Passing props from child components to parents
Unanswered
Chum salmon posted this in #help-forum
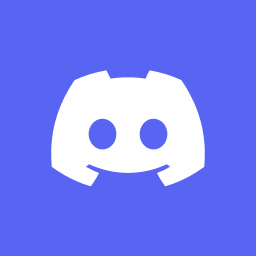
Chum salmonOP
I'm working on my first social media type web-app and I'm running into a problem when it comes to fetching posts after a post is successfully created (due to the way my file structure is).
I want the user to be able to create a post anywhere on the site, so I have my
Is there a way to do this or is there a better way to restructure this?
CreatePost.tsx
layout.tsx
page.tsx
I want the user to be able to create a post anywhere on the site, so I have my
<CreatePost />
component inside my root layout.tsx
. The function that fetches posts is inside the root page.tsx
("use client"). So I would like to create some kind of trigger that when the post is successfully created, it will pass a prop into the page.tsx which will run the fetchPost function. Is there a way to do this or is there a better way to restructure this?
CreatePost.tsx
export default function CreatePost() {
const supabase = useSupabaseClient();
const [content, setContent] = useState('');
const handleSubmit = () => {
supabase.from('tableName')
.insert({
author: session?.user.id,
content
}).then(result => {
if (!result.error) {
setContent('')
handleClose()
// where I want the trigger to happen
}
})
};
}
layout.tsx
export default function RootLayout({children}) {
return (
<html>
<body>
<NavBar />
{children}
<CreatePost /> {/* trigger from here */}
</body>
</html>
)
}
page.tsx
export default function Home() {
const session = useSession();
const supabase = useSupabaseClient()
const [posts, setPosts] = useState([])
useEffect(() => {
if (!session?.user.id) {
redirect('/login')
}
fetchPosts()
}, [])
const fetchPosts = () => {
supabase.from('tableName')
.select()
.order('created_at', {ascending: false})
.then(result => {
setPosts(result.data)
})
}
return (
<main>
<div>
{posts.length > 0 && posts.map((post: any) => (
<Post key={post.id} {...post} />
))}
</div>
</main>
)
}