date formatting
Unanswered
Cape lion posted this in #help-forum
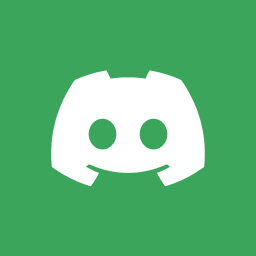
Cape lionOP
how do I format my date so that I get 2023-10-01 instead of 2023-10-1?
3 Replies
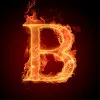
You can do it like that:
let date = moment().format();
console.log(date); // 2022-08-23T16:50:22-07:00
And then split a „T“ and get the first argument from the array
let date = moment().format();
console.log(date); // 2022-08-23T16:50:22-07:00
And then split a „T“ and get the first argument from the array
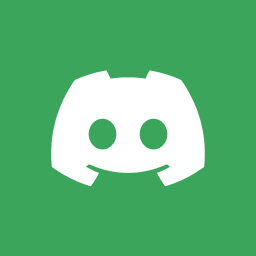
Largehead hairtail
import format from 'date-fns/format';
format(new Date(publish_time), 'yyyy-MMM-dd')

Assuming that you want to get local time, I'd suggest to use the native JS
Notice that the locale (the first argument from
toLocaleDateString
method:const date = new Date('2023-10-01')
const deDate = date.toLocaleDateString('de', {
timeZone: 'Europe/Zurich',
month: '2-digit',
day: '2-digit',
year: 'numeric',
})
// => "01.10.2023"
const enDate = date.toLocaleDateString('en', {
timeZone: 'Europe/Zurich',
month: '2-digit',
day: '2-digit',
year: 'numeric',
})
// => "10/01/2023"
Notice that the locale (the first argument from
toLocaleDateString
) is important to find a proper format. So I'd suggest you to use 'sv' if you want a UTC-like output format:const date = new Date('2023-10-01')
const svDate = date.toLocaleDateString('sv', {
timeZone: 'Europe/Zurich',
month: '2-digit',
day: '2-digit',
year: 'numeric',
})
// => "2023-10-01"