Fetching data
Answered
Shaurya posted this in #help-forum
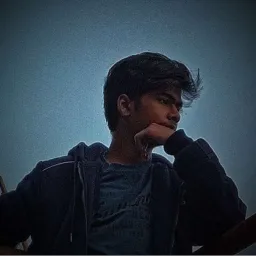
ShauryaOP
export function Stats() {
const [totalGuilds, setTotalGuilds] = useState("N/A")
useEffect(() => {
async function getGuilds() {
const guilds = await getTotalGuilds()
setTotalGuilds(guilds)
}
getGuilds()
}, [])
return (
<div className={styles.stats}>
{totalGuilds}
</div>
)
}
export async function getTotalGuilds() {
const token = process.env.NEXT_PUBLIC_BOT_TOKEN
if (!token) return "N/A"
const guildsEndpoint = `${dapi}/users/@me/guilds`
const response = await fetch(guildsEndpoint, {
headers: {
Authorization: `Bot ${token}`
}
})
const guilds = await response.json() as Guild[] | undefined
const totalGuilds = guilds ? guilds.length.toString() : "N/A"
return totalGuilds
}
This works okay but I get the errorGET https://discord.com/api/v10/users/@me/guilds 429 (Too Many Requests)
but I'm fetching only once? how is it repeating?Answered by joulev
server component, or [route handler](https://nextjs.org/docs/app/building-your-application/routing/route-handlers), or your separate backend, etc basically anywhere that is not in the browser
9 Replies

idk what's the answer to your question, but bot tokens are secrets so do not expose them to the browser by using
NEXT_PUBLIC
instead make authenticated server-side api routes instead, and use the bot token there
and fetch that api route from your frontend
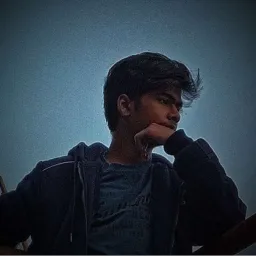
ShauryaOP
oh, yeah
do you mean making it a server component?
can you link me the docs please if that's not what you meant

server component, or [route handler](https://nextjs.org/docs/app/building-your-application/routing/route-handlers), or your separate backend, etc basically anywhere that is not in the browser
Answer
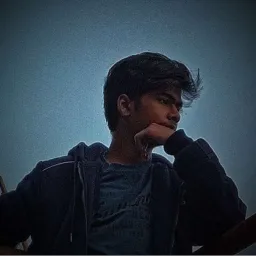
ShauryaOP
thanks,
this one is safe right? got rid of the
export default async function Stats() {
const data = await getTotalGuilds()
return (
<div className={styles.stats}>
{data}
</div>
)
}
export async function getTotalGuilds() {
const token = process.env.BOT_TOKEN
if (!token) return "N/A"
const response = await fetch(`${dapi}/users/@me/guilds`, {
headers: {
Authorization: `Bot ${token}`
}
})
const guilds = await response.json() as Guild[] | undefined
const totalGuilds = Array.isArray(guilds) ? guilds.length.toString() : "N/A"
return totalGuilds
}
it's working good rn, not getting the too many requests errorthis one is safe right? got rid of the
NEXT_PUBLIC

yes that is safe