SWR weirdness
Answered
Spectacled bear posted this in #help-forum
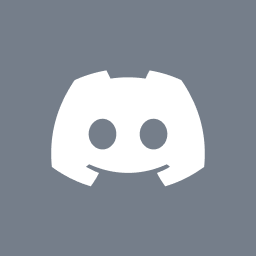
Spectacled bearOP
//image-card.tsx
export function ImageCard(props: { imageID: string }) {
const { data: statusData, mutate } = useSWR<{
payload: {
queuePosition: number
url: string
status: "pending" | "complete"
}
}>(
"https://foobar",
(url: string) =>
fetch(url, {
method: "POST",
body: JSON.stringify({
sessionID: "foobar",
imageID: props.imageID,
}),
}).then((r) => r.json()),
{
refreshInterval: (latestData) =>
latestData?.payload.status !== "complete" ? 1000 : 0,
revalidateIfStale: false,
revalidateOnFocus: false,
revalidateOnReconnect: false,
},
)
useEffect(() => {
void mutate()
// eslint-disable-next-line react-hooks/exhaustive-deps
}, [props.imageID])
if (!statusData) return
if (statusData.payload.status === "pending")
return (
<Skeleton className="grid h-[500px] w-[500px] place-content-center">
{statusData.payload.queuePosition}
</Skeleton>
)
return (
<img
src={statusData.payload.url}
alt="generated-img"
className="max-w-lg"
/>
)
}
//app.tsx
<div>
{images.map((el) => (
<ImageCard key={el} imageID={el} />
))}
</div>
This renders the same image for all items in the array and I can't understand why.
Answered by risky
Pass your image Id into the swr input... This is so that swr can see that they are different items...
3 Replies
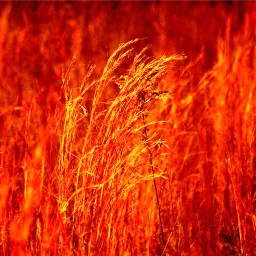
Pass your image Id into the swr input... This is so that swr can see that they are different items...
Answer
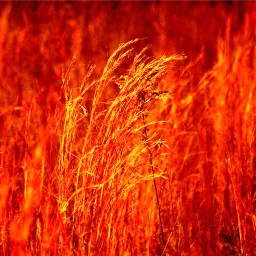
@Spectacled bear see https://swr.vercel.app/docs/arguments#multiple-arguments for more info
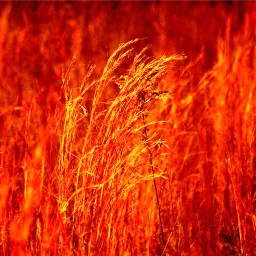
@risky <@985619203132362812> see https://swr.vercel.app/docs/arguments#multiple-arguments for more info
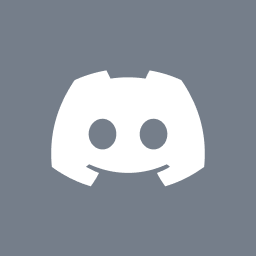
Spectacled bearOP
Thanks. Didn't expected to get an answer for this. Saved meðŸ™