How to secure specific pages using NextAuth and Middleware after authentication
Unanswered
Hamodi posted this in #help-forum
Original message was deleted.
17 Replies
Try using the middleware this way:
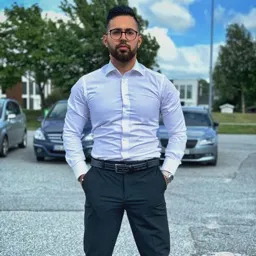
Hamodi
Something like that:
import { withAuth } from 'next-auth/middleware';
export default withAuth(
{
callbacks: {
authorized: ({ req, token }) => {
if (req.nextUrl.pathname === "/dashboard") {
return token?.role=== "ADMIN"
}
if (req.nextUrl.pathname === "/booking") {
return token?.role=== "USER"
}
return !!token;
},
},
}
);
export const config = {
matcher: ["/dashboard/:path*", "/booking/:path*", "/settings/:path*"]
};
But what about the / and /signup, I don't want to show them if user is authenticated.
Clown
If i remember correctly, the Authorized callback is supposed to return a boolean. If its true you continue, if its not true the user ends up going to the sign up page.
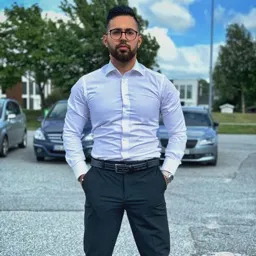
Hamodi
After the changes: if the user has a role of "USER" and is logged in and the user tries to access /dashboard then he is sent to callbackUrl "the sign in page"

Clown
Are you returning true?
In the condition?
Just console log to check if you are even getting the userRole passed correctly
Cause its gonna return false otherwise
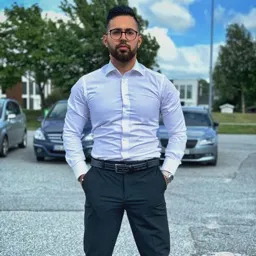
Hamodi
import { withAuth } from 'next-auth/middleware';
export default withAuth(
{
callbacks: {
authorized: ({ req, token }) => {
if (req.nextUrl.pathname === "/dashboard" && token?.role !== "ADMIN") {
console.log("req.nextUrl.pathname", req.nextUrl.pathname);
console.log("token?.role", token?.role);
//return to /booking if the role is not admin
//return true will give access to /dashboard
//return false will not give access to /dashboard nd send to callbackUrl "signin"
}
if (req.nextUrl.pathname === "/booking" && token?.role !== "USER") {
console.log("req.nextUrl.pathname", req.nextUrl.pathname);
console.log("token?.role", token?.role);
//return to /dashboard if the role is not user
//return true will give access to /booking
//return false will not give access to /booking and send to callbackUrl "signin"
}
return !!token;
},
},
}
);
export const config = {
matcher: ["/dashboard/:path*", "/booking/:path*", "/settings/:path*"]
};
I am getting the pathname and role in console: req.nextUrl.pathname /dashboardtoken?.role USER

Clown
Wasnt it supposed to be token.userRole???
Thats why i am saying do console.log to check if you are receiving the token and its properties correctly
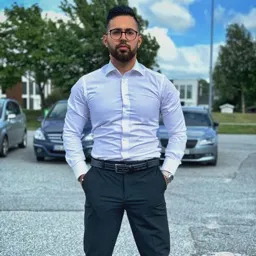
Hamodi
No. it is role I made a typo

According to the documentation: "The middleware function will only be invoked if the authorized callback returns true."
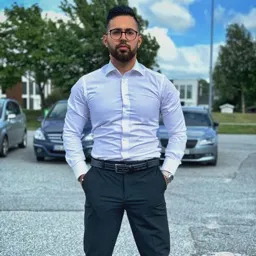
Hamodi
#next-auth Any one who can help?

Clown
Try #next-auth