Getting results as undefined
Answered
Wuchang bream posted this in #help-forum
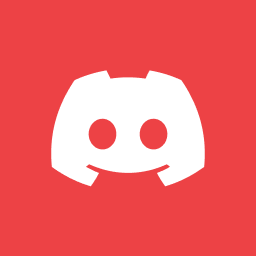
Wuchang breamOP
I am trying to implement server side rendering using nextjs app router but I am getting results as undefined.
I even tried logging the movies variable and i am getting the required result.
I even tried logging the movies variable and i am getting the required result.
import config from "@/config.json";
// Components
import Banner from "@/components/Banner";
const apiKey = process.env.API_KEY;
const Home: React.FC<{}> = async () => {
const movies = await getPopularMovies();
return (
<div className="w-full h-[110vh] grid grid-rows-2 align-center justify-items-center py-10">
<Banner
movieName={movies.results[0].original_title}
movieCover={`${config.tmdbImageURL}${movies.results[0].backdrop_path}`}
/>
</div>
);
};
const getPopularMovies = async () => {
"use server";
var json;
try {
const data = await fetch(
`https://api.themoviedb.org/3/movie/popular?language=en-US&page=1&api_key=${apiKey}`,
{
method: "GET",
cache: "no-store",
}
);
json = await data.json();
} catch (err) {
console.error(err);
}
return json;
};
export default Home;

Answered by Ray
first, you don't need to use
'use server'
here and try thisimport config from "@/config.json";
// Components
import Banner from "@/components/Banner";
const apiKey = process.env.API_KEY;
const Home: React.FC<{}> = async () => {
const movies = await getPopularMovies();
return (
<div className="w-full h-[110vh] grid grid-rows-2 align-center justify-items-center py-10">
<Banner
movieName={movies?.results[0].original_title}
movieCover={`${config.tmdbImageURL}${movies.results[0].backdrop_path}`}
/>
</div>
);
};
const getPopularMovies = async () => {
const data = await fetch(
`https://api.themoviedb.org/3/movie/popular?language=en-US&page=1&api_key=${apiKey}`,
{
method: "GET",
cache: "no-store",
}
);
return data.json();
};
export default Home;
53 Replies
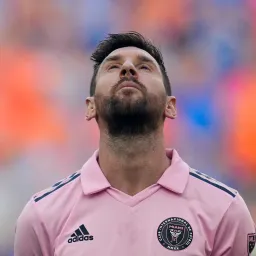
first, you don't need to use
'use server'
here and try thisimport config from "@/config.json";
// Components
import Banner from "@/components/Banner";
const apiKey = process.env.API_KEY;
const Home: React.FC<{}> = async () => {
const movies = await getPopularMovies();
return (
<div className="w-full h-[110vh] grid grid-rows-2 align-center justify-items-center py-10">
<Banner
movieName={movies?.results[0].original_title}
movieCover={`${config.tmdbImageURL}${movies.results[0].backdrop_path}`}
/>
</div>
);
};
const getPopularMovies = async () => {
const data = await fetch(
`https://api.themoviedb.org/3/movie/popular?language=en-US&page=1&api_key=${apiKey}`,
{
method: "GET",
cache: "no-store",
}
);
return data.json();
};
export default Home;
Answer
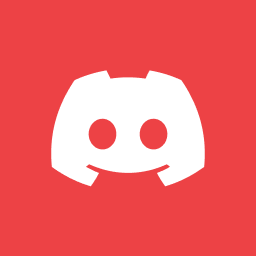
Wuchang breamOP
why no need to use 'use server'?
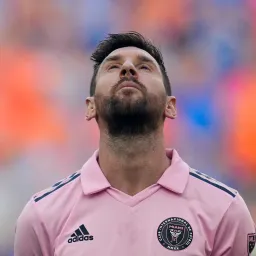
use server
is only for server actionyou are not using server action here
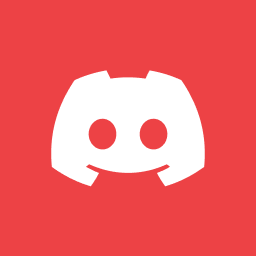
Wuchang breamOP
and how should i implement getServerSideProps of page router similar to app router?
can u explain with example?
