GET Function on App Router
Answered
Polar bear posted this in #help-forum
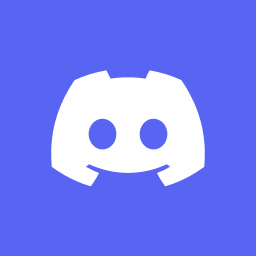
Polar bearOP
This is my code:
But for some reason, the value of email is always undefined. The API endpoint I'm using is
I've been dealing this for days and I don't know why it's returning as undefined. I tried checking req.query but still undefined.
Thank you in advance
export async function GET(req) {
try {
const email = await req.query.email;
if (!email) {
return NextResponse.json({ message: "Email query parameter is required." }, { status: 400 });
}
return NextResponse.json({ message: `Email received: ${email}` }, { status: 200 });
} catch (error) {
console.error(error);
return NextResponse.json({ error: error, message: "Encountered an error" }, { status: 500 });
}
}
But for some reason, the value of email is always undefined. The API endpoint I'm using is
/api/user?email=test@test.com
. I don't have any config for bodyParser. I even tried adding the config but still returning as undefined. But for some reason, from the same file, POST function works fine using await req.json(). I've been dealing this for days and I don't know why it's returning as undefined. I tried checking req.query but still undefined.
Thank you in advance
Answered by American
Can you try this?
export async function GET(req) {
try {
const email = req.nextUrl.searchParams.get("email");
if (!email) {
return NextResponse.json(
{ message: "Email query parameter is required." },
{ status: 400 }
);
}
return NextResponse.json(
{ message: `Email received: ${email}` },
{ status: 200 }
);
} catch (error) {
console.error(error);
return NextResponse.json(
{ error: error, message: "Encountered an error" },
{ status: 500 }
);
}
}
17 Replies
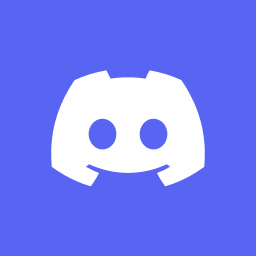
Polar bearOP
This is the response I'm getting:
{
"error": {},
"message": "Encountered an error"
}
{
"error": {},
"message": "Encountered an error"
}

Clown
Its very likely your link is getting messed up due to that @
Try printing the req.query object and the req.url to check it out
Also you can send the email inside the request header or body instead of doing this
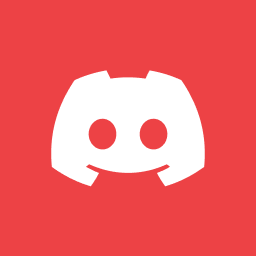
American
I'm doing it like this:
export function POST(request: NextRequest) {
const tag = request.nextUrl.searchParams.get("tag");
...
}
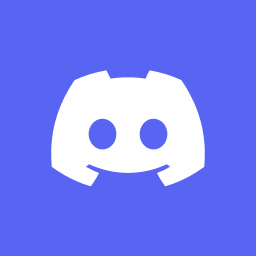
Polar bearOP
Thank you for the fast support. I tried printing these values and found out that req.query also returns undefined but for the req.url, it returns the correct url which is:
http://localhost:3000/api/user?email=test@test.com
I'm using the GET function so I can easily locate the data from my user list.
http://localhost:3000/api/user?email=test@test.com
I'm using the GET function so I can easily locate the data from my user list.
For my POST function, it works fine without any issues. req.json() returns a value.
But let me try using this on my GET
export async function POST(req) {
try {
const body = await req.json();
const { username, email, password } = body;
...
}
}
But let me try using this on my GET
Btw, I'm using js instead of typescript.

Clown
That and this are two different things. One is using the request body and the other is using query params
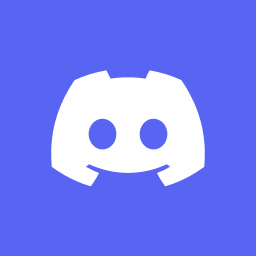
Polar bearOP
Yes correct. So by default, my API endpoint is correct and I'm getting the data. But for some reason, the GET function doesn't seem to get the value of my query. I'm not really sure what's the next step for this.
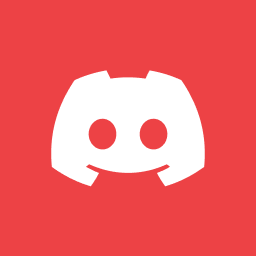
American
should still work
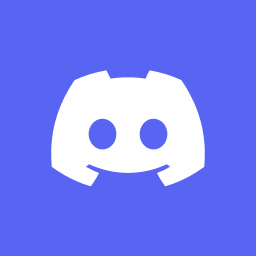
Polar bearOP
Thank you, For some reason, this returns the correct value: const test = await req.nextUrl.searchParams.get("email");. May I ask if why req.query is not returning any value but req.nextUrl returns a value?
I'm sorry, I'm new with the new features of Nextjs
I'm sorry, I'm new with the new features of Nextjs
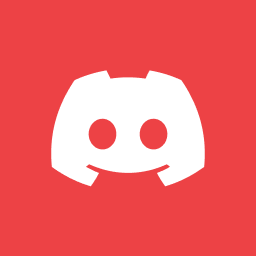
American
Can you try this?
export async function GET(req) {
try {
const email = req.nextUrl.searchParams.get("email");
if (!email) {
return NextResponse.json(
{ message: "Email query parameter is required." },
{ status: 400 }
);
}
return NextResponse.json(
{ message: `Email received: ${email}` },
{ status: 200 }
);
} catch (error) {
console.error(error);
return NextResponse.json(
{ error: error, message: "Encountered an error" },
{ status: 500 }
);
}
}
Answer
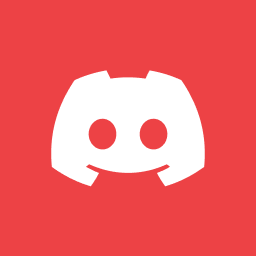
American
May I ask if why req.query is not returning any value but req.nextUrl returns a value?No idea actually, sorry!
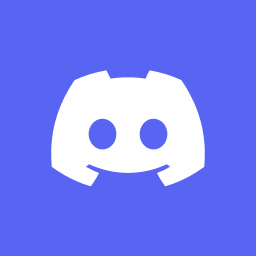
Polar bearOP
This works. Thank you so much! Here's my updated file:
export async function GET(req) {
try {
const email = await req.nextUrl.searchParams.get("email");
if (!email) {
return NextResponse.json({ message: "Email query parameter is required." }, { status: 400 });
}
const user = await prisma.user.findUnique({
where: { email: email },
});
return NextResponse.json({ message: `Email received: ${email}`, user: user }, { status: 200 });
} catch (error) {
console.error(error);
return NextResponse.json({ error: error, message: "Encountered an error" }, { status: 500 });
}
}
Thank you so much @American and @Clown for taking time in checking my concern! Really appreciate it!
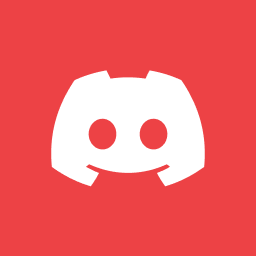
American
No worries, good luck with your app!