POST Request Body Returning as Undefined
Answered
Cornish Rex posted this in #help-forum
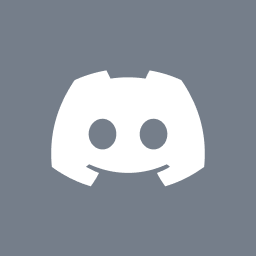
Cornish RexOP
I have an api request to my backend api to check whether a license key is valid or not, but the request is not working properly.
page.tsx
route.js
I have tried troubleshooting for hours but nothing has worked.
page.tsx
'use client';
import React from 'react'
import { useState } from 'react';
import { Button } from '@components/ui/button'
import { Card, CardContent, CardDescription, CardFooter, CardHeader, CardTitle } from '@components/ui/card'
import { Input } from '@components/ui/input'
const page = () => {
const [inputValue, setInputValue] = useState('')
const checkKey = async () => {
console.log(`Param: ${inputValue}`);
const res = await fetch('/api/auth/checkkey', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
key: inputValue,
}),
});
const data = await res.json();
console.log(data);
};
const closeTab = () => {
window.close()
}
return (
<div style={{ display: 'flex', justifyContent: 'center', alignItems: 'center', height: '100vh' }}>
<Card className="dark">
<CardHeader>
<CardTitle>License Key</CardTitle>
<CardDescription>Please enter your license key to access /bleeds eye</CardDescription>
</CardHeader>
<CardContent>
<Input value={inputValue} onChange={(e) => setInputValue(e.target.value)} />
</CardContent>
<CardFooter>
<Button className="dark mr-3" onClick={checkKey}>Check Key</Button>
<Button className="dark" variant="outline" onClick={closeTab}>Cancel</Button>
</CardFooter>
</Card>
</div>
)
}
export default page
route.js
import { connectToDB } from "@utils/database";
import Keys from "@models/keys";
export const POST = async (request) => {
try {
connectToDB();
const token = request.body.key
console.log(`Key: ${token}`);
const newKey = new Keys({
key: token,
expires: new Date(Date.now() + 30 * 24 * 60 * 60 * 1000),
uses: 100,
suspended: false,
});
await newKey.save();
return new Response(JSON.stringify({ success: true }), {
headers: { "Content-Type": "application/json" },
});
} catch (error) {
return new Response(JSON.stringify({ success: false + error }), {
headers: { "Content-Type": "application/json" },
});
}
};
I have tried troubleshooting for hours but nothing has worked.
Answered by ncls.
The
Try this in your
body
property of your request
is a stream.Try this in your
route.js
:const { token } = await request.json();
5 Replies
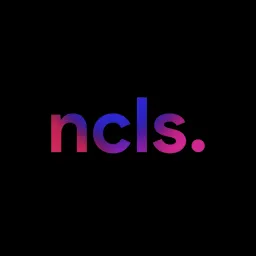
@ncls. The `body` property of your `request` is a stream.
Try this in your `route.js`:
JS
const { token } = await request.json();
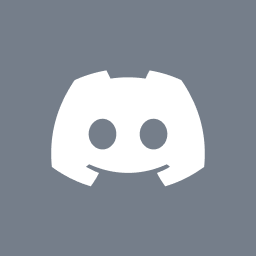
Cornish RexOP
still getting undefined
wait
i got it
we good now