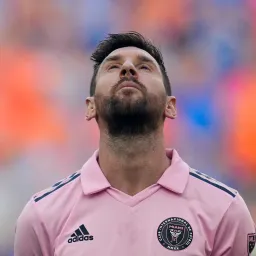
just like this
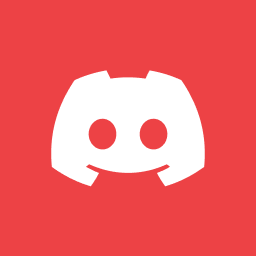
Wuchang breamOP
wdym by a server action
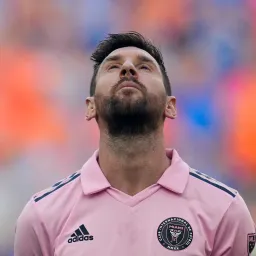
the doc have good explanation
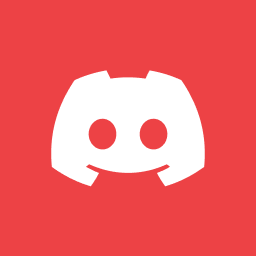
Wuchang breamOP
but i am sending a request to an api of a backend rather than using with useEffect hook
so ig its a server action only
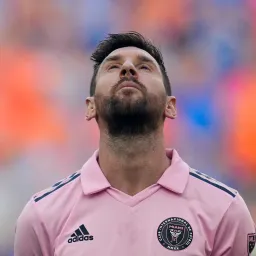
I don't see you are using
useEffect
here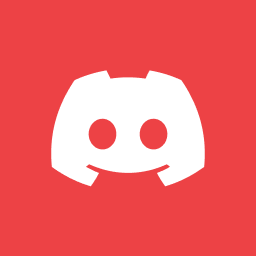
Wuchang breamOP
yeah cause i rendered it as a server side rendering
to hide my api keys ykyk
rather than fetching on client side
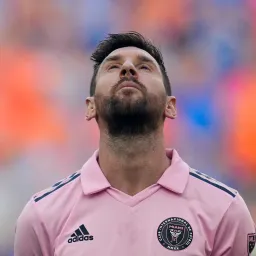
wdym by this?
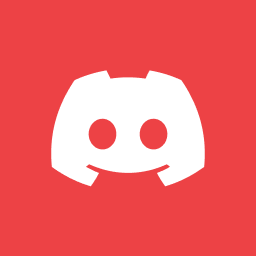
Wuchang breamOP
uh like
i dont understand the meaning of server actions
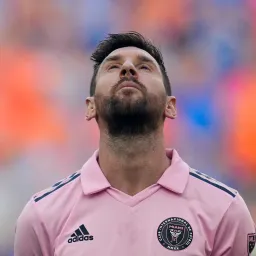
read the doc here
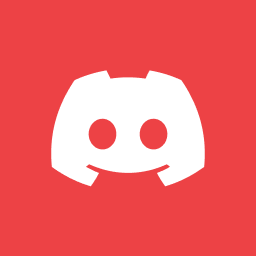
Wuchang breamOP
you basically use it to send an api request only right
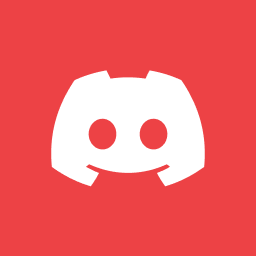
Wuchang breamOP
oh i see
and i did as you said but i am getting an error of "fetch failed"
even removed the "use server"
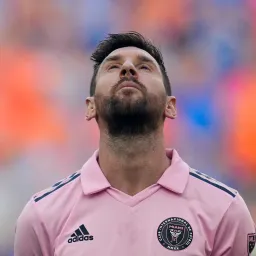
then check the url and the api key
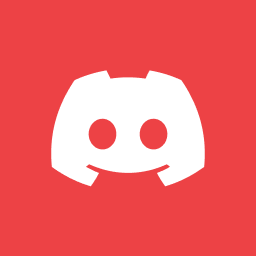
Wuchang breamOP
its perfectly fine
i am logging the results and i can see the json data
in terminal
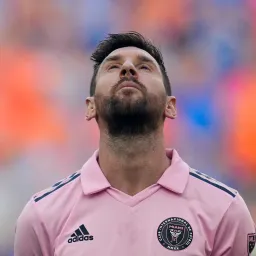
so its not fetch failed?
