next.js v13 that behaviour changed and if you want to preserve it you need to pass the legacyBehaviour
prop to the Link
Either do this:
or this:
<Link href="">Your link label</Link>
or this:
<Link href="" legacyBehaviour>
<a>Your link label</a>
</Link>
Answer