Update cookie in server
Answered
Banjara Hound posted this in #help-forum
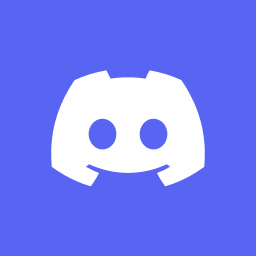
Banjara HoundOP
i have a method that check token is valid or not.
and i get exception that cookie can only modified inside server action or route handler.
then i move all code inside tokenIsValid to route handler (in other word nextjs api route)
and in route handler cookie set not work. when i check inside middleware i get undefined.
so how to set and update cookie in next js?
"use server";
import {cookie} from "next/headers"
export function tokenIsValid(){
// in here i check token is valid or not if is not get new token and set it to cookie with cookie method that came from next/headers
}
and i get exception that cookie can only modified inside server action or route handler.
then i move all code inside tokenIsValid to route handler (in other word nextjs api route)
and in route handler cookie set not work. when i check inside middleware i get undefined.
so how to set and update cookie in next js?
Answered by Anay-208
this is how I got it working for middleware:
and for server functions,
import { NextResponse } from 'next/server'
import type { NextRequest } from 'next/server'
// This function can be marked `async` if using `await` inside
function setTokenCookie(response: NextResponse): string {
// generate a token
const token = "idk";
response.cookies.set('token', token)
return token
}
export function middleware(request: NextRequest) {
// Given incoming request /home
let response = NextResponse.next()
// Set a cookie to hide the banner
const token = setTokenCookie(response)
// Response will have a `Set-Cookie:show-banner=false;path=/home` header
return response
}
// See "Matching Paths" below to learn more
export const config = {
matcher: '/',
}
and for server functions,
cookies().set()
is enough41 Replies
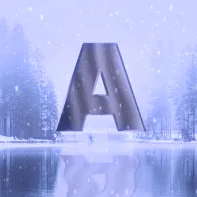
you can use method
Doc link: https://nextjs.org/docs/app/api-reference/functions/cookies
cookies().get
in server side to get cookies, and update it using cookies().update
Doc link: https://nextjs.org/docs/app/api-reference/functions/cookies
can you elaborate further what you need to do ?
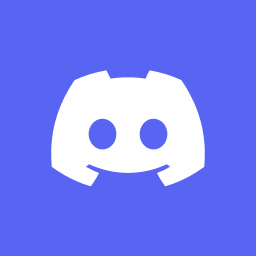
Banjara HoundOP
there is no update method.
only delete get has set getAll
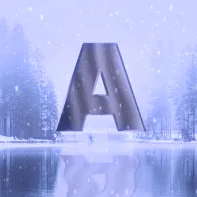
just use set method, it'll update the cookie
'use server'
import { cookies } from 'next/headers'
async function create(data) {
cookies().set('name', 'lee')
// or
cookies().set('name', 'lee', { secure: true })
// or
cookies().set({
name: 'name',
value: 'lee',
httpOnly: true,
path: '/',
})
}
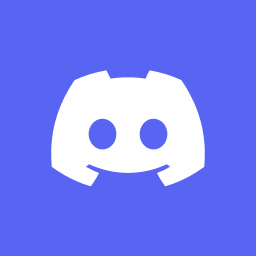
Banjara HoundOP
and as i said it's not work. it's not set any cookie.
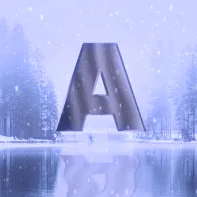
can you send your code where you're trying to use it
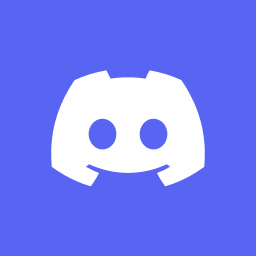
Banjara HoundOP
i use it inside middleware.
and code is like this.
cookies().set("random", `${Math.random()}`, { secure: true, httpOnly: true, sameSite: true, });
and in middleware is something like this:
tokenIsValid();
console.log(request.cookies.get("random")?.value)
this method is server action right?
because if i use that method i get exception that cookie can be modified inside server action or route handler.
it's not work.
it's not work.
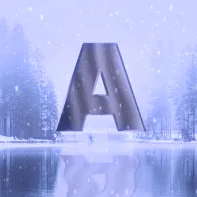
let me see
Ya, its same for me after trying, I'll give you a solution
@Banjara Hound why don't you set cookies using nextResponse?
This code is working for me:
import { NextResponse } from 'next/server'
import type { NextRequest } from 'next/server'
// This function can be marked `async` if using `await` inside
export function middleware(request: NextRequest) {
// Given incoming request /home
let response = NextResponse.next()
// Set a cookie to hide the banner
response.cookies.set('show-banner', 'false')
// Response will have a `Set-Cookie:show-banner=false;path=/home` header
return response
}
// See "Matching Paths" below to learn more
export const config = {
matcher: '/',
}
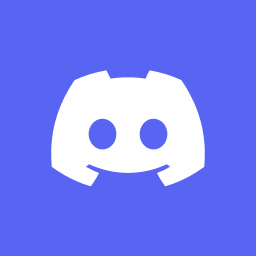
Banjara HoundOP
i want create another function for this. how to do that.
and just call it inside middleware and anywhere else that is server component. is that possible?
