Disable SSG but keep SSR or ISR?
Unanswered
Saltwater Crocodile posted this in #help-forum
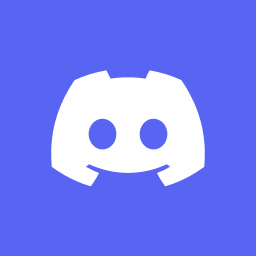
Saltwater CrocodileOP
I am feeling very dense, but I'm trying to figure out how to make a page that is rendered on the server and cached, but only after deployment. I 100% rely on data from a headless system that is only available on the server after deployment, so I don't want SSG to get a copy of my local data for example and deploy it.
I read, or tried to read, https://nextjs.org/docs/app/building-your-application/upgrading/app-router-migration#static-site-generation-getstaticprops but I'm still confused / uncertain.
There seems to be some magic happening that is triggered when you're using various functions and things, but it's not clear to me.
Here is a very simple example, a simplified version of my
I read, or tried to read, https://nextjs.org/docs/app/building-your-application/upgrading/app-router-migration#static-site-generation-getstaticprops but I'm still confused / uncertain.
There seems to be some magic happening that is triggered when you're using various functions and things, but it's not clear to me.
Here is a very simple example, a simplified version of my
/src/app/page.tsx
"homepage":const getBlockContent = async (code: string): Promise<Content | null> => {
const client = createClient();
// makes a request to backend resources, doesn't work client side
const result = await getContentByCode(client, ContentType.BLOCK, code);
return result;
};
export default async function Home(): Promise<JSX.Element> {
return (
<div>
<div className="blog-content">
<Block content={await getBlockContent("home-page-top")} />
</div>
</div>
);
}
24 Replies

Alfonsus Ardani
ISR is an extension of SSG
you need to choose between statically computing the routes: SSG or ISR
or dynamically computing the routes at request time: SSR
or dynamically computing the routes at request time: SSR
how to make a page that is rendered on the server and cached, but only after deploymentMake the routes SSR by using
export const dynamic = 'force-dynamic'
and use Data Cache to cache fetches/getters/fns to persists the result in between requests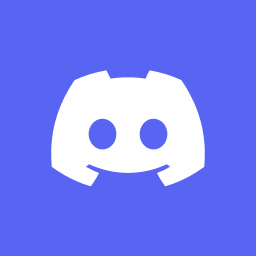
Saltwater CrocodileOP
Thanks a ton @Alfonsus Ardani , really appreciate it.
I'm sorry to ask a lazy follow up but my head is all over the place and I'm struggling to parse some of this.
When you said
It sounds like you're suggesting my solution is to force SSR, and then granularly at the application / code level cache things - ideall I do not want to cache at that granularity, rather I want to use (as I understand it) ISR, except without the initial deploy / build time SSG.
Does that make sense? In other words, I want to cache the full document / html for the SSR page, but at the page / level not at the fetch/getter/fncs level.
I'm sorry to ask a lazy follow up but my head is all over the place and I'm struggling to parse some of this.
When you said
Make the routes SSR by using export const dynamic = 'force-dynamic' and use Data Cache to cache fetches/getters/fns to persists the result in between requests
It sounds like you're suggesting my solution is to force SSR, and then granularly at the application / code level cache things - ideall I do not want to cache at that granularity, rather I want to use (as I understand it) ISR, except without the initial deploy / build time SSG.
Does that make sense? In other words, I want to cache the full document / html for the SSR page, but at the page / level not at the fetch/getter/fncs level.

