Working with Context API, I need to pass props to child component?
Unanswered
demonslayer posted this in #help-forum
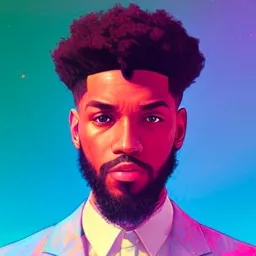
demonslayerOP
So Im working with context API, created a theme provider and wrapped it around children in my layout component. The goal is to try to avoid "use client" to fully utilize the power of SSR. How do I pass my context API prop into all my child component without having to convert them?
I want to pass the currentTheme var from context API without using "use client"
// /app/layout.tsx //This is the root layout
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
if (!process.env.NEXT_PUBLIC_GA_MEASUREMENT_ID) return null;
const measurementId = process.env.NEXT_PUBLIC_GA_MEASUREMENT_ID;
return (
<html lang="en">
<ThemeProvider>
<body className={roboto.className}>
<Header />
{children}
<Footer />
<GoogleAnalytics gaId={measurementId} />
</body>
</ThemeProvider>
</html>
);
}
// /app/page.tsx
// Home Page - Main Page of the Application
// Sections Components
import HeroSection from "@/components/containers/Hero";
import AboutSection from "@/components/containers/About";
const Home = () => {
return (
<main className="h-full">
{/* Home Page Sections */}
<HeroSection />
<AboutSection />
... rest of the code
);
};
export default Home;
I want to pass the currentTheme var from context API without using "use client"
// /components/ServicesSection.tsx - component
import { listOfServices } from "@/constants";
import SectionTitle from "@/components/ui/SectionTitle";
import ServiceCard from "@/components/ui/Cards/Service";
const ServicesSection = () => {
return (
<section
className="h-auto bg-[--color-dark-gray] text-white flex flex-col p-10"
id="services"
>
... rest of the code
</section>
);
};
export default ServicesSection;
2 Replies
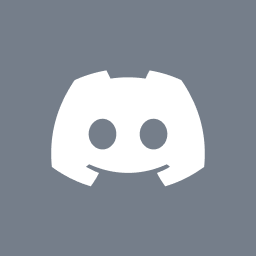
English Angora
You would have to make the services section a client component. But don’t worry just because it’s a client component doesn’t mean it’s not being rendered in the server. Ideally you make the client component as deep down in the render tree as possible
In this case since you are using a theme which is dependent on context it may not be able to render in the server as the results of the theme will be different depending on if you’re on the server or the client. Due to this you should use an effect to wait for the component to mount and once it’s mounted render the component based on the theme