Can't use fs with client component
Unanswered
Grand Griffon Vendéen posted this in #help-forum
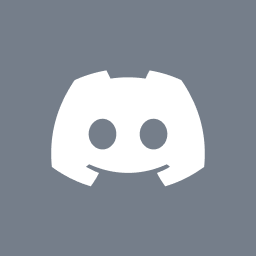
Grand Griffon VendéenOP
I'm trying to create a google drive file using googleapi. The <button> inside my client component calls the function to create a file. I believe this function uses fs, and it's complaining because I can't use fs in a client component.
So how am I suppose to create a button that runs this function that can only be called from server components, but I can only create buttons in client components?
'use client'
import React from 'react'
import { drive as googleDrive, auth } from '@googleapis/drive'
async function createFile() {
const client = new auth.OAuth2(
process.env.GOOGLE_ID as string,
process.env.GOOGLE_SECRET as string,
"http://localhost:3000/api/auth/callback/google"
);
const drive = googleDrive(
{
version: "v3",
auth: client
}
)
const newFile = drive.files.create({
requestBody: {
name: "My first file!",
mimeType: 'text/plain'
},
media: {
mimeType: 'text/plain',
body: "Some content"
}
})
}
const NewBoxButton = () => {
return (
<button onClick={async() => createFile()}>
</button>
)
}
export default NewBoxButton
So how am I suppose to create a button that runs this function that can only be called from server components, but I can only create buttons in client components?
10 Replies
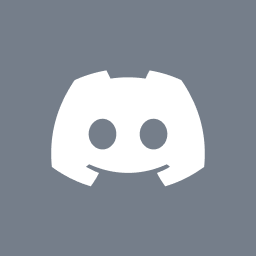
Grand Griffon VendéenOP
Here is the error: ./node_modules/gcp-metadata/build/src/gcp-residency.js:19:13
Module not found: Can't resolve 'fs'
https://nextjs.org/docs/messages/module-not-found
Import trace for requested module:
./node_modules/gcp-metadata/build/src/index.js
./node_modules/google-auth-library/build/src/index.js
./node_modules/googleapis-common/build/src/index.js
./node_modules/@googleapis/drive/build/index.js
./src/app/components/NewBoxButton.tsx
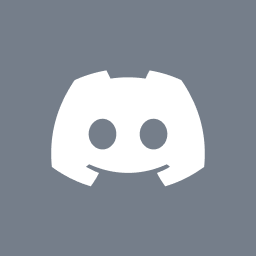
Grand Griffon VendéenOP
is getServerSideProps the answer? :D
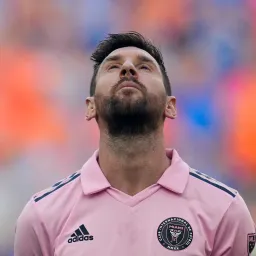
Ray
the answer should be turn
createFile
to a server action
Alfonsus Ardani
What are you tring to do? Where do you want to create a file? In the server? in Google Drive? in the client's computer?
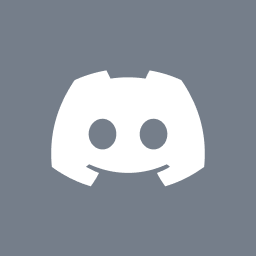
Grand Griffon VendéenOP
In the users google drive. I use nextauth to sign in the user

Alfonsus Ardani
then you need to find the Google Drive specific API to create a file
FS is to literally create a file in the server's folder....
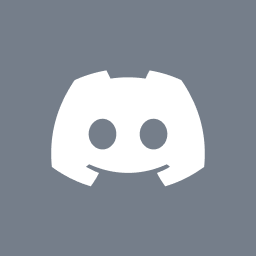
Grand Griffon VendéenOP
Idk why it’s referring to fs. I’m calling googleDrive.files.create()
googleDrive is from an import

Alfonsus Ardani
Perhaps this API is only meant to be run in the server and not in the client. Have you checked?