React Query Problem
Answered
Saltwater Crocodile posted this in #help-forum
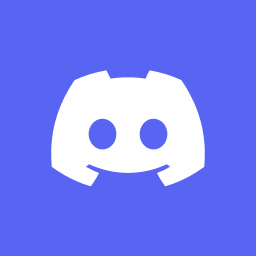
Saltwater CrocodileOP
Hello Guys,
I have a Problem on one of my current Projects on the signup Page with React Query. From the official Documentation i can read that this should work.
Here i have the registerUser function with the axios:
This then gets called in the client component on the top:
And then on the onSubmit function the mutateAsync function is called:
When console logging mutation all of the properties are undefined so i can't forward from this. When calling the submit a second time the properties get filled and it works. Does anyone know why this occurs?
I have a Problem on one of my current Projects on the signup Page with React Query. From the official Documentation i can read that this should work.
Here i have the registerUser function with the axios:
export const registerUser = async (values: RegisterUserFormData) => {
return await axios.post(BACKEND_URL + '/auth/signup', values, {
headers: {
'Content-Type': 'application/json',
Accept: 'application/json',
},
})
}
This then gets called in the client component on the top:
const mutation = useMutation({ mutationFn: registerUser });
And then on the onSubmit function the mutateAsync function is called:
const submitData = async (formData: RegisterUserFormData) => {
const name = formData['name'];
const email = formData['email'];
const password = formData['password'];
const values = {
name,
email,
password,
};
await mutation.mutateAsync(values);
console.log('MUTATION: ', mutation);
if (mutation.isSuccess) {
toast.success(`${toaster('register')}`, {
description: `${toaster('registerdescription')}`,
duration: 2000,
});
setTimeout(() => {
window.location.href = '/signin';
}, 2000);
} else if (mutation.isError) {
toast.error(`${toaster('error')}`, {
description: `${toaster('errordescription')}`,
});
setTimeout(() => {
window.location.reload();
}, 2000);
}
};
When console logging mutation all of the properties are undefined so i can't forward from this. When calling the submit a second time the properties get filled and it works. Does anyone know why this occurs?
Answered by Wesley Janse
Exactly what I meant yes. You should have a look at using the callback functions for "isSuccess and isError":
the mutate/mutateAsync function returned from react-query also return calback functions:
From the docs: (react-query.v4):
Looking at this you could refactor your code to look something like the following:
the same for onError
the mutate/mutateAsync function returned from react-query also return calback functions:
From the docs: (react-query.v4):
mutateAsync: (variables: TVariables, { onSuccess, onSettled, onError }) => Promise<TData>
Looking at this you could refactor your code to look something like the following:
await mutation.mutateAsync(values, {
onSuccess: () => {
toast.success(`${toaster('register')}`, {
description: `${toaster('registerdescription')}`,
duration: 2000,
});
setTimeout(() => {
window.location.href = '/signin';
}, 2000);
},
onError: () => { ...Error logic here }
});
the same for onError
49 Replies
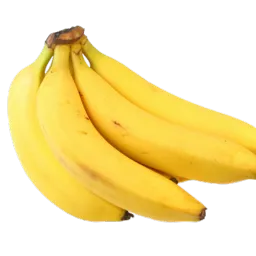
You mean that the
formData
is undefined or which values are undefined?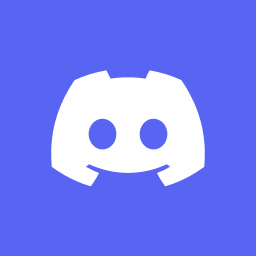
Saltwater CrocodileOP
no the mutation is undefined
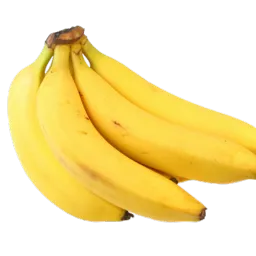
Oh sorry yes i misread it
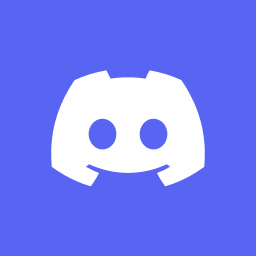
Saltwater CrocodileOP
So all the properties like isSuccess isError data and so on
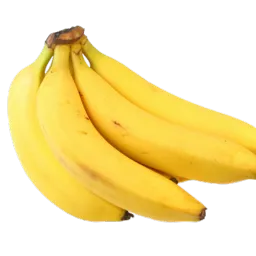
oke but mutateAsync is working? or are you also getting an undefined error on this?
