connect for websocket for notification.
Unanswered
Sun bear posted this in #help-forum
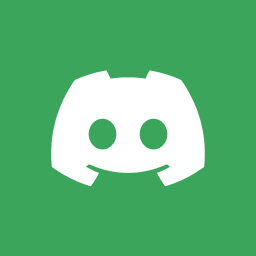
Sun bearOP
i want to connect for websocket for notification. i am using nextjs for frontend. backend give me a
1 Reply
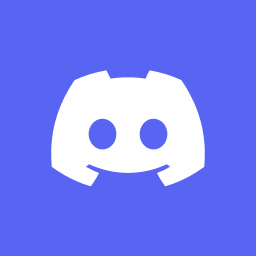
American black bear
Install WebSocket Library:
First, you'll need a WebSocket library. socket.io-client is a popular choice. Install it using npm:
Create a WebSocket Connection:
In your Next.js component or page, create a WebSocket connection. For example, in a React component:
Ensure that you replace 'your-backend-url' with the actual URL of your WebSocket server.
Backend Integration:
Ensure that your backend is set up to handle WebSocket connections. If you're using Node.js, you might have a setup similar to the following:
First, you'll need a WebSocket library. socket.io-client is a popular choice. Install it using npm:
npm install socket.io-client
Create a WebSocket Connection:
In your Next.js component or page, create a WebSocket connection. For example, in a React component:
import { useEffect } from 'react';
import io from 'socket.io-client';
const MyComponent = () => {
useEffect(() => {
// Replace 'your-backend-url' with the actual URL of your WebSocket server
const socket = io('your-backend-url');
// Handle events from the server
socket.on('notification', (data) => {
console.log('Received notification:', data);
// Update your component state or trigger a notification UI
});
// Clean up the socket connection when the component unmounts
return () => {
socket.disconnect();
};
}, []);
return (
<div>
{/* Your component content */}
</div>
);
export default MyComponent;
};
Ensure that you replace 'your-backend-url' with the actual URL of your WebSocket server.
Backend Integration:
Ensure that your backend is set up to handle WebSocket connections. If you're using Node.js, you might have a setup similar to the following:
const httpServer = require('http').createServer();
const io = require('socket.io')(httpServer);
io.on('connection', (socket) => {
console.log('A user connected');
// Example: Send a notification to all connected clients
setInterval(() => {
socket.emit('notification', { message: 'Hello, this is a notification!' });
}, 5000); // Send a notification every 5 seconds
socket.on('disconnect', () => {
console.log('User disconnected');
});
});
const PORT = process.env.PORT || 3001;
httpServer.listen(PORT, () => {
console.log(`Server listening on port ${PORT}`);
});