500 Internal Server Error
Unanswered
European anchovy posted this in #help-forum
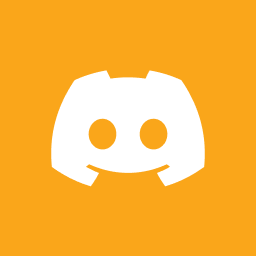
European anchovyOP
I'm currently using Next.js 13 with app directory, TypeScript, Prisma, formik, yup and next auth in my project. I create a form for create a new project and try to write an api for write form data in the database but when I try submit the form, get 500 internal server error in network page at browser devtools.
Form component as in the picture below. It's a bit long so I only share in a image sorry for that.
In below my api file code.
Form component as in the picture below. It's a bit long so I only share in a image sorry for that.
In below my api file code.
@/app/api/project/route.ts
import prisma from "@/lib/prisma";
import { NextResponse } from "next/server";
export async function POST(request: Request) {
// Handle POST request
const cookie = request.headers.get("cookie");
const res = await fetch("http://localhost:3000/api/auth/session", {
headers: {
cookie: cookie!,
},
});
if (!cookie) {
return new Response("Unauthorized", { status: 401 });
}
const session = await res.json();
console.log("Session data:", session);
if (!session || !session.user || !session.user.email) {
return new Response("Unauthorized", { status: 401 });
}
try {
const data = await request.json();
const project = await prisma.project.create({
data: {
name: data.name,
status: data.status,
startDate: data.startDate,
endDate: data.endDate,
description: data.description,
user: {
connect: { email: session.user.email },
},
},
});
let json_data = {
status: 201,
data: {
project,
},
};
return new NextResponse(JSON.stringify(json_data), {
status: 201,
headers: {
"Content-Type": "application/json",
},
});
} catch (error) {
console.error("Error creating project:", error);
return new Response("Error creating project", { status: 500 });
}
}

11 Replies
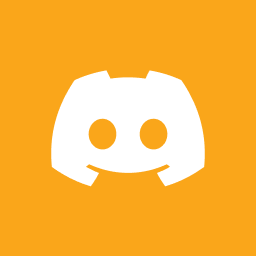
European anchovyOP
My form file:



risky
i see that you are fetching your own api in route handler: https://nextjs-faq.com/fetch-api-in-rsc ( fyi you shouldnt)
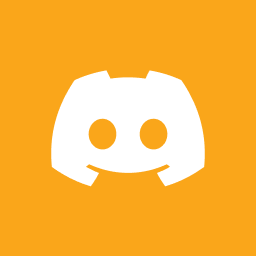
European anchovyOP
@risky
I'm new to Next13, what I tried to do here was to create a folder called API just like we did in the pages folder and write a simple API in it. But for the application directory this seems more complicated. Actually, I can explain what I am trying to do as in the example below. However, I had a hard time doing this with the app directory.
I'm new to Next13, what I tried to do here was to create a folder called API just like we did in the pages folder and write a simple API in it. But for the application directory this seems more complicated. Actually, I can explain what I am trying to do as in the example below. However, I had a hard time doing this with the app directory.
import { NextApiRequest, NextApiResponse } from "next";
import { getSession } from "next-auth/client";
import { PrismaClient } from "@prisma/client";
const prisma = new PrismaClient();
export default async function handler(
req: NextApiRequest,
res: NextApiResponse
) {
if (req.method !== "POST") {
return res.status(405).json({ error: "Method not allowed" });
}
const session = await getSession({ req });
if (!session) {
return res.status(401).json({ error: "Unauthorized" });
}
try {
const { name, status, startDate, endDate, description } = req.body;
if (!name || !status || !startDate || !endDate || !description) {
return res.status(400).json({ error: "Missing parameters" });
}
const user = await prisma.user.findUnique({
where: {
email: session.user.email,
},
});
if (!user) {
return res.status(401).json({ error: "Unauthorized" });
}
const project = await prisma.project.create({
data: {
name,
status,
startDate,
endDate,
description,
userId: user.id, // Assuming a direct foreign key relationship in your Prisma model
},
});
return res.status(201).json({
success: true,
project,
});
} catch (error) {
console.error(error);
return res.status(500).json({ error: "Internal server error" });
} finally {
await prisma.$disconnect();
}
}
Do you have any resource suggestions for something like this? I couldn't find a sufficient source. Also, is it possible to create an API like this with the app directory?
i don't know if there is a good convert resource tho (i only really learnt app dir)
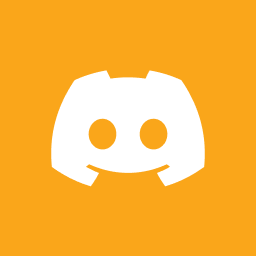
European anchovyOP
These look nice, thanks for helping dude 💙

risky
let me know how this goes 🙂
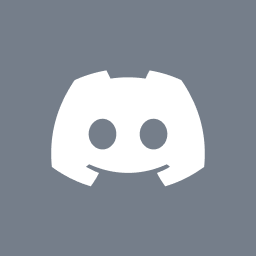
Mackenzie River Husky
@risky @European anchovy
I am have a similar issue any chance you could browse my post?
I am have a similar issue any chance you could browse my post?