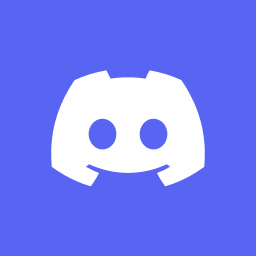
Saltwater CrocodileOP
what do you mean? The user gets created with the right values
If you meant that
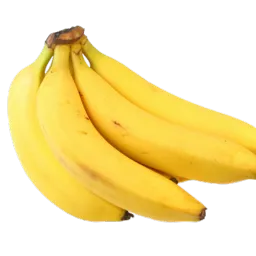
Exactly what I meant yes. You should have a look at using the callback functions for "isSuccess and isError":
the mutate/mutateAsync function returned from react-query also return calback functions:
From the docs: (react-query.v4):
Looking at this you could refactor your code to look something like the following:
the same for onError
the mutate/mutateAsync function returned from react-query also return calback functions:
From the docs: (react-query.v4):
mutateAsync: (variables: TVariables, { onSuccess, onSettled, onError }) => Promise<TData>
Looking at this you could refactor your code to look something like the following:
await mutation.mutateAsync(values, {
onSuccess: () => {
toast.success(`${toaster('register')}`, {
description: `${toaster('registerdescription')}`,
duration: 2000,
});
setTimeout(() => {
window.location.href = '/signin';
}, 2000);
},
onError: () => { ...Error logic here }
});
the same for onError
Answer
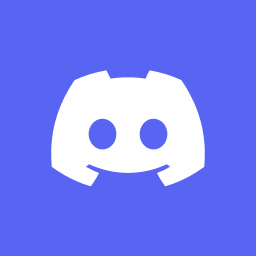
Saltwater CrocodileOP
Oh okay
But isnt there a way to deescturcture the isLoading
Because whils isLoading is true there is a small spinner on the saubmit button that indicates that something is happening
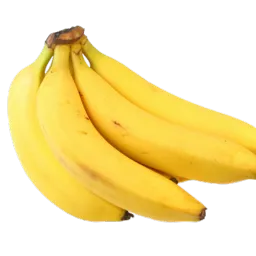
isloading should be fine to destructure from the mutation object
I'm not sure why the status is undefined in the onsubmit, have to look deeper into it. I always tent to use the callback structure for these usecases
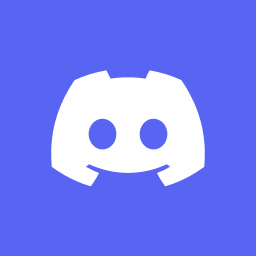
Saltwater CrocodileOP
okay thank you very much
I^ll try it in a bit
Seems like neon tech is down
:(
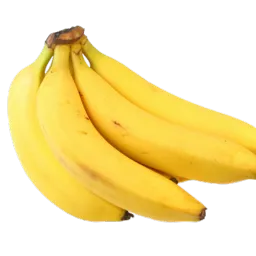
Possibly also update
to router.push('/signin')
window.location.href = '/signin';
to router.push('/signin')
No worries, let me know how it goes!
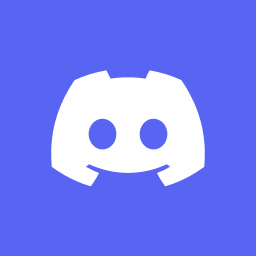
Saltwater CrocodileOP
From usRouter?
