Is it good practice to pass down getStaticProps variables as props to the children component?
Unanswered
Transvaal lion posted this in #help-forum
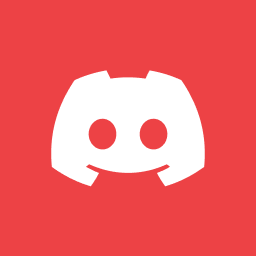
Transvaal lionOP
I have my
passing props to the child component, then the child takes this as props and passes it to another child component:
then it is finallly used in the
I'm getting the error:
Error: Failed prop type: The prop
Should I use
index.tsx
return (
<>
<DynamicLoad props={props} />
</>
)
passing props to the child component, then the child takes this as props and passes it to another child component:
return (
<>
<Socials props={props} />
</>
)
then it is finallly used in the
return(<>
<Link href={props.GitHub} >
<svg>...</svg>
</Link>
</>
)
I'm getting the error:
Error: Failed prop type: The prop
href
expects a string
or object
in <Link>
, but got undefined
instead.Should I use
getStaticProps
in the component where I use the props? I use the child component in the folder level src > pages > components > Socials.tsx
.4 Replies
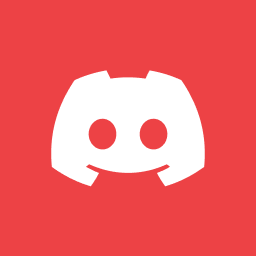
Asian black bear
You are likely getting the undefined error because props.GitHub does not exist yet so its important that you do checks to make sure that it exists before passing the value into the href.
Also is this value ever going to change? If you are using a variable that is never going to change then just pass the path in either directly or by creating a utils - constants folder and storing your constant variables in there and importing this into the Link
Also is this value ever going to change? If you are using a variable that is never going to change then just pass the path in either directly or by creating a utils - constants folder and storing your constant variables in there and importing this into the Link
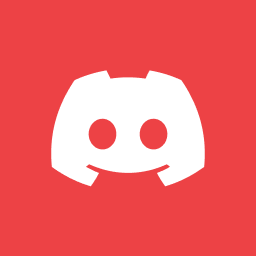
Transvaal lionOP
I wanted to understand how
getStaticProps
works and wanted to see if I could send variables through components. The values are going to be constant. I don't understand how the Github
variable is undefined since I do a simple console.log(Github)
. I'm guessing that console.log
is working on the server and not on the client or something? I'm not sure.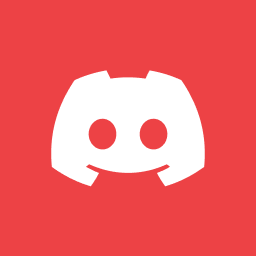
Asian black bear
The console.log will run on the server or the client depending on what you are telling it run on i.e using 'use client'. You can send variables through components as long as they are being sent down from the parent to the child. Also using getStaticProps is server rendered and used when making api calls which again means you have to wait for the data to be fetched before trying to return anything:
return(
#Unknown Channel
{props.GitHub &&
<Link href={props.GitHub}>
<svg>...</svg>
</Link>
}
</>
);
return(
#Unknown Channel
{props.GitHub &&
<Link href={props.GitHub}>
<svg>...</svg>
</Link>
}
</>
);

Marchy
getStaticProps only runs at build time and is only used for static site generation
https://nextjs.org/docs/pages/building-your-application/data-fetching/get-static-props
Data gets passed down through props of the page component, the code you've shown doesn't show this part
https://nextjs.org/docs/pages/building-your-application/data-fetching/get-static-props
Data gets passed down through props of the page component, the code you've shown doesn't show this part
export async function getStaticProps() {
const res = await fetch('https://api.github.com/repos/vercel/next.js')
const repo = await res.json()
return { props: { repo } }
}
export default function Page({ repo }) {
return repo.stargazers_count
}