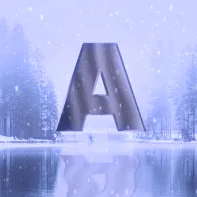
You want to create another function for middleware, which'll set cookies?
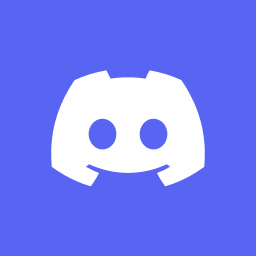
Banjara HoundOP
i want create a function that run in server and can be called in any where that can get and set cookie.
that function return valid token. if there is any.
that function return valid token. if there is any.
so return type of tokenIsValid is string or null.
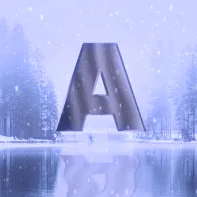
so you want to create a function, which'll set the cookies of the user and return the NextResponse.next() with cookies?
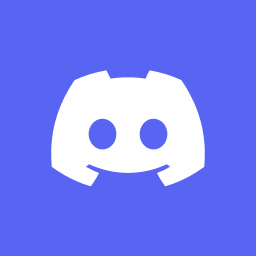
Banjara HoundOP
no just set cookie and return token as string.
return type of my function is string or null. not NesxtResponse or anything else.
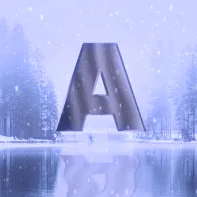
So will you be using it in middleware or server actions/route.ts?
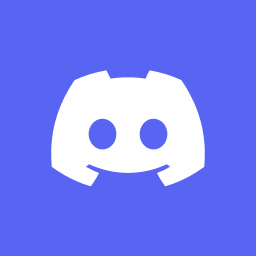
Banjara HoundOP
and one thing
when i have a route like this "http://localhost:3000/api/random"
why i can't call it like this.
when i have a route like this "http://localhost:3000/api/random"
why i can't call it like this.
await fetch("api/random")
or
await fetch("/api/random"
and have to call it with full url.
await fetch("http://localhost:3000/api/random")
both maybe in server action may be in middleware.
if that is not possible two function one for middleware and one for server action.
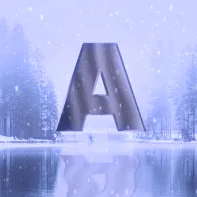
Create a seperate #help-forum for it. This will make it easier when other users are searching
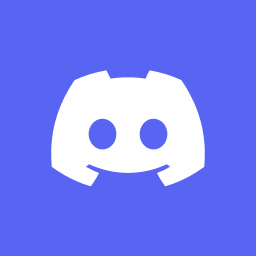
Banjara HoundOP
ok. i will do it after this problem solved
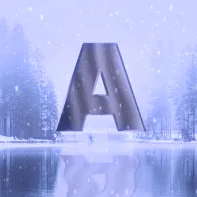
this is how I got it working for middleware:
and for server functions,
import { NextResponse } from 'next/server'
import type { NextRequest } from 'next/server'
// This function can be marked `async` if using `await` inside
function setTokenCookie(response: NextResponse): string {
// generate a token
const token = "idk";
response.cookies.set('token', token)
return token
}
export function middleware(request: NextRequest) {
// Given incoming request /home
let response = NextResponse.next()
// Set a cookie to hide the banner
const token = setTokenCookie(response)
// Response will have a `Set-Cookie:show-banner=false;path=/home` header
return response
}
// See "Matching Paths" below to learn more
export const config = {
matcher: '/',
}
and for server functions,
cookies().set()
is enoughAnswer
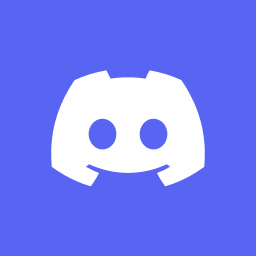
Banjara HoundOP
server function is a function that has "use server" or "formData"?
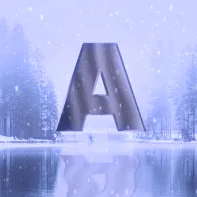
server action or route handler. server action = 'use server', and route.ts = route handler
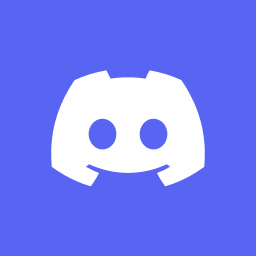
Banjara HoundOP
thanks for helping.
now i know how to create twp separate function that will work on middleware and server action.
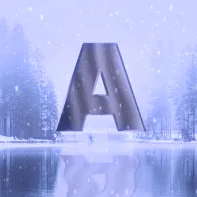
Alr, can you mark my answer as a solution?
and create a seperate help forum for that route