Alfonsus Ardani
it make sense
but i havent tried it
what are the routes that you are planning to use?
afaik you can just use
generateStaticParams
and return an empty array and that should work as ISR since none of the routes are generated at deployment but it will be built at request time, once, per invalidationthat only works for dynamic variadic routes like
/[id]
though, idk what to do for static routeswhats keeping you from using SSR? if i may know
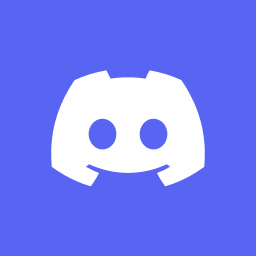
Saltwater CrocodileOP
My use case is very simple, it's a small site with a blog. That said, the content all comes frmo a headless CMS, and the data is environment specific, so I really don't want to generate the pages during deployment.
I worry that my answer here could be unclear (sorry!) - I actually WANT to use SSR, I just want the result cached. And I don't want to cache at the application / code level.
whats keeping you from using SSR? if i may know
I worry that my answer here could be unclear (sorry!) - I actually WANT to use SSR, I just want the result cached. And I don't want to cache at the application / code level.

Alfonsus Ardani
Ah yeah i meant, whats keeping you from using dynamically coputed routes that would render server components at request time, per user? Its usually inexpensive to render those stuff if you have the data cached already
In Next.js, SSR is commonly known as rendering codes at request time, per user. I know the fact that SSG is also "server side rendering" but they are more specific towards outputting a static html, hence static site generation. So sorry for the confusion
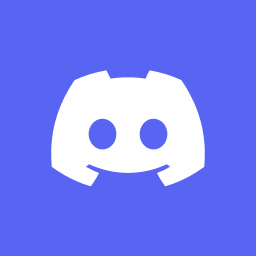
Saltwater CrocodileOP
Yeah nothing really stopping me I guess - I just like the idea of ISR. I'm using
open-next
so everything is served out of S3 if it's cached correctly I think.
Alfonsus Ardani
The data cache when using dynamically computed routes can also behave like the cache used in ISR: background revalidation, on-demand revalidation, fetch if cache is absent.
but yeah, back to your question, i dont think theres a way to disable initial Render at Deployment Time with ISR unless you are using variadic/dynamic routes like
/[id]
or /[...catchall]
a.k.a in places where generateStaticParams
would be relevantusing generateStaticParams would allow you to build HTMLs at request time and have it cached later long after deployment
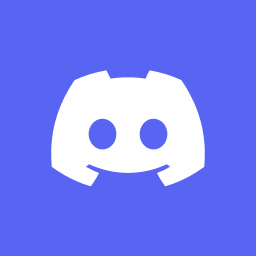
Saltwater CrocodileOP
ok. Well, I really appreciate it. To be honest I think I need to spend a bit of time experiementing perhaps to build up a better understanding of some of this.
generateStaticParams
for example I've seen but it doesn't mean a ton to me at this poitn because I haven't used it and I am new to Next.js and am still a bit confused when I see something like that if it's relevant for the "Page router" vs "App router", etc... so perhaps I'll start with what you originally mentioned (const dynamic = 'force-dynamic'
) and experiement with that and go from there
Alfonsus Ardani
const dynamic = 'force-dynamic'
will make your route be computed at runtime for every request and userif thats what you want then ok...
oh ive been answering the question based on App Router, sorry 🤣
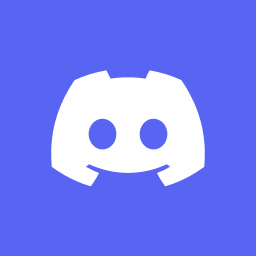
Saltwater CrocodileOP
perfect, that's what i'm using 🙂
if thats what you want then ok.
It's not, but it's the "nuclear option" i can start with, if that makes sense.
Then I'll do as you say and figure out / test how to use
generateStaticParams
instead, and see how that behavior differs
Alfonsus Ardani
theres like 2 ways a route can be computed:
- dynamically computed at every request time ->
- static, once, either from a known route or opt-in to ISR ->
- dynamically computed at every request time ->
const dynamic = 'force-dynamic'
-> usually called SSR- static, once, either from a known route or opt-in to ISR ->
generateStaticParams
-> usually called SSG