useState hook does not update the UI unless the page is refreshed :(
Unanswered
Exotic Shorthair posted this in #help-forum
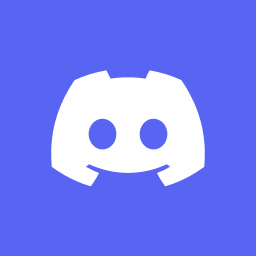
Exotic ShorthairOP
i tried using the useEffect and the (prevState) with the updater function but still doesn't work
13 Replies
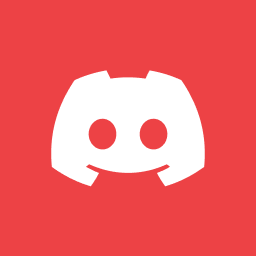
Chausie
paste your code here please
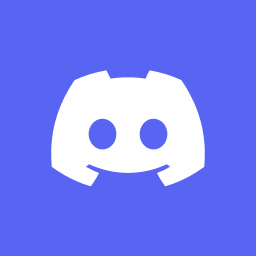
Exotic ShorthairOP
okay, one moment
"use client";
import { useEffect, useState } from "react";
import CardSheet from "./CardSheet";
function Cart() {
const [forceUpdate, setForceUpdate] = useState(false);
const [cart, setCart] = useState([]);
useEffect(() => {
const handleStorageChange = () => {
const storedCart = localStorage.getItem("cart");
setCart(storedCart ? JSON.parse(storedCart) : []);
setForceUpdate((prevState) => !prevState);
};
handleStorageChange();
// Listen for changes to localStorage
window.addEventListener("storage", handleStorageChange);
return () => {
// Remove the event listener when the component unmounts
window.removeEventListener("storage", handleStorageChange);
};
}, []); // The empty dependency array ensures the effect runs only once
return (
<div>
<div className="relative">
<CardSheet cart={cart} />
</div>
</div>
);
}
export default Cart;
import { useEffect, useState } from "react";
import CardSheet from "./CardSheet";
function Cart() {
const [forceUpdate, setForceUpdate] = useState(false);
const [cart, setCart] = useState([]);
useEffect(() => {
const handleStorageChange = () => {
const storedCart = localStorage.getItem("cart");
setCart(storedCart ? JSON.parse(storedCart) : []);
setForceUpdate((prevState) => !prevState);
};
handleStorageChange();
// Listen for changes to localStorage
window.addEventListener("storage", handleStorageChange);
return () => {
// Remove the event listener when the component unmounts
window.removeEventListener("storage", handleStorageChange);
};
}, []); // The empty dependency array ensures the effect runs only once
return (
<div>
<div className="relative">
<CardSheet cart={cart} />
</div>
</div>
);
}
export default Cart;
chat gpt did it's thing but still doesn't work
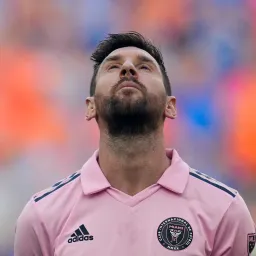
Ray
chatgpt suck
where are you firing the storage event?
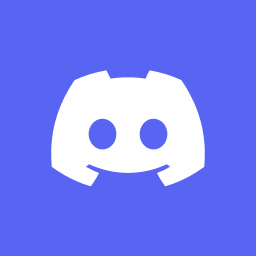
Exotic ShorthairOP
just in this file
i don't know how else can i get the cart items after they change in the local storage
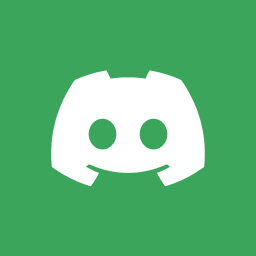
Plott Hound
i'll try to have a look at this tonight when i finish work but at first glance i would consider a centralized function for updating the cart
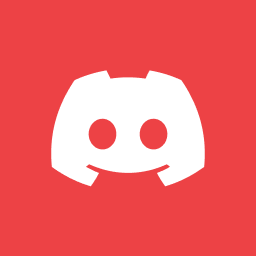
Chausie
"use client";
import { useCallback, useEffect, useState } from "react";
import CardSheet from "./CardSheet";
function Cart() {
const [cart, setCart] = useState([]);
const handleStorageChange = useCallback(() => {
const storedCart = localStorage.getItem("cart");
if (storedCart) {
setCart(JSON.parse(storedCart));
}
}, []);
useEffect(() => {
handleStorageChange();
// Listen for changes to localStorage
window.addEventListener("storage", handleStorageChange);
return () => {
// Remove the event listener when the component unmounts
window.removeEventListener("storage", handleStorageChange);
};
}, []); // The empty dependency array ensures the effect runs only once
return (
<div>
<div className="relative">
<CardSheet cart={cart} />
</div>
</div>
);
}
export default Cart;
Also check where you fire the event, It is also worth looking in better localStorage api's if you use it alot like localForage
https://www.npmjs.com/package/localforage which gives you async await.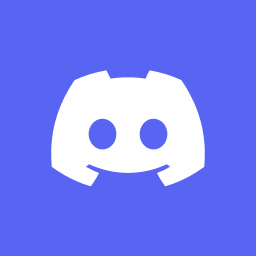
Exotic ShorthairOP
interesting
okay noted
btw anyone got link to a proper tutorial where they build a shopping cart with react using the "centralized" functions