A better way to get and store user guilds.
Unanswered
Atlantic menhaden posted this in #help-forum
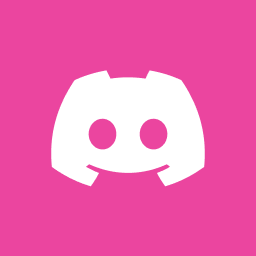
Atlantic menhadenOP
This is how i currently get and store user guilds:
i do it in the login callback so the guilds are only fetched upon login in. I do this to prevent api abuse and rate limiting from discord. But if the user is in a lot of servers i get a 431 where the headers are too large for the request. So i was wondering if anyone had any tips to store the guilds better.
import NextAuth from 'next-auth';
import DiscordProvider from 'next-auth/providers/discord';
import axios from 'axios';
import mongoConnect from '@util/mongo-connect';
export default NextAuth({
providers: [
DiscordProvider({
clientId: process.env.DISCORD_CLIENT_ID,
clientSecret: process.env.DISCORD_CLIENT_SECRET,
authorization: { params: { scope: 'identify guilds email' } },
}),
],
callbacks: {
async jwt({ token, account }) {
if (account) {
token.accessToken = account.access_token;
try {
const result = await axios({
url: 'https://discord.com/api/v10/users/@me/guilds',
method: 'GET',
headers: {
Authorization: `Bearer ${account.access_token}`,
},
})
token.guilds = result.data;
} catch (err) {
token.guilds = [];
}
}
return token
},
async session({ session, token }) {
session.accessToken = token.accessToken;
session.user.guilds = token.guilds
return session
},
},
})
i do it in the login callback so the guilds are only fetched upon login in. I do this to prevent api abuse and rate limiting from discord. But if the user is in a lot of servers i get a 431 where the headers are too large for the request. So i was wondering if anyone had any tips to store the guilds better.
2 Replies

Guri
Store only necessary data: Instead of storing all the guilds in the session, you could store only the necessary information. For example, you might only need the guild IDs and not the entire guild objects.
OR
Use a database: You could store the guilds in a database and only keep the user ID in the session. When you need the guilds, you can fetch them from the database using the user ID. This would require a database query each time you need the guilds, but it would keep your session data small.
OR
Use a database: You could store the guilds in a database and only keep the user ID in the session. When you need the guilds, you can fetch them from the database using the user ID. This would require a database query each time you need the guilds, but it would keep your session data small.
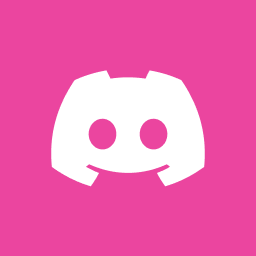
Atlantic menhadenOP
thank you