How to make loader to count 100% if window loads
Unanswered
Ojos Azules posted this in #help-forum
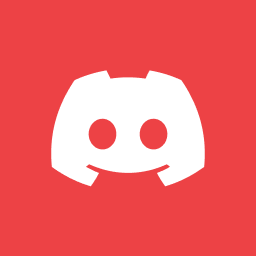
Ojos AzulesOP
Hello, I would like my loading to start from 0 and count until the window is fully loaded. If the window is loaded, then it should immediately go to 100%.
This is my page.tsx with Suspense Boundary
This is my loading.tsx
This is my page.tsx with Suspense Boundary
import getCategories from "@/actions/get-category";
import getProducts from "@/actions/get-products";
import getVarieties from "@/actions/get-varieties";
import Menu from "@/components/menu";
import { Suspense } from "react";
import Loading from "./loading";
export const revalidate = 0;
const HomePage = async () => {
const categories = await getCategories();
const products = await getProducts({
isFeatured: true,
});
const varieties = await getVarieties();
const filteredVarieties = varieties.filter(
(item) => item.categoryName === "Coffees"
);
const sortedProducts = products.sort((b, a) => {
const dateA = new Date(a.createdAt);
const dateB = new Date(b.createdAt);
return dateB.getTime() - dateA.getTime();
});
return (
<div className="w-full 2xl:px-4 md:px-12 xl:px-[0.25rem] px-0 overflow-hidden min-h-screen">
<Suspense fallback={<Loading />}>
<Menu
items={sortedProducts}
category={categories}
varieties={filteredVarieties}
/>
</Suspense>
</div>
);
};
export default HomePage;
This is my loading.tsx
"use client";
import React, { useState, useEffect } from "react";
import Styles from "@/app/Styles/loading.module.css";
const Loading = () => {
return (
<div className="min-h-screen w-full flex justify-center items-center">
<div className={`${Styles.loading_box}`}>
<p className={`${Styles.loading_title}`}>Loading</p>
<div className={`${Styles.loading_circle}`}>
<p className={`${Styles.loading_count}`}>
<span id="loadingNumber">0</span>%
</p>
</div>
</div>
</div>
);
};
export default Loading;
6 Replies
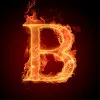
B33fb0n3
you got the right thing. Inside the layout you can add a interval to update the number. Then just display the number. That's the plain way without a library. You can also use a library for that. The loading will be hidden when the page.js finished loading @Ojos Azules
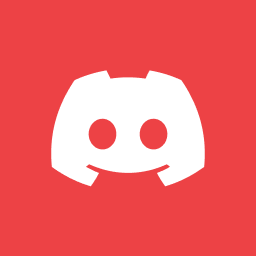
Ojos AzulesOP
I think suspense removes the component and doesnt let it to go 100% so if i want to make it to go 100% first and then load the page i should remove the suspense and make it with if statements
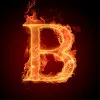
B33fb0n3
yea, the suspense will be hidden directly when the page.js finished loading. So to remove the suspense can work ðŸ‘
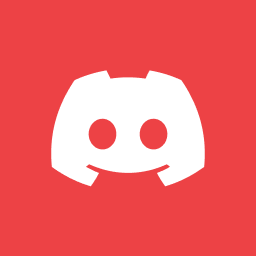
Ojos AzulesOP
Thank you so much
I will leave the suspense its better i think
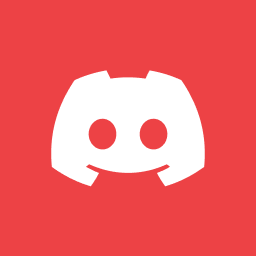
Ojos AzulesOP
Anyway, thank you so much! @B33fb0n3