Posts rendering on dev build but not on vercel app
Answered
Pavement ant posted this in #help-forum
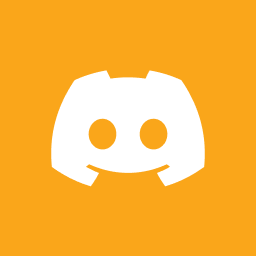
Pavement antOP
Hi, Ive recently made a app which uses PostgreSQL that i've recently launched on vercel, However when I use the
npm run build
version of the app, the posts map normally as they should, through whatever is in the db, however in the launched vercel app they wont load at all, The build had no errors, and works perfectly fine on the dev buildAnswered by Hong
Try this:
import { PrismaClient } from "@prisma/client";
import { Posts } from "./Posts";
import { unstable_noStore as noStore } from 'next/cache' // <-- add this
const prisma: PrismaClient = new PrismaClient();
async function getPosts() {
noStore(); // <-- add this
const posts = await prisma.post.findMany({
where: { published: true },
include: {
author: {
select: { name: true },
},
},
});
return posts;
}
export async function Feed() {
const posts = await getPosts();
return (
<div>
<div className="mt-5 flex flex-col w-full ">
{posts.map((post) => (
<div
key={post.id}
className="mt-5 p-5 outline outline-1 shadow-md shadow-slate-400 outline-gray-400 rounded-lg"
>
<Posts
id={post.id}
title={post.title}
content={post.content}
authorName={post.author?.name}
/>
</div>
))}
</div>
</div>
);
}
9 Replies
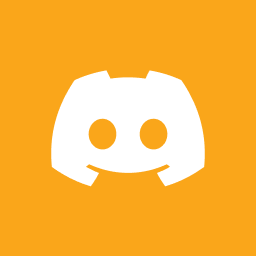
Pavement antOP
For reference, this is the Vercel app

This is the dev build

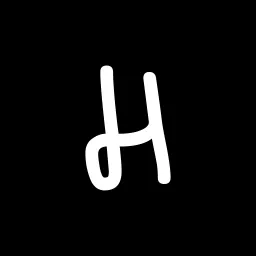
@Pavement ant Can you provide some code? How to do you fetch the data?
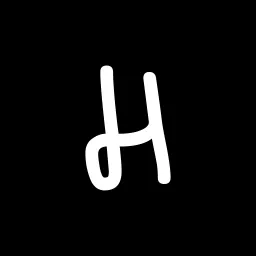
@Hong <@315879407908814848> Can you provide some code? How to do you fetch the data?
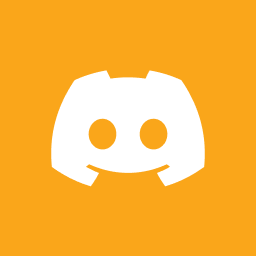
Pavement antOP
// This is in Components/Feed.tsx
import { PrismaClient } from "@prisma/client";
import { Posts } from "./Posts";
const prisma: PrismaClient = new PrismaClient();
async function getPosts() {
const posts = await prisma.post.findMany({
where: { published: true },
include: {
author: {
select: { name: true },
},
},
});
return posts;
}
export async function Feed() {
const posts = await getPosts();
return (
<div>
<div className="mt-5 flex flex-col w-full ">
{posts.map((post) => (
<div
key={post.id}
className="mt-5 p-5 outline outline-1 shadow-md shadow-slate-400 outline-gray-400 rounded-lg"
>
<Posts
id={post.id}
title={post.title}
content={post.content}
authorName={post.author?.name}
/>
</div>
))}
</div>
</div>
);
}
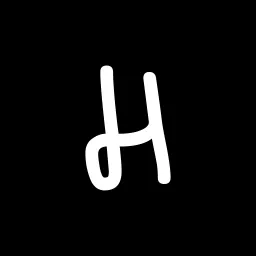
Try this:
import { PrismaClient } from "@prisma/client";
import { Posts } from "./Posts";
import { unstable_noStore as noStore } from 'next/cache' // <-- add this
const prisma: PrismaClient = new PrismaClient();
async function getPosts() {
noStore(); // <-- add this
const posts = await prisma.post.findMany({
where: { published: true },
include: {
author: {
select: { name: true },
},
},
});
return posts;
}
export async function Feed() {
const posts = await getPosts();
return (
<div>
<div className="mt-5 flex flex-col w-full ">
{posts.map((post) => (
<div
key={post.id}
className="mt-5 p-5 outline outline-1 shadow-md shadow-slate-400 outline-gray-400 rounded-lg"
>
<Posts
id={post.id}
title={post.title}
content={post.content}
authorName={post.author?.name}
/>
</div>
))}
</div>
</div>
);
}
Answer
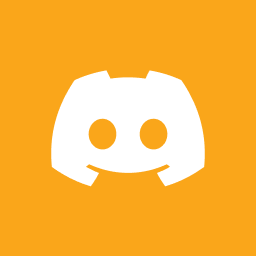
Pavement antOP
This worked thanks for your help!
Can I get a little lecture as to what noStore does because I dont really understand it from what i've googled 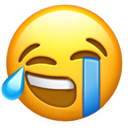
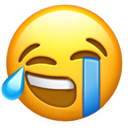
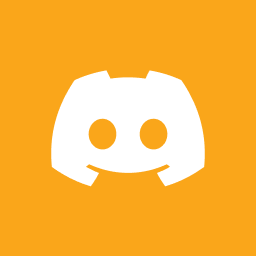
@Pavement ant Can I get a little lecture as to what noStore does because I dont really understand it from what i've googled <:lolsob:753870958489632819>
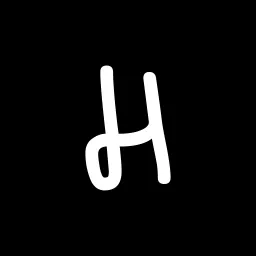
The data of
getPosts()
is cached on build time. Using noStore
can prevent it from being cached. Haha, I do not understand caching in deep actually. Hope that I am correct. 😂