if you can see the result in terminal
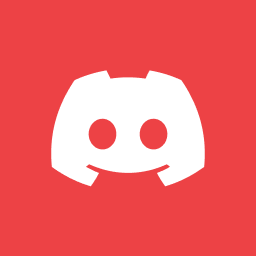
Wuchang breamOP

this is the component i am passing the props into
"use client";
import Link from "next/link";
import Image from "next/image";
import { Montserrat } from "next/font/google";
const montserrat = Montserrat({
display: "swap",
weight: ["200", "400", "500", "600", "700"],
subsets: ["latin-ext"],
});
type BannerProps = {
movieName: string;
movieDescription?: string;
movieCover: string;
movieLink?: string;
movieGenre?: string[];
};
const Banner: React.FC<BannerProps> = ({
movieName,
movieDescription,
movieCover,
movieLink,
movieGenre,
}) => {
return (
<div className="w-[90vw] h-[75vh] bg-oxford-blue grid grid-cols-3">
<section>
<h1 className="text-white">{movieName}</h1>
</section>
<section className="flex items-center justify-center">
{/* <Image src={movieCover} alt={movieName} fill={true} className="" /> */}
</section>
</div>
);
};
export default Banner;
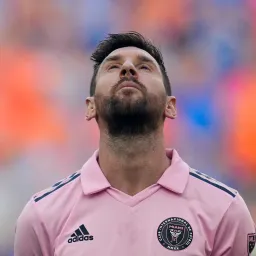
where do you see the json data?
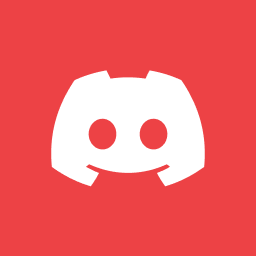
Wuchang breamOP
the terminal
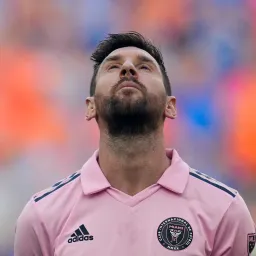
you mean this?
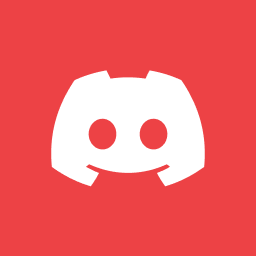
Wuchang breamOP
yus
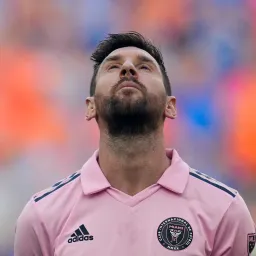
check your url and the api key
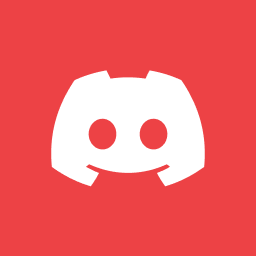
Wuchang breamOP
should i be using "use server" in the banner component?
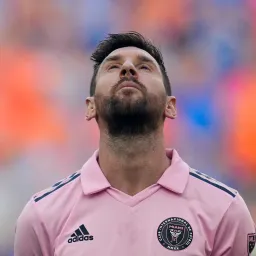
no
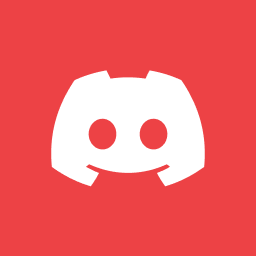
Wuchang breamOP
o
see

o nvm its working now
idk how
so this data is being fetched on the server side and i cant use redux with it right?
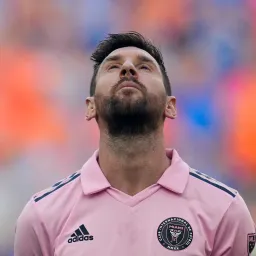
you could pass the data to it
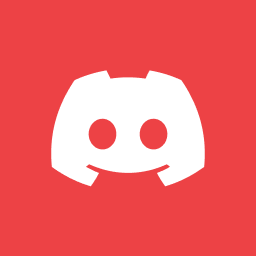
Wuchang breamOP
oh
ok
i will try
thank you
but there is not really a point in using that rn
ig