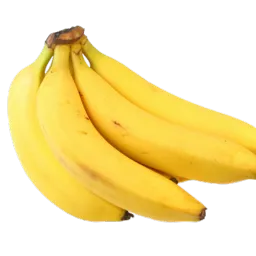
Yes
useRouter from 'next/navigation' (for app router)
useRouter from 'next/router' (for pages router)
useRouter from 'next/router' (for pages router)
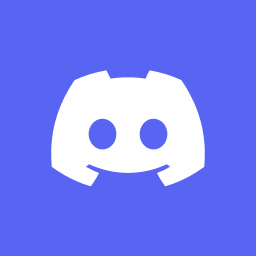
Saltwater CrocodileOP
Thank you
I^ll text you once i was able to try it
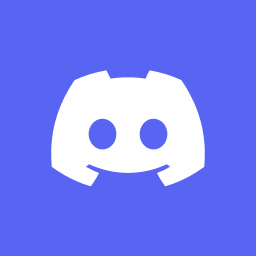
Saltwater CrocodileOP
Now it works
Thank you very much
Finally i can move on
haha
@Wesley Janse
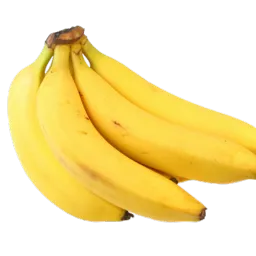
Great to hear!
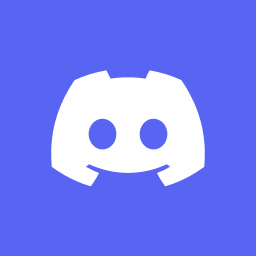
Saltwater CrocodileOP
I was searching on whole youtube but i didnt find anyhting. The last good tutorials were like 2 years ago
And it didnt work
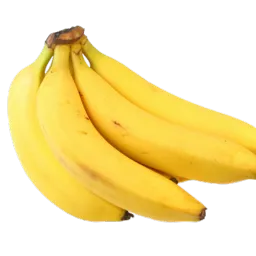
React query has great documentation and examples on the site
You should always look there
Dont forgot the close the topic/mark it as solved 😉
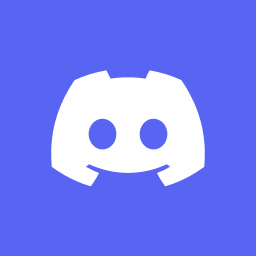
Saltwater CrocodileOP
Yes i will
Thank you
@Wesley Janse do you also know some things about next auth?
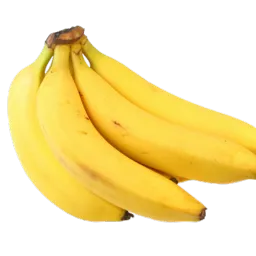
Yes i do,
Are you on the app router by any means?
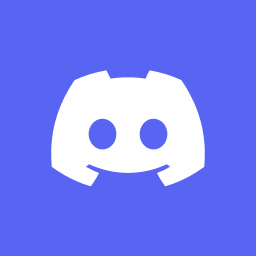
Saltwater CrocodileOP
Yes i am
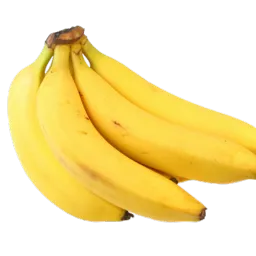
Nextjs released a free course including nextauth yesterday
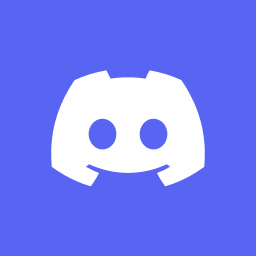
Saltwater CrocodileOP
oh okay
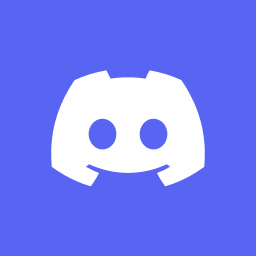
Saltwater CrocodileOP
But it is a kinda complex problem i think
I'll create a new Post
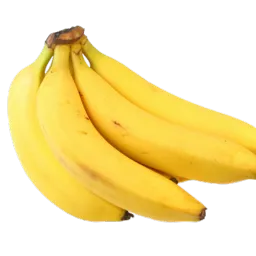
Yes!
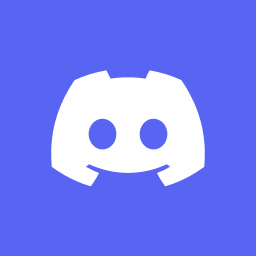
Saltwater CrocodileOP
MAybe you know something
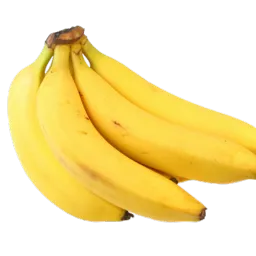
